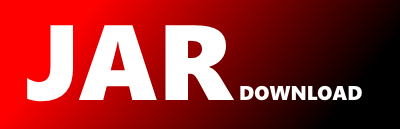
org.ow2.petals.cli.connection.AuthenticatedConnectionParametersImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.connection;
import java.net.UnknownHostException;
import org.ow2.petals.cli.api.connection.AuthenticatedConnectionParameters;
import org.ow2.petals.cli.api.connection.exception.HostMissingException;
import org.ow2.petals.cli.api.connection.exception.InvalidPortException;
import org.ow2.petals.cli.api.connection.exception.PasswordMissingException;
import org.ow2.petals.cli.api.connection.exception.UsernameMissingException;
public class AuthenticatedConnectionParametersImpl extends AuthenticatedConnectionParameters {
private final String username;
private final String password;
private final String passPhrase;
/**
*
* @param host
* @param port
* @param username
* Username. Can not be null
* @param password
* Password. Can not be null
* @param passPhrase
* Password. Can be null
* @throws UnknownHostException
* The host name can not be determined, or the IP address is invalid.
* @throws HostMissingException
* The host is null
* @throws InvalidPortException
* The host is invalid
* @throws UsernameMissingException
* The user name is null
* @throws PasswordMissingException
* The password is null
*/
public AuthenticatedConnectionParametersImpl(final String host, final int port, final String username,
final String password, final String passPhrase) throws UnknownHostException, HostMissingException,
InvalidPortException, UsernameMissingException, PasswordMissingException {
super(host, port);
if (username == null) {
throw new UsernameMissingException();
}
if (password == null) {
throw new PasswordMissingException();
}
this.username = username;
this.password = password;
this.passPhrase = passPhrase;
}
public String getUsername() {
return this.username;
}
public String getPassword() {
return this.password;
}
public String getPassPhrase() {
return this.passPhrase;
}
@Override
public int hashCode() {
final int prime = 31;
int result = super.hashCode();
assert this.username != null : "username is not null, see constructor";
assert this.password != null : "password is not null, see constructor";
result = prime * result + (this.passPhrase.hashCode());
result = prime * result + (this.password.hashCode());
result = prime * result + ((this.username == null) ? 0 : this.username.hashCode());
return result;
}
@Override
public boolean equals(final Object obj) {
if (this == obj)
return true;
if (!super.equals(obj))
return false;
if (getClass() != obj.getClass())
return false;
assert this.username != null : "username is not null, see constructor";
assert this.password != null : "password is not null, see constructor";
final AuthenticatedConnectionParametersImpl other = (AuthenticatedConnectionParametersImpl) obj;
if (this.passPhrase == null) {
if (other.passPhrase != null) {
return false;
}
} else if (!this.passPhrase.equals(other.passPhrase)) {
return false;
}
if (!this.password.equals(other.password)) {
return false;
}
if (!this.username.equals(other.username)) {
return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy