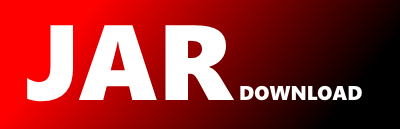
org.ow2.petals.cli.shell.PetalsInteractiveCli Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2023 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell;
import java.io.IOException;
import jline.TerminalFactory;
import jline.console.ConsoleReader;
import org.ow2.petals.cli.api.command.exception.CommandException;
import org.ow2.petals.cli.api.command.exception.CommandInvalidException;
import org.ow2.petals.cli.api.exception.NoInteractiveShellException;
import org.ow2.petals.cli.api.pref.Preferences;
import org.ow2.petals.cli.api.shell.ShellExtension;
import org.ow2.petals.cli.api.shell.exception.ShellException;
import org.ow2.petals.cli.shell.completer.CommandCompleter;
import org.ow2.petals.cli.shell.exception.UnknownCommandException;
public class PetalsInteractiveCli extends AbstractShell {
/**
* The response 'yes' to enter on confirmation
*/
private static final String YES = "y";
/**
* The response 'no' to enter on confirmation
*/
private static final String NO = "n";
/**
* Character masking password
*/
private static final char PASSWORD_MASK = '*';
ConsoleReader cr = null;
/**
*
* @param isDebugEnabled
* @param isYesFlagEnable
* Flag to enable the automatic confirmation
* @param preferences
* Preferences
* @param shellExtensions
* A list of shell extension, or null
if no shell extension is available
* @param basePrompt
* The base prompt
* @throws IOException
*/
public PetalsInteractiveCli(final boolean isDebugEnabled, final boolean isYesFlagEnable,
final Preferences preferences, final ShellExtension[] shellExtensions, final String basePrompt)
throws IOException {
super(System.out, System.err, isDebugEnabled, isYesFlagEnable, preferences, shellExtensions, basePrompt);
this.cr = new ConsoleReader(System.in, System.out, TerminalFactory.create());
this.cr.setHistoryEnabled(true);
// Because of a problem in JLine about escape characters ('\\') of regexp, event designators
// (http://www.gnu.org/software/bash/manual/html_node/Event-Designators.html) support is disabled.
this.cr.setExpandEvents(false);
this.setDefaultPrompt();
}
@Override
public void run() {
try {
// init completion
final CommandCompleter commandCompleter = new CommandCompleter(this.getCommands());
this.cr.addCompleter(commandCompleter);
String line;
while (this.isCommandRead && (line = this.cr.readLine()) != null) {
try {
this.evaluate(line);
} catch (final CommandInvalidException ce) {
this.printCommandSyntaxError(ce);
} catch (final CommandException ce) {
this.printCommandExecutionError(ce);
} catch (final UnknownCommandException uce) {
// No stack trace even in debug mode
this.errStream.println("ERROR: " + uce.getMessage());
} finally {
this.cr.flush();
}
}
} catch (final IOException ioe) {
this.printError(ioe);
this.setExitStatus(1);
}
}
@Override
public void setPrompt(final String prompt) {
this.cr.setPrompt(prompt);
}
@Override
public String askQuestion(final String question, final boolean isReplyPassword)
throws NoInteractiveShellException, ShellException {
if (this.isYesFlagEnabled) {
throw new NoInteractiveShellException();
} else {
try {
this.cr.setHistoryEnabled(false);
final String prompt = this.cr.getPrompt();
final String response;
if (isReplyPassword) {
response = this.cr.readLine(question, Character.valueOf(PASSWORD_MASK));
} else {
response = this.cr.readLine(question);
}
this.cr.setPrompt(prompt);
this.cr.setHistoryEnabled(true);
return response;
} catch (final IOException e) {
throw new ShellException(e);
}
}
}
/**
*
* Special notes for the implementation {@link PetalsInteractiveCli}: if the
* 'yes' flag is not enabled, the message is displayed, and the user is
* invited to reply by 'y' or 'n'.
*
*/
@Override
public boolean confirms(final String message) throws ShellException {
if (!super.confirms(message)) {
String confirmationResponse;
do {
confirmationResponse = this.askQuestion(message, false);
} while (!confirmationResponse.equals(YES) && !confirmationResponse.equals(NO));
return confirmationResponse.equals(YES);
}
return true;
}
@Override
public boolean isInteractive() {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy