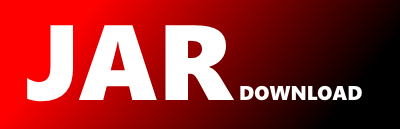
org.ow2.petals.cli.shell.command.Stop Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell.command;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.MissingArgumentException;
import org.apache.commons.cli.MissingOptionException;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.commons.cli.UnrecognizedOptionException;
import org.ow2.petals.admin.api.ContainerAdministration;
import org.ow2.petals.admin.api.PetalsAdministrationFactory;
import org.ow2.petals.admin.api.exception.ContainerAdministrationException;
import org.ow2.petals.admin.api.exception.DuplicatedServiceException;
import org.ow2.petals.admin.api.exception.MissingServiceException;
import org.ow2.petals.cli.Constants;
import org.ow2.petals.cli.api.command.AbstractCommand;
import org.ow2.petals.cli.api.command.CommandUtil;
import org.ow2.petals.cli.api.command.exception.CommandBadArgumentNumberException;
import org.ow2.petals.cli.api.command.exception.CommandException;
import org.ow2.petals.cli.api.command.exception.CommandInvalidException;
import org.ow2.petals.cli.api.command.exception.CommandMissingArgumentException;
import org.ow2.petals.cli.api.command.exception.CommandMissingOptionsException;
import org.ow2.petals.cli.api.command.exception.CommandMissingYesFlagException;
import org.ow2.petals.cli.api.shell.Shell;
import org.ow2.petals.cli.api.shell.exception.ShellException;
/**
* This command stop a petals ESB container.
*
* @author Sebastien Andre - EBM WebSourcing
* @author Nicolas Oddoux - EBM WebSourcing
*/
public class Stop extends AbstractCommand {
/**
* The shutdown option
*/
public static final String SHUTDOWN_SHORT_OPTION = "s";
private static final String SHUTDOWN_LONG_OPTION = "shutdown";
private static final Option SHUTDOWN_OPTION = Option.builder(SHUTDOWN_SHORT_OPTION).hasArg(false)
.longOpt(SHUTDOWN_LONG_OPTION).desc("A flag to skip confirmation.").build();
/**
* The confirmation skip option
*/
public final static String YESFLAG_SHORT_OPTION = "y";
private static final Option YESFLAG_OPTION = Option.builder(YESFLAG_SHORT_OPTION).hasArg(false)
.desc("A flag to skip confirmation.").build();
public final static String SHUTDOWN_CONFIRMATION_MSG = "Are you sure you want to shutdown the container? (y/n) ";
public Stop() {
super();
this.setUsage(CommandUtil.formatCommandUsage(this));
this.setDescription("Stop the container");
}
@Override
public void doExecute(final String[] args) throws CommandException {
try {
final CommandLineParser parser = new DefaultParser();
final CommandLine cmd = parser.parse(this.getOptions(), args);
final Shell shell = this.getShell();
if (!cmd.hasOption(SHUTDOWN_SHORT_OPTION)) {
if (cmd.hasOption(YESFLAG_SHORT_OPTION)) {
throw new CommandInvalidException(this, String.format(
Constants.CommandMessages.UNRECOGNIZED_OPTION, YESFLAG_SHORT_OPTION));
}
this.stopContainer();
} else {
if (!cmd.hasOption(YESFLAG_SHORT_OPTION)) {
if (shell.confirms(SHUTDOWN_CONFIRMATION_MSG)) {
this.shutdownContainer();
} else if (!shell.isInteractive()) {
throw new CommandMissingYesFlagException(this, YESFLAG_OPTION);
}
} else {
this.shutdownContainer();
}
}
} catch (final UnrecognizedOptionException e) {
throw new CommandBadArgumentNumberException(this, e);
} catch (final MissingArgumentException e) {
throw new CommandMissingArgumentException(this, e.getOption(), e);
} catch (final MissingOptionException e) {
throw new CommandMissingOptionsException(this, e.getMissingOptions(), e);
} catch (final ParseException e) {
throw new CommandInvalidException(this, e);
} catch (final ContainerAdministrationException e) {
throw new CommandException(this, e);
} catch (final ShellException e) {
throw new CommandException(this, e);
}
}
/**
* Stop the container on which we are connected
*
* @throws ContainerAdministrationException
* An error occurs during the stop
*/
private void stopContainer() throws ContainerAdministrationException {
try {
final ContainerAdministration containerAdministration = PetalsAdministrationFactory
.getInstance().newPetalsAdministrationAPI().newContainerAdministration();
containerAdministration.stopContainer();
this.getShell().setDefaultPrompt();
} catch (final DuplicatedServiceException e) {
throw new ContainerAdministrationException(e);
} catch (final MissingServiceException e) {
throw new ContainerAdministrationException(e);
}
}
/**
* Shutdown the container on which we are connected
*
* @throws ContainerAdministrationException
* An error occurs during the shutdown
*/
private void shutdownContainer() throws ContainerAdministrationException {
try {
final ContainerAdministration containerAdministration = PetalsAdministrationFactory
.getInstance().newPetalsAdministrationAPI().newContainerAdministration();
containerAdministration.shutdownContainer();
this.getShell().setDefaultPrompt();
} catch (final DuplicatedServiceException e) {
throw new ContainerAdministrationException(e);
} catch (final MissingServiceException e) {
throw new ContainerAdministrationException(e);
}
}
@Override
public Options getOptions() {
final Options options = new Options();
options.addOption(YESFLAG_OPTION);
options.addOption(SHUTDOWN_OPTION);
return options;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy