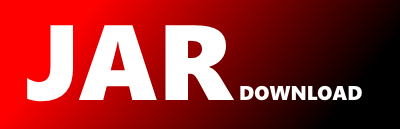
org.ow2.petals.cli.base.junit.AbstractConsoleInOutTest Maven / Gradle / Ivy
/**
* Copyright (c) 2015-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.base.junit;
import static org.hamcrest.Matchers.endsWith;
import static org.junit.Assert.assertTrue;
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStream;
import java.util.Properties;
import org.hamcrest.Matcher;
import org.junit.After;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Rule;
import org.junit.contrib.java.lang.system.EnvironmentVariables;
import org.junit.contrib.java.lang.system.SystemErrRule;
import org.junit.contrib.java.lang.system.SystemOutRule;
import org.junit.rules.TemporaryFolder;
import org.ow2.petals.cli.base.junit.rules.ConsoleInput;
import com.jayway.awaitility.Awaitility;
import com.jayway.awaitility.Duration;
import jline.TerminalFactory;
import jline.TerminalFactory.Type;
/**
*
* @author vnoel
*
*/
public class AbstractConsoleInOutTest {
static {
// disable advanced feature for completion and stuffs in the terminal
// This avoids to break the output in the terminal when tests are executed from the command line with maven.
TerminalFactory.configure(Type.NONE);
}
@Rule
public final EnvironmentVariables envVars = new EnvironmentVariables();
@Rule
public final SystemOutRule systemOut = new SystemOutRule().muteForSuccessfulTests().enableLog();
@Rule
public final SystemErrRule systemErr = new SystemErrRule().muteForSuccessfulTests().enableLog();
@Rule
public final ConsoleInput systemIn = new ConsoleInput();
@Rule
public final TemporaryFolder tempFolder = new TemporaryFolder();
public void initAwaitTimeout() {
Awaitility.setDefaultTimeout(Duration.ONE_SECOND);
}
protected void checkOut(final Matcher matcher) {
Awaitility.await().untilCall(Awaitility.to(systemOut).getLog(), matcher);
assertTrue("An error occurs", systemErr.getLog().isEmpty());
systemOut.clearLog();
// let's reinit for next call
initAwaitTimeout();
}
protected void checkErrEndsWith(final String end) {
checkErr(endsWith(String.format("%s%n", end)));
}
protected void checkErr(final Matcher matcher) {
Awaitility.await().untilCall(Awaitility.to(systemErr).getLog(), matcher);
systemErr.clearLog();
// let's reinit for next call
initAwaitTimeout();
}
protected void setUpPrefFile(final String varName, final Properties preferenceProperties) throws Exception {
final File prefFile = tempFolder.newFile("pref-file.properties");
envVars.set(varName, prefFile.getAbsolutePath());
try (final OutputStream fos = new FileOutputStream(prefFile)) {
preferenceProperties.store(fos, "");
}
}
protected void setUpUnreadablePrefFile(final String varName) throws Exception {
final File prefFile = tempFolder.newFile("unreadable.properties");
prefFile.setReadable(false);
envVars.set(varName, prefFile.getAbsolutePath());
}
protected void setUpInvalidPrefFile(final String varName) throws Exception {
final File prefFile = tempFolder.newFile("invalid.properties");
prefFile.delete();
envVars.set(varName, prefFile.getAbsolutePath());
}
protected void setUpDirectoryAsPrefFile(final String varName) throws Exception {
final File prefFile = tempFolder.newFolder("dirAsPrefFile.properties");
envVars.set(varName, prefFile.getAbsolutePath());
}
protected void setUpEmptyPrefFile(final String varName) throws Exception {
envVars.set(varName, "");
}
protected volatile AssertionError assertion;
private Thread petalsMockCliThread;
/**
* If one use this method, one must call {@link #waitThreadOrFail()} at the end!
*
*/
protected void runCli(final Runnable run) {
this.petalsMockCliThread = new Thread(new Runnable() {
@Override
public void run() {
try {
run.run();
} catch (final AssertionError e) {
assertion = e;
}
}
}, "Petals-Mock-CLI-Thread");
this.petalsMockCliThread.start();
}
protected void waitThreadOrFail() {
waitThreadOrFail(2000);
}
protected void waitThreadOrFail(final long timeout) {
try {
this.petalsMockCliThread.join(timeout);
} catch (InterruptedException e) {
Assert.fail("join was interrupted");
}
Assert.assertFalse("Thread " + this.petalsMockCliThread.getName() + " should have stopped, but hasn't",
this.petalsMockCliThread.isAlive());
this.petalsMockCliThread = null;
if (this.assertion != null) {
try {
throw this.assertion;
} finally {
this.assertion = null;
}
}
}
@Before
public void cleanAssertion() {
this.assertion = null;
}
@After
public void noCliThreadLeft() {
Assert.assertNull("waitThreadOrFail() should have been called!", this.petalsMockCliThread);
Assert.assertNull(this.assertion);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy