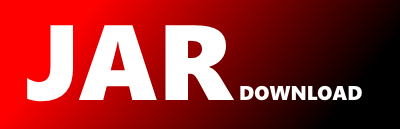
org.ow2.petals.cli.shell.command.MoveContainer Maven / Gradle / Ivy
/**
* Copyright (c) 2014-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell.command;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.MissingArgumentException;
import org.apache.commons.cli.MissingOptionException;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.commons.cli.UnrecognizedOptionException;
import org.ow2.petals.admin.api.ContainerAdministration;
import org.ow2.petals.admin.api.PetalsAdministrationFactory;
import org.ow2.petals.admin.api.exception.ContainerAdministrationException;
import org.ow2.petals.admin.api.exception.DuplicatedServiceException;
import org.ow2.petals.admin.api.exception.MissingServiceException;
import org.ow2.petals.cli.api.command.AbstractCommand;
import org.ow2.petals.cli.api.command.CommandUtil;
import org.ow2.petals.cli.api.command.exception.CommandBadArgumentNumberException;
import org.ow2.petals.cli.api.command.exception.CommandException;
import org.ow2.petals.cli.api.command.exception.CommandInvalidException;
import org.ow2.petals.cli.api.command.exception.CommandMissingArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingOptionsException;
import org.ow2.petals.cli.api.command.exception.CommandMissingYesFlagException;
import org.ow2.petals.cli.api.command.exception.CommandUnparsableArgumentException;
import org.ow2.petals.cli.api.shell.exception.ShellException;
import org.ow2.petals.jmx.api.api.JMXClient;
/**
* The Petals CLI command 'move-container'
* @author Christophe DENEUX - Linagora
*/
public class MoveContainer extends AbstractCommand {
/**
* The option defining the target domain name
*/
public static final String TARGET_DOMAIN_LONG_OPTION = "target-domain";
protected static final Option TARGET_DOMAIN_OPTION = Option.builder().required(false).numberOfArgs(1)
.argName("domain").longOpt(TARGET_DOMAIN_LONG_OPTION)
.desc("The target domain name, the one that will be joined.").build();
/**
* The option defining the target host name
*/
public static final String TARGET_HOST_LONG_OPTION = "target-host";
protected static final Option TARGET_HOST_OPTION = Option.builder().required(false).numberOfArgs(1)
.argName("hostname").longOpt(TARGET_HOST_LONG_OPTION)
.desc("The host name of the container of the target domain. Default value: localhost").build();
/**
* The option defining the jmx port of the target container
*/
public static final String TARGET_PORT_LONG_OPTION = "target-port";
protected static final Option TARGET_PORT_OPTION = Option.builder().required(false).numberOfArgs(1)
.argName("jmx-port").longOpt(TARGET_PORT_LONG_OPTION)
.desc("The JMX port of the container of the target domain. Default value: " + JMXClient.DEFAULT_PORT)
.build();
/**
* The option defining the jmx username of the target container
*/
public static final String TARGET_USER_LONG_OPTION = "target-user";
protected static final Option TARGET_USER_OPTION = Option.builder().required(false).numberOfArgs(1)
.argName("jmx-user").longOpt(TARGET_USER_LONG_OPTION)
.desc("The JMX username of the container of the target domain. Default value: petals").build();
/**
* The option defining the jmx password of the target container
*/
public static final String TARGET_PWD_LONG_OPTION = "target-pwd";
protected static final Option TARGET_PWD_OPTION = Option.builder().required(false).numberOfArgs(1)
.argName("jmx-password").longOpt(TARGET_PWD_LONG_OPTION)
.desc("The JMX password of the container of the target domain. Default value: petals").build();
public static final String ATTACH_CONFIRMATION_MSG = "Are you sure you want to move the current container? (y/n) ";
public static final String DETACH_CONFIRMATION_MSG = "Are you sure you want to detach the current container? (y/n) ";
/**
* The option defining the pass-phrase of the target container
*/
public static final String TARGET_PASSPHRASE_LONG_OPTION = "target-pass-phrase";
protected static final Option PASSPHRASE_OPTION = Option.builder().required(false).numberOfArgs(1)
.argName("target-pass-phrase").longOpt(TARGET_PASSPHRASE_LONG_OPTION)
.desc("The security pass-phrase to access sensible information.").build();
/**
* The confirmation skip option
*/
public static final String YESFLAG_SHORT_OPTION = "y";
private static final Option YESFLAG_OPTION = Option.builder(YESFLAG_SHORT_OPTION).hasArg(false)
.desc("A flag to skip confirmation.").build();
public MoveContainer() {
super("move-container");
this.setUsage(CommandUtil.formatCommandUsage(this));
this.setDescription("Move a container from a domain to another one.");
this.setOptionsDescription(CommandUtil.formatCommandOptionsDescription(this));
}
@Override
public void doExecute(final String[] args) throws CommandException {
final CommandLineParser parser = new DefaultParser();
try {
final CommandLine cli = parser.parse(this.getOptions(), args);
if (cli.hasOption(TARGET_DOMAIN_LONG_OPTION)) {
// Attaching or moving a container to a domain
final String targetDomainName = cli.getOptionValue(TARGET_DOMAIN_LONG_OPTION);
final String targetContainerHost;
if (cli.hasOption(TARGET_HOST_LONG_OPTION)) {
targetContainerHost = cli.getOptionValue(TARGET_HOST_LONG_OPTION);
} else {
targetContainerHost = "localhost";
}
final int targetContainerPort;
if (cli.hasOption(TARGET_PORT_LONG_OPTION)) {
final String targetContainerPortStr = cli.getOptionValue(TARGET_PORT_LONG_OPTION);
try {
targetContainerPort = Integer.parseInt(targetContainerPortStr);
} catch (final NumberFormatException e) {
throw new CommandUnparsableArgumentException(this, TARGET_PORT_OPTION, targetContainerPortStr);
}
} else {
targetContainerPort = JMXClient.DEFAULT_PORT;
}
final String targetContainerUser;
if (cli.hasOption(TARGET_USER_LONG_OPTION)) {
targetContainerUser = cli.getOptionValue(TARGET_USER_LONG_OPTION);
} else {
targetContainerUser = "petals";
}
final String targetContainerPwd;
if (cli.hasOption(TARGET_PWD_LONG_OPTION)) {
targetContainerPwd = cli.getOptionValue(TARGET_PWD_LONG_OPTION);
} else {
targetContainerPwd = "petals";
}
final String securityPassPhrase;
if (cli.hasOption(TARGET_PASSPHRASE_LONG_OPTION)) {
securityPassPhrase = cli.getOptionValue(TARGET_PASSPHRASE_LONG_OPTION);
} else {
final List missingOptions = new ArrayList<>();
missingOptions.add(TARGET_PASSPHRASE_LONG_OPTION);
throw new CommandMissingOptionsException(this, missingOptions);
}
if (!cli.hasOption(YESFLAG_SHORT_OPTION)) {
if (this.getShell().confirms(ATTACH_CONFIRMATION_MSG)) {
this.moveContainer(targetDomainName, targetContainerHost,
targetContainerPort, targetContainerUser, targetContainerPwd, securityPassPhrase);
} else if (!this.getShell().isInteractive()) {
throw new CommandMissingYesFlagException(this, YESFLAG_OPTION);
}
} else {
this.moveContainer(targetDomainName, targetContainerHost,
targetContainerPort, targetContainerUser, targetContainerPwd, securityPassPhrase);
}
} else if (!cli.hasOption(TARGET_DOMAIN_LONG_OPTION) && !cli.hasOption(TARGET_HOST_LONG_OPTION)
&& !cli.hasOption(TARGET_PORT_LONG_OPTION) && !cli.hasOption(TARGET_USER_LONG_OPTION)
&& !cli.hasOption(TARGET_PWD_LONG_OPTION) && !cli.hasOption(TARGET_PASSPHRASE_LONG_OPTION)) {
// Detaching a container from a domain
if (!cli.hasOption(YESFLAG_SHORT_OPTION)) {
if (this.getShell().confirms(DETACH_CONFIRMATION_MSG)) {
this.detachContainer();
} else if (!this.getShell().isInteractive()) {
throw new CommandMissingYesFlagException(this, YESFLAG_OPTION);
}
} else {
this.detachContainer();
}
} else {
throw new CommandBadArgumentNumberException(this);
}
} catch (final UnrecognizedOptionException e) {
throw new CommandBadArgumentNumberException(this, e);
} catch (final MissingArgumentException e) {
throw new CommandMissingArgumentException(this, e.getOption(), e);
} catch (final MissingOptionException e) {
throw new CommandMissingOptionsException(this, e.getMissingOptions(), e);
} catch (final ParseException e) {
throw new CommandInvalidException(this, e);
} catch (final ContainerAdministrationException e) {
throw new CommandException(this, e);
} catch (final ShellException e) {
throw new CommandException(this, e);
}
}
/**
* Move the container on which we are connected
*
* @see ContainerAdministration#attachContainer(String, String, int, String, String, String)
*/
private void moveContainer(final String targetDomainName, final String targetContainerHost,
final int targetContainerPort, final String targetContainerUser, final String targetContainerPwd,
final String securityPassPhrase) throws ContainerAdministrationException {
try {
final ContainerAdministration containerAdministration = PetalsAdministrationFactory.getInstance().newPetalsAdministrationAPI()
.newContainerAdministration();
containerAdministration.attachContainer(targetDomainName, targetContainerHost, targetContainerPort,
targetContainerUser, targetContainerPwd, securityPassPhrase);
this.getShell().setDefaultPrompt();
} catch (final DuplicatedServiceException e) {
throw new ContainerAdministrationException(e);
} catch (final MissingServiceException e) {
throw new ContainerAdministrationException(e);
}
}
/**
* Detach the container on which we are connected
*/
private void detachContainer() throws ContainerAdministrationException {
try {
final ContainerAdministration containerAdministration = PetalsAdministrationFactory.getInstance().newPetalsAdministrationAPI()
.newContainerAdministration();
containerAdministration.detachContainer();
this.getShell().setDefaultPrompt();
} catch (final DuplicatedServiceException e) {
throw new ContainerAdministrationException(e);
} catch (final MissingServiceException e) {
throw new ContainerAdministrationException(e);
}
}
@Override
public Options createOptions() {
final Options options = new Options();
options.addOption(TARGET_DOMAIN_OPTION);
options.addOption(TARGET_HOST_OPTION);
options.addOption(TARGET_PORT_OPTION);
options.addOption(TARGET_USER_OPTION);
options.addOption(TARGET_PWD_OPTION);
options.addOption(PASSPHRASE_OPTION);
options.addOption(YESFLAG_OPTION);
return options;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy