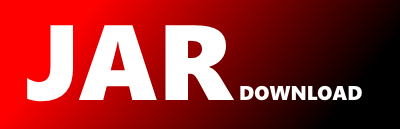
org.ow2.petals.cli.shell.command.Loggers Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell.command;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.MissingArgumentException;
import org.apache.commons.cli.MissingOptionException;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.commons.cli.UnrecognizedOptionException;
import org.ow2.petals.admin.api.ContainerAdministration;
import org.ow2.petals.admin.api.PetalsAdministrationFactory;
import org.ow2.petals.admin.api.artifact.Logger;
import org.ow2.petals.admin.api.exception.ContainerAdministrationException;
import org.ow2.petals.admin.api.exception.DuplicatedServiceException;
import org.ow2.petals.admin.api.exception.MissingServiceException;
import org.ow2.petals.cli.api.command.AbstractCommand;
import org.ow2.petals.cli.api.command.CommandUtil;
import org.ow2.petals.cli.api.command.exception.CommandBadArgumentNumberException;
import org.ow2.petals.cli.api.command.exception.CommandException;
import org.ow2.petals.cli.api.command.exception.CommandInvalidException;
import org.ow2.petals.cli.api.command.exception.CommandMissingArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingOptionsException;
import com.ebmwebsourcing.easycommons.lang.CollectionHelper;
import com.ebmwebsourcing.easycommons.lang.CollectionHelper.Filter;
import jline.console.completer.Completer;
import jline.console.completer.StringsCompleter;
/**
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class Loggers extends AbstractCommand {
static final String LOGGER_NAME_REGEXP_SHORT_OPTION = "p";
static final String LOGGER_NAME_REGEXP_LONG_OPTION = "pattern";
private static final Option LOGGER_NAME_REGEXP_OPTION = Option.builder(LOGGER_NAME_REGEXP_SHORT_OPTION)
.required(false).longOpt(LOGGER_NAME_REGEXP_LONG_OPTION).desc("The regexp to filter logger names.")
.numberOfArgs(1).argName("logger-name-regexp").build();
private final CommandLineParser clParser;
public Loggers() {
super();
this.clParser = new DefaultParser();
this.setUsage(CommandUtil.formatCommandUsage(this));
this.setDescription("Return all the loggers");
this.setOptionsDescription(CommandUtil.formatCommandOptionsDescription(this));
}
@Override
public void doExecute(final String[] args) throws CommandException {
try {
final CommandLine cli = this.clParser.parse(getOptions(), args, false);
final ContainerAdministration containerAdministration = PetalsAdministrationFactory
.getInstance().newPetalsAdministrationAPI().newContainerAdministration();
final Set loggers = new TreeSet(
containerAdministration.getLoggers());
if (cli.hasOption(LOGGER_NAME_REGEXP_SHORT_OPTION)) {
final String loggerNameRegex = cli.getOptionValue(LOGGER_NAME_REGEXP_SHORT_OPTION);
final List> filters = new ArrayList>();
filters.add(new RegexFilter(loggerNameRegex));
CollectionHelper.filter(loggers, filters);
} else if (args.length > 0) {
throw new CommandBadArgumentNumberException(this);
}
for (final Logger logger : loggers) {
final String loggerName = logger.getName();
this.getShell()
.getPrintStream()
.println(
(loggerName.isEmpty() ? "" : loggerName) + " "
+ logger.getLevel());
}
} catch (final UnrecognizedOptionException e) {
throw new CommandBadArgumentNumberException(this, e);
} catch (final MissingArgumentException e) {
throw new CommandMissingArgumentException(this, e.getOption(), e);
} catch (final MissingOptionException e) {
throw new CommandMissingOptionsException(this, e.getMissingOptions(), e);
} catch (final ParseException e) {
throw new CommandInvalidException(this, e);
} catch (final ContainerAdministrationException e) {
throw new CommandException(this, e);
} catch (final DuplicatedServiceException e) {
throw new CommandException(this, e);
} catch (final MissingServiceException e) {
throw new CommandException(this, e);
}
}
@Override
public Map getOptionCompleters() {
try {
final ContainerAdministration containerAdministration = PetalsAdministrationFactory
.getInstance().newPetalsAdministrationAPI().newContainerAdministration();
final List loggerNames = new LinkedList();
final List loggers = containerAdministration.getLoggers();
for (final Logger logger : loggers) {
loggerNames.add(logger.getName());
}
this.completers.put(LOGGER_NAME_REGEXP_SHORT_OPTION, new StringsCompleter(loggerNames));
} catch (final ContainerAdministrationException e) {
// no available completion
} catch (final DuplicatedServiceException e) {
// no available completion
} catch (final MissingServiceException e) {
// no available completion
}
return this.completers;
}
@Override
public Options createOptions() {
final Options options = new Options();
options.addOption(LOGGER_NAME_REGEXP_OPTION);
return options;
}
private class RegexFilter implements Filter {
private final Pattern pattern;
public RegexFilter(final String regex) {
this.pattern = Pattern.compile(regex);
}
@Override
public boolean accept(final Logger logger) {
final Matcher m = this.pattern.matcher(logger.getName());
return m.matches();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy