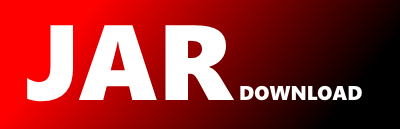
org.ow2.petals.cli.api.shell.Shell Maven / Gradle / Ivy
/**
* Copyright (c) 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.api.shell;
import java.io.PrintStream;
import java.util.Map;
import org.ow2.petals.cli.api.command.Command;
import org.ow2.petals.cli.api.command.ConnectionCommand;
import org.ow2.petals.cli.api.command.DisconnectionCommand;
import org.ow2.petals.cli.api.connection.ConnectionParameters;
import org.ow2.petals.cli.api.exception.NoInteractiveShellException;
import org.ow2.petals.cli.api.pref.Preferences;
import org.ow2.petals.cli.api.shell.exception.ConnectionCommandAlreadyRegisteredException;
import org.ow2.petals.cli.api.shell.exception.DisconnectionCommandAlreadyRegisteredException;
import org.ow2.petals.cli.api.shell.exception.DuplicatedCommandException;
import org.ow2.petals.cli.api.shell.exception.ShellException;
/**
* Basic interface of a shell
*
* @author Christophe DENEUX - Linagora
*/
public interface Shell {
/**
* Register a command
*
* @param command
* The command to register. Not null
.
* @throws IllegalArgumentException
* The command is null
.
* @throws DuplicatedCommandException
* The command is already defined
*/
public void registersCommand(final Command command) throws DuplicatedCommandException,
IllegalArgumentException;
/**
*
* Register the connection command.
*
*
* Note: Only one connection command can be registered.
*
*
* @param connectionCommand
* The connection command to register. Not null
.
* @throws IllegalArgumentException
* The command is null
.
* @throws ConnectionCommandAlreadyRegisteredException
* A connection command is already registered
* @throws DuplicatedCommandException
* The connection command is already defined as a command
*/
public void registersConnectionCommand(final ConnectionCommand connectionCommand)
throws ConnectionCommandAlreadyRegisteredException, DuplicatedCommandException, IllegalArgumentException;
/**
*
* Register the disconnection command.
*
*
* Note: Only one disconnection command can be registered.
*
*
* @param disconnectionCommand
* The disconnection command to register. Not null
.
* @throws IllegalArgumentException
* The command is null
.
* @throws DisconnectionCommandAlreadyRegisteredException
* A disconnection command is already registered
* @throws DuplicatedCommandException
* The disconnection command is already defined as a command
*/
public void registersDisconnectionCommand(final DisconnectionCommand disconnectionCommand)
throws DisconnectionCommandAlreadyRegisteredException, DuplicatedCommandException, IllegalArgumentException;
/**
* Get the available commands
*
* @return a set of commands mapped with their name
*/
public Map getCommands();
/**
* Get the connection command
*
* @return The connection command, or null
is no connection command was registered.
*/
public ConnectionCommand getConnectionCommand();
/**
* Get the disconnection command
*
* @return The disconnection command, or null
is no disconnection command was registered.
*/
public DisconnectionCommand getDisconnectionCommand();
/**
* Set an exit code and exit the current shell
*
* @param exitStatus
* the exit code
*/
public void exit(int status);
/**
* Set the exit code of the current shell
*
* @param exitStatus
* the exit code
*/
public void setExitStatus(int exitStatus);
/**
* Get the output stream where anything can be written, mainly by commands.
*
* @return The output stream.
*/
public PrintStream getPrintStream();
/**
* Get the error stream where anything can be written, mainly by commands.
*
* @return The error stream.
*/
public PrintStream getErrorStream();
/**
* Interpolates variables and special characters in a string
*
* @param str
* the string to be interpolated
* @return a new string with interpolated variables
*/
public String interpolate(final String str);
/**
* Asks a question
*
* @param message
* The asked question
* @param isReplyPassword
* true
if the expected reply is a password,
* false
otherwise.
* @return The reply to the question
* @throws NoInteractiveShellException
* If the shell is not interactive
* @throws ShellException
* An error occurs asking the question
*/
public String askQuestion(final String question, final boolean isReplyPassword)
throws NoInteractiveShellException, ShellException;
/**
* Confirms an action to do
*
* @param message
* The message to display if needed
* @return true
if the action is confirmed, false
* otherwise
* @throws ShellException
* An error occurs confirming the message
*/
public boolean confirms(final String message) throws ShellException;
/**
* @return true
if the shell is interactive, false
* otherwise
*/
public boolean isInteractive();
/**
*
* Set the shell prompt to a custom value.
*
*
* If the shell has no prompt, this method will do nothing.
*
*
* @param prompt
*/
public void setPrompt(final String prompt);
/**
*
* Set the shell prompt to its default value.
*
*
* If the shell has no prompt, this method will do nothing.
*
*/
public void setDefaultPrompt();
/**
* @return true if the debug mode is enabled, false otherwise
*/
public boolean isDebugModeEnable();
/**
* Defines the connection parameters of the Petals node on which the shell
* is connected.
*
* @param connectionParameters
* The connection parameters
*/
public void setConnectionParameters(final ConnectionParameters connectionParameters);
/**
* @return The connection parameters used by the shell to establish the
* connection to the Petals node. Can be null
if the
* shell is not connected.
*/
public ConnectionParameters getConnectionParameters();
/**
* @return true
if an asynchronous command is in progress,
* false
otherwise.
*/
public boolean isAsynchronousCommandInProgress();
/**
* Register a new asynchronous command in progress
*/
public void addAsynchronousCommand();
/**
* Deregister a new asynchronous command in progress
*/
public void removeAsynchronousCommand();
/**
* @return The shell extensions
*/
public ShellExtension[] getExtensions();
/**
* @return The preferences contains in the preference file
*/
public Preferences getPreferences();
/**
* Inform the shell that a connection is established
*/
public void onConnectionEstablished();
/**
* Inform the shell that a connection is disconnected.
*/
public void onDisconnection();
/**
* @return The connection state: true
is a connection is established, false
otherwise
*/
public boolean isConnectionEstablished();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy