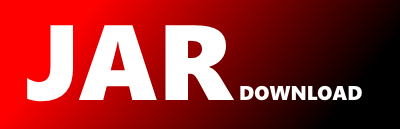
org.ow2.petals.cli.base.junit.Assert Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.base.junit;
import java.util.Iterator;
import java.util.List;
import org.ow2.petals.cli.api.command.exception.CommandInvalidArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingOptionsException;
public class Assert extends org.junit.Assert {
/**
* Assertions about the content of {@link CommandMissingOptionsException}.
*
* @param commandMissingOptionsException
* The exception to check
* @param expectedOptions
* The expected missing options.
*/
public static void assertCommandMissingOptionsException(
final CommandMissingOptionsException commandMissingOptionsException, final String... expectedOptions) {
assertTrue("Error label is missing.",
commandMissingOptionsException.getMessage().startsWith(CommandMissingOptionsException.MISSING_OPTIONS));
assertEquals(expectedOptions.length, commandMissingOptionsException.getMissingOptions().size());
for (final String expectedOption : expectedOptions) {
assertTrue("The option '-" + expectedOption + "' shoul be missing.",
commandMissingOptionsException.getMissingOptions().contains(expectedOption));
assertTrue("The option '-" + expectedOption + "' shoul be missing.",
commandMissingOptionsException.getMissingOptions().contains(expectedOption));
}
assertTrue("Missing options are missing in error message.", commandMissingOptionsException.getMessage()
.endsWith(convertOptions2String(commandMissingOptionsException.getMissingOptions())));
}
private static String convertOptions2String(final List missingOptions) {
final StringBuffer message = new StringBuffer();
final Iterator itMissingOptions = missingOptions.iterator();
while (itMissingOptions.hasNext()) {
message.append(itMissingOptions.next());
if (itMissingOptions.hasNext()) {
message.append(", ");
}
}
return message.toString();
}
/**
* Assertions about the content of {@link CommandInvalidArgumentException}.
*
* @param commandInvalidArgumentException
* The exception to check
* @param expectedOption
* The expected option having an invalid value.
* @param expectedInvalidValue
* The expected invalid value.
*/
public static void assertCommandInvalidArgumentException(
final CommandInvalidArgumentException commandInvalidArgumentException, final String expectedOption,
final String expectedInvalidValue) {
assertEquals("The invalid argument is not relative to the option '-" + expectedOption + "'", expectedOption,
commandInvalidArgumentException.getOption().getOpt());
assertEquals("Unexpected invalid value", expectedInvalidValue, commandInvalidArgumentException.getValue());
}
/**
* Assertions about the content of {@link CommandMissingArgumentException}.
*
* @param commandMissingArgumentException
* The exception to check
* @param expectedOption
* The expected option for which the value is missing.
*/
public static void assertCommandMissingArgumentException(
final CommandMissingArgumentException commandMissingArgumentException, final String expectedOption) {
assertTrue("Error label is missing.", commandMissingArgumentException.getMessage()
.startsWith(CommandMissingArgumentException.MISSING_ARGUMENT));
assertEquals("The option '-" + expectedOption + "' should have a missing argument.", expectedOption,
commandMissingArgumentException.getOption().getOpt());
assertTrue("Missing argument is missing in error message.",
commandMissingArgumentException.getMessage().endsWith(expectedOption));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy