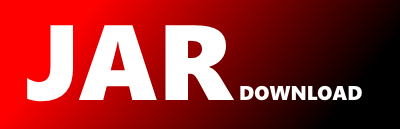
org.ow2.petals.cli.shell.command.ReloadConfiguration Maven / Gradle / Ivy
/**
* Copyright (c) 2015-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell.command;
import static org.ow2.petals.cli.Constants.CommonOption.URL_OPTION;
import static org.ow2.petals.cli.Constants.CommonOption.URL_SHORT_OPTION;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Map;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.MissingArgumentException;
import org.apache.commons.cli.MissingOptionException;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.commons.cli.UnrecognizedOptionException;
import org.ow2.petals.admin.api.ArtifactAdministration;
import org.ow2.petals.admin.api.PetalsAdministrationFactory;
import org.ow2.petals.admin.api.exception.ArtifactAdministrationException;
import org.ow2.petals.admin.api.exception.DuplicatedServiceException;
import org.ow2.petals.admin.api.exception.MissingServiceException;
import org.ow2.petals.cli.api.command.AbstractCommand;
import org.ow2.petals.cli.api.command.CommandUtil;
import org.ow2.petals.cli.api.command.exception.CommandBadArgumentNumberException;
import org.ow2.petals.cli.api.command.exception.CommandException;
import org.ow2.petals.cli.api.command.exception.CommandInvalidException;
import org.ow2.petals.cli.api.command.exception.CommandMissingArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingOptionsException;
import org.ow2.petals.cli.api.command.exception.CommandTooManyArgumentsException;
import org.ow2.petals.cli.shell.completer.StartedOrStoppedArtifactNameCompleter;
import org.ow2.petals.cli.shell.util.Utils;
import jline.console.completer.Completer;
import jline.console.completer.FileNameCompleter;
/**
* This command reload the placeholders configuration of a CDK-based JBI component.
*
* @author Christophe DENEUX - Linagora
*/
public class ReloadConfiguration extends AbstractCommand implements ArtifactBasedCommand {
private final ArtifactAdministration artifactAdministration;
public ReloadConfiguration() throws CommandException {
super("reload-configuration");
this.setUsage(CommandUtil.formatCommandUsage(this));
this.setDescription("Reload placeholders configuration a JBI component");
this.setOptionsDescription(CommandUtil.formatCommandOptionsDescription(this));
this.completers.put(URL_SHORT_OPTION, new FileNameCompleter());
try {
this.artifactAdministration = PetalsAdministrationFactory.getInstance().newPetalsAdministrationAPI()
.newArtifactAdministration();
} catch (final DuplicatedServiceException e) {
throw new CommandException(this, e);
} catch (final MissingServiceException e) {
throw new CommandException(this, e);
}
}
@Override
public void doExecute(final String[] args) throws CommandException {
final CommandLineParser parser = new DefaultParser();
try {
final CommandLine cl = parser.parse(getOptions(), args, true);
if (cl.hasOption(URL_SHORT_OPTION)) {
if (!this.checkArguments(args, 2, 2)) {
throw new CommandTooManyArgumentsException(this, args);
}
final URL artifactURL = Utils.toURL(cl.getOptionValue(URL_SHORT_OPTION));
this.artifactAdministration.reloadConfiguration(artifactURL);
} else if (cl.hasOption(ARTIFACT_SHORT_OPTION)) {
if (!this.checkArguments(args, 2, 2)) {
throw new CommandTooManyArgumentsException(this, args);
}
this.artifactAdministration.reloadConfiguration(cl.getOptionValue(ARTIFACT_SHORT_OPTION));
} else {
throw new CommandBadArgumentNumberException(this);
}
} catch (final UnrecognizedOptionException e) {
throw new CommandBadArgumentNumberException(this, e);
} catch (final MissingArgumentException e) {
throw new CommandMissingArgumentException(this, e.getOption(), e);
} catch (final MissingOptionException e) {
throw new CommandMissingOptionsException(this, e.getMissingOptions(), e);
} catch (final ParseException e) {
throw new CommandInvalidException(this, e);
} catch (final ArtifactAdministrationException e) {
throw new CommandException(this, e);
} catch (final MalformedURLException e) {
throw new CommandException(this, e);
}
}
@Override
public Options createOptions() {
final Options options = new Options();
options.addOption(ARTIFACT_OPTION);
options.addOption(URL_OPTION);
return options;
}
@Override
public Map getOptionCompleters() {
this.completers.put(ARTIFACT_SHORT_OPTION, new StartedOrStoppedArtifactNameCompleter(
this.artifactAdministration));
return this.completers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy