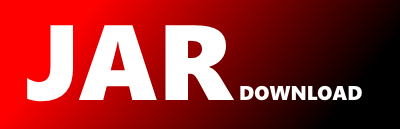
org.ow2.petals.cli.command.SystemCall Maven / Gradle / Ivy
/**
* Copyright (c) 2012 EBM WebSourcing, 2012-2023 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.command;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.PrintStream;
import org.apache.commons.cli.CommandLine;
import org.ow2.petals.cli.api.command.AbstractCommand;
import org.ow2.petals.cli.api.command.exception.CommandBadArgumentNumberException;
import org.ow2.petals.cli.api.command.exception.CommandException;
/**
* @author Alexandre Lagane - Linagora
*/
public class SystemCall extends AbstractCommand {
public SystemCall() {
super("system");
this.setUsage("usage: system ...");
this.setDescription("Execute system command");
}
/**
* {@inheritDoc}
*
*
* Dedicated processing to the command 'system': No connection is required
* to execute it.
*
*/
@Override
public boolean isConnectionRequired(CommandLine cli) {
return false;
}
@Override
public void doExecute(CommandLine cli, final String... args) throws CommandException {
if (args.length > 0) {
final StringBuilder toExecute = new StringBuilder();
final String osName = System.getProperty("os.name");
if (osName != null && osName.toLowerCase().contains("win")) {
toExecute.append("cmd /c ");
}
for (final String arg : args) {
toExecute.append(arg).append(' ');
}
try {
final Process process = Runtime.getRuntime().exec(toExecute.toString());
final BufferedInputStream stdout = new BufferedInputStream(process.getInputStream());
try {
final PrintStream ps = this.getShell().getPrintStream();
int c;
while ((c = stdout.read()) != -1) {
ps.write(c);
}
final int exitCode = process.waitFor();
this.getShell().setExitStatus(exitCode);
} finally {
stdout.close();
}
} catch (final IOException e) {
throw new CommandException(this, e);
} catch (final InterruptedException e) {
throw new CommandException(this, e);
}
} else {
throw new CommandBadArgumentNumberException(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy