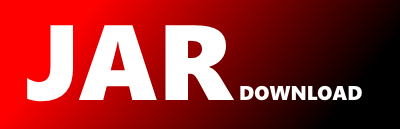
org.ow2.petals.cli.shell.ShellFactory Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2023 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell;
import java.io.IOException;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.OptionGroup;
import org.apache.commons.cli.ParseException;
import org.ow2.petals.cli.api.command.CommandRegistration;
import org.ow2.petals.cli.api.pref.Preferences;
import org.ow2.petals.cli.api.shell.ShellExtension;
import org.ow2.petals.cli.api.shell.exception.DuplicatedCommandException;
import org.ow2.petals.cli.shell.exception.ShellCreationException;
/**
* Shell factory creating the right shell according to provided on command line.
* @author Christophe DENEUX - Linagora
* @author Alexandre Lagane - Linagora
*/
public class ShellFactory {
public static final String MISSING_DOUBLE_DASH = "Missing -- before the command in parameter";
public static final String MISSING_COMMAND = "Missing command";
/**
* The short name of the option enabling debug information
*/
public static final String DEBUG_SHORT_OPTION = "d";
/**
* The long name of the option enabling debug information
*/
public static final String DEBUG_LONG_OPTION = "debug";
/**
* The short name of the 'yes' flag option
*/
public static final String YESFLAG_SHORT_OPTION = "y";
/**
* The long name of the 'yes' flag option
*/
public static final String YESFLAG_LONG_OPTION = "yes";
/**
* The short name of the option executing a command from the command line
*/
public static final String COMMAND_SHORT_OPTION = "c";
/**
* The short name of the option enabling the mode 'console'
*/
public static final String CONSOLE_SHORT_OPTION = "C";
/**
* The short name of the option enabling the file execution
*/
public static final String FILE_SHORT_OPTION = null;
/**
* The long name of the option enabling the file execution
*/
public static final String FILE_LONG_OPTION = "file";
private static final String USAGE = "[--" + FILE_LONG_OPTION + "] | -"
+ COMMAND_SHORT_OPTION + " -- | -" + CONSOLE_SHORT_OPTION + "]";
public static final ShellFactory INSTANCE = new ShellFactory();
private ShellFactory() {
// NOP
}
public static ShellFactory getInstance() {
return INSTANCE;
}
public static void addShellOptions(final OptionGroup optionsGrp) {
optionsGrp.addOption(Option.builder(CONSOLE_SHORT_OPTION).longOpt("console").hasArg(false)
.desc("Enable the mode 'console'.").build());
optionsGrp.addOption(Option.builder(FILE_SHORT_OPTION).argName("filename").longOpt(FILE_LONG_OPTION).hasArg()
.desc("Enable the script file execution. If filename is '-', commands are read from the stdin.")
.build());
optionsGrp.addOption(Option.builder(COMMAND_SHORT_OPTION).longOpt("command")
.desc("Execute a command given on the command line after '--'.").build());
}
public static String getUsage() {
return USAGE;
}
/**
*
* Create a new shell from arguments of the command line, and register all commands available on classpath:
*
*
* - - "-c": command execution on command line ({@link PetalsCli},
* - - "-C": interactive mode ({@link PetalsInteractiveCli},
* - - "--file": file mode ({@link PetalsScriptShell},
*
*
* @param cmdLine
* The command line parsed
* @param args
* Initial arguments of the command line
* @param preferences
* Preferences
* @param shellExtensions
* A list of shell extension, or null
if no shell extension is available
* @param basePrompt
* The base prompt
* @return
* @throws DuplicatedCommandException
* A command has been registered twice. As it's a hard coded registration, it's a BIG bug.
* @throws ParseException
* An error occurs about provided options or arguments.
* @throws ShellCreationException
* An error occurs creating the shell
*/
public AbstractShell newShell(final CommandLine cmdLine, final String[] args, final Preferences preferences,
final ShellExtension[] shellExtensions, final String basePrompt) throws DuplicatedCommandException,
ParseException, ShellCreationException {
final AbstractShell shell;
if (cmdLine.hasOption(COMMAND_SHORT_OPTION)) {
final String[] commandArgs = cmdLine.getArgs();
if (!checkDoubleDash(args, commandArgs)) {
throw new ParseException(MISSING_DOUBLE_DASH);
}
if (commandArgs.length == 0) {
throw new ParseException(MISSING_COMMAND);
}
shell = new PetalsCli(commandArgs, cmdLine.hasOption(DEBUG_SHORT_OPTION),
cmdLine.hasOption(YESFLAG_SHORT_OPTION), preferences, shellExtensions, basePrompt);
} else if (cmdLine.hasOption(CONSOLE_SHORT_OPTION)) {
try {
shell = new PetalsInteractiveCli(cmdLine.hasOption(DEBUG_SHORT_OPTION),
cmdLine.hasOption(YESFLAG_SHORT_OPTION), preferences, shellExtensions, basePrompt);
} catch (final IOException e) {
throw new ShellCreationException(e);
}
} else if (cmdLine.hasOption(FILE_LONG_OPTION)) {
shell = new PetalsFileScriptShell(cmdLine.getOptionValue(FILE_LONG_OPTION), cmdLine.getArgs(),
cmdLine.hasOption(DEBUG_SHORT_OPTION), cmdLine.hasOption(YESFLAG_SHORT_OPTION), preferences,
shellExtensions,
basePrompt);
} else {
// File mode
final String[] remainingArgs = cmdLine.getArgs();
if (remainingArgs.length > 0) {
final String filename = remainingArgs[0];
if ("-".equals(filename)) {
// Stdin mode
shell = new PetalsInlinedScriptShell(remainingArgs,
cmdLine.hasOption(DEBUG_SHORT_OPTION),
cmdLine.hasOption(YESFLAG_SHORT_OPTION), preferences, shellExtensions, basePrompt);
} else {
// File mode
shell = new PetalsFileScriptShell(filename, remainingArgs,
cmdLine.hasOption(DEBUG_SHORT_OPTION),
cmdLine.hasOption(YESFLAG_SHORT_OPTION), preferences, shellExtensions, basePrompt);
}
} else {
// A file name is missing
shell = null;
}
}
if (shell != null) {
CommandRegistration.registers(shell);
}
return shell;
}
/**
* Return true if double dash ({@code --}) is present in the right position when executing command from the command
* line, else return false
*
* @param args
* Initial arguments of the command line
* @param commandArgs
* Left-over non-recognized options and arguments after parsing
* @return true if double dash is present in the right position, else false
*/
private boolean checkDoubleDash(String[] args, String[] commandArgs) {
return args[args.length - 1 - commandArgs.length].equals("--");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy