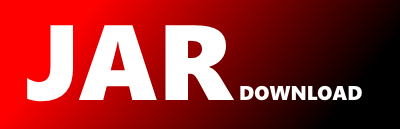
org.ow2.petals.cli.shell.command.Deploy Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell.command;
import static org.ow2.petals.cli.Constants.CommonOption.URL_OPTION;
import static org.ow2.petals.cli.Constants.CommonOption.URL_SHORT_OPTION;
import java.io.File;
import java.io.IOException;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.MissingArgumentException;
import org.apache.commons.cli.MissingOptionException;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.OptionGroup;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.commons.cli.UnrecognizedOptionException;
import org.ow2.petals.admin.api.ArtifactAdministration;
import org.ow2.petals.admin.api.PetalsAdministrationFactory;
import org.ow2.petals.admin.api.conf.PetalsAdminConfiguration;
import org.ow2.petals.admin.api.exception.ArtifactAdministrationException;
import org.ow2.petals.admin.api.exception.DuplicatedServiceException;
import org.ow2.petals.admin.api.exception.MissingServiceException;
import org.ow2.petals.cli.Constants;
import org.ow2.petals.cli.api.command.AbstractCommand;
import org.ow2.petals.cli.api.command.CommandUtil;
import org.ow2.petals.cli.api.command.exception.CommandBadArgumentNumberException;
import org.ow2.petals.cli.api.command.exception.CommandException;
import org.ow2.petals.cli.api.command.exception.CommandInvalidException;
import org.ow2.petals.cli.api.command.exception.CommandMissingArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingOptionsException;
import org.ow2.petals.cli.api.command.exception.CommandTooManyArgumentsException;
import org.ow2.petals.cli.api.command.exception.CommandUnparsableArgumentException;
import org.ow2.petals.cli.shell.util.ArtifactUrlRewriter;
import org.ow2.petals.cli.shell.util.Utils;
import jline.console.completer.FileNameCompleter;
/**
* This command deploys a JBI artifact on petals ESB.
* @author Sebastien Andre - EBM WebSourcing
*/
public class Deploy extends AbstractCommand {
public final static String PROPERTIES_FILE_SHORT_OPTION = "f";
public final static String PROPERTIES_FILE_LONG_OPTION = "file";
public final static String INLINED_PROPERTIES_SHORT_OPTION = "D";
protected static final Option INLINED_PROPERTIES_OPTION = Option.builder(INLINED_PROPERTIES_SHORT_OPTION)
.argName("property1=value1,property2=value2").hasArgs().valueSeparator(',')
.desc("List of '=', separated by a comma character, where is the name of the property to configure with . This argument is used only if the artifact is a component. This argument is exclusive with .")
.build();
protected static final Option PROPERTIES_FILE_OPTION = Option.builder(PROPERTIES_FILE_SHORT_OPTION).numberOfArgs(1)
.argName("configuration-file").longOpt(PROPERTIES_FILE_LONG_OPTION)
.desc("The local file name or the URL of the properties file used to configure the artifact. This argument is used only if the artifact is a component. This argument is exclusive with .")
.build();
/**
* The bulk option
*/
public final static String BULK_SHORT_OPTION = Constants.CommonOption.BULK_SHORT_OPTION;
private final static String BULK_LONG_OPTION = Constants.CommonOption.BULK_LONG_OPTION;
protected static final Option BULK_OPTION = Option.builder(BULK_SHORT_OPTION).numberOfArgs(1).argName("url")
.longOpt(BULK_LONG_OPTION).desc("Deploy and start artifacts found in the given directory").build();
/**
* The option to skip startup
*/
private final static String SKIP_START_SHORT_OPTION = "s";
public final static String SKIP_START_LONG_OPTION = "skip-start";
private final static Option SKIP_START_OPTION = Option.builder(SKIP_START_SHORT_OPTION).hasArg(false)
.longOpt(SKIP_START_LONG_OPTION).desc("Does not execute the startup during deploiement").build();
public Deploy() {
super();
this.setUsage(CommandUtil.formatCommandUsage(this));
this.setDescription("Deploy and start a JBI artifact");
this.setOptionsDescription(CommandUtil.formatCommandOptionsDescription(this));
final FileNameCompleter c = new FileNameCompleter();
this.completers.put(BULK_SHORT_OPTION, c);
this.completers.put(URL_SHORT_OPTION, c);
this.completers.put(PROPERTIES_FILE_SHORT_OPTION, c);
}
@Override
public void doExecute(final String[] args) throws CommandException {
final CommandLineParser parser = new DefaultParser();
try {
final ArtifactAdministration artifactAdministration = PetalsAdministrationFactory.getInstance()
.newPetalsAdministrationAPI(new PetalsAdminConfiguration(new ArtifactUrlRewriter(this.getShell())))
.newArtifactAdministration();
final CommandLine cli = parser.parse(this.getOptions(), args);
// bulk deployment
if (cli.hasOption(BULK_SHORT_OPTION)) {
if (!this.checkArguments(args, 2, 2)) {
throw new CommandTooManyArgumentsException(this, args);
}
final URL bulk = Utils.toURL(cli.getOptionValue(BULK_SHORT_OPTION));
if ("file".equals(bulk.getProtocol())) {
final File filePath = new File(bulk.toURI());
artifactAdministration.deployAndStartArtifacts(Utils.getUrls(filePath),
cli.hasOption(SKIP_START_SHORT_OPTION));
} else {
throw new CommandException(this,
"Directory deployment is only allowed for file protocol");
}
} else if (cli.hasOption(URL_SHORT_OPTION)) {
final URL url = Utils.toURL(cli.getOptionValue(URL_SHORT_OPTION));
if (cli.hasOption(PROPERTIES_FILE_SHORT_OPTION)) {
if (!this.checkArguments(args, 2, 4)) {
throw new CommandTooManyArgumentsException(this, args);
}
final URL propertiesFileUrl = Utils.toURL(cli.getOptionValue(PROPERTIES_FILE_SHORT_OPTION));
artifactAdministration.deployAndStartArtifact(url, propertiesFileUrl,
cli.hasOption(SKIP_START_SHORT_OPTION));
} else if (cli.hasOption(INLINED_PROPERTIES_SHORT_OPTION)) {
final Properties configurationProperties = new Properties();
final String[] properties = cli.getOptionValues(INLINED_PROPERTIES_SHORT_OPTION);
for (final String propertyEntry : properties) {
final String[] property = propertyEntry.split("=");
if (property.length != 2) {
throw new CommandUnparsableArgumentException(this,
INLINED_PROPERTIES_OPTION,
propertyEntry, "Maybe a space was forgotten between -D and its arguments?");
}
configurationProperties.put(property[0], property[1]);
}
artifactAdministration.deployAndStartArtifact(url, configurationProperties,
cli.hasOption(SKIP_START_SHORT_OPTION));
} else {
if ((this.checkArguments(args, 2, 2) && !cli.hasOption(SKIP_START_SHORT_OPTION))
|| (this.checkArguments(args, 2, 3) && cli.hasOption(SKIP_START_SHORT_OPTION))) {
artifactAdministration.deployAndStartArtifact(url, cli.hasOption(SKIP_START_SHORT_OPTION));
} else {
throw new CommandTooManyArgumentsException(this, args);
}
}
}
else {
final List missingOptions = new ArrayList();
missingOptions.add(BULK_SHORT_OPTION);
missingOptions.add(URL_SHORT_OPTION);
throw new CommandMissingOptionsException(this, missingOptions);
}
} catch (final UnrecognizedOptionException e) {
throw new CommandBadArgumentNumberException(this, e);
} catch (final MissingArgumentException e) {
throw new CommandMissingArgumentException(this, e.getOption(), e);
} catch (final MissingOptionException e) {
throw new CommandMissingOptionsException(this, e.getMissingOptions(), e);
} catch (final ParseException e) {
throw new CommandInvalidException(this, e);
} catch (final ArtifactAdministrationException e) {
throw new CommandException(this, e);
} catch (final IOException e) {
throw new CommandException(this, e);
} catch (final DuplicatedServiceException e) {
throw new CommandException(this, e);
} catch (final MissingServiceException e) {
throw new CommandException(this, e);
} catch (final URISyntaxException e) {
throw new CommandException(this, e);
}
}
@Override
public Options createOptions() {
final Options options = new Options();
final OptionGroup optionGroup = new OptionGroup();
optionGroup.addOption(BULK_OPTION);
optionGroup.addOption(URL_OPTION);
final OptionGroup propertiesOptionGroup = new OptionGroup();
propertiesOptionGroup.addOption(PROPERTIES_FILE_OPTION);
propertiesOptionGroup.addOption(INLINED_PROPERTIES_OPTION);
options.addOptionGroup(optionGroup);
options.addOptionGroup(propertiesOptionGroup);
options.addOption(SKIP_START_OPTION);
return options;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy