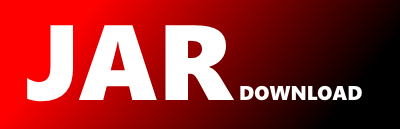
org.ow2.petals.cli.http.EmbeddedHttpServerImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2014-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.http;
import java.net.InetAddress;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.UnknownHostException;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.logging.Logger;
import javax.servlet.Servlet;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.servlet.ServletContextHandler;
import org.eclipse.jetty.servlet.ServletHolder;
import org.eclipse.jetty.util.log.Log;
import org.ow2.petals.cli.api.exception.EmbeddedHttpServerException;
import org.ow2.petals.cli.api.http.EmbeddedHttpServer;
import com.ebmwebsourcing.easycommons.uuid.SimpleUUIDGenerator;
/**
* Implementation of the embedded HTTP server required to deploy artifacts on remote containers
*
* @author Christophe DENEUX - Linagora
*/
public class EmbeddedHttpServerImpl implements EmbeddedHttpServer {
private static final String CONTEXT = "/artifacts";
private Logger log = Logger.getLogger(EmbeddedHttpServerImpl.class.getName());
/**
* The context of the collection of artifact URL registered
*/
private final ServletContextHandler artifactContext;
/**
* The configuration of the embedded HTTP server
*/
private final EmbeddedHttpServerConfig embeddedHttpServerConfig;
/**
* The Jetty HTTP server
*/
private Server server;
/**
* A lock required to control start/stop of the server
*/
private final Object serverStarterLock = new Object();
private final ConcurrentMap registeredArtifacts = new ConcurrentHashMap();
/**
* Creates a new instance of a temporary HTTP server
*
* @param httpPort
* HTTP listening port
*/
public EmbeddedHttpServerImpl(final EmbeddedHttpServerConfig embeddedHttpServerConfig) {
Log.setLog(new JettyLogger(log));
this.embeddedHttpServerConfig = embeddedHttpServerConfig;
this.server = new Server(embeddedHttpServerConfig.getHttpPort());
this.artifactContext = new ServletContextHandler();
this.artifactContext.setContextPath(CONTEXT);
this.server.setHandler(this.artifactContext);
}
private void start() throws Exception {
this.log.fine("Starting the embedded HTTP server ...");
this.server.start();
this.log.fine("Embedded HTTP server started");
}
private void stop() throws Exception {
this.log.fine("Stopping the embedded HTTP server ...");
this.server.stop();
this.server.destroy();
this.log.fine("Embedded HTTP server stopped");
}
@Override
public URL registerArtifactUrl(final URL artifactUrl) throws EmbeddedHttpServerException {
final Servlet servlet = new DownloadingArtifactServlet(artifactUrl);
final ServletHolder servletHolder = new ServletHolder(servlet);
final String uid = new SimpleUUIDGenerator().getNewID();
this.artifactContext.addServlet(servletHolder, "/" + uid + ".zip");
try {
this.registeredArtifacts.put(artifactUrl.toURI(), servlet);
synchronized (this.serverStarterLock) {
if (this.registeredArtifacts.size() == 1) {
try {
this.start();
} catch (final Exception e) {
throw new EmbeddedHttpServerException("An error occurs starting the embedding HTTP server", e);
}
}
}
try {
return new URL("http", InetAddress.getLocalHost().getHostAddress(),
this.embeddedHttpServerConfig.getHttpPort(), CONTEXT + "/" + uid + ".zip");
} catch (final MalformedURLException e) {
throw new EmbeddedHttpServerException("An error occurs computing the rewriten artifact URL", e);
} catch (final UnknownHostException e) {
throw new EmbeddedHttpServerException("An error occurs computing the rewriten artifact URL", e);
}
} catch (final URISyntaxException e) {
throw new EmbeddedHttpServerException("The URL '" + artifactUrl.toString()
+ "'can not be converted into URI", e);
}
}
public void unregisterArtifactUrl(final URL artifactUrl) throws EmbeddedHttpServerException {
try {
final Servlet servlet = this.registeredArtifacts.remove(artifactUrl.toURI());
servlet.destroy();
synchronized (this.serverStarterLock) {
if (this.registeredArtifacts.isEmpty()) {
try {
this.stop();
} catch (final Exception e) {
throw new EmbeddedHttpServerException("An error occurs stopping the embedding HTTP server", e);
}
}
}
} catch (final URISyntaxException e) {
throw new EmbeddedHttpServerException("The URL '" + artifactUrl.toString()
+ "'can not be converted into URI", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy