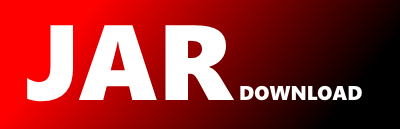
org.ow2.petals.cli.shell.util.Utils Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.cli.shell.util;
import java.io.File;
import java.io.FileFilter;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.regex.Pattern;
/**
* @author Mathieu CARROLLE - EBM WebSourcing
*/
public final class Utils {
private static final Pattern PROTO_PATTERN = Pattern.compile("^[a-zA-Z]+:/+");
private Utils() {
// nothing
}
/**
* Add file protocol, if no protocol is defined in the string.
*
* @param arg
* @return if arg
is an URL, this URL is returned, otherwise an URL associated to a local file is
* returned
* @throws MalformedURLException
*/
public static URL toURL(final String arg) throws MalformedURLException {
assert arg != null;
final URL url;
final boolean containsProtocol = PROTO_PATTERN.matcher(arg).find();
if (containsProtocol) {
url = new URL(arg);
} else {
url = toFileReplaceHome(arg).toURI().toURL();
}
return url;
}
/**
* @return null
if it's not a file
*/
public static File toFile(final String arg) {
assert arg != null;
final boolean containsProtocol = PROTO_PATTERN.matcher(arg).find();
if (containsProtocol) {
return null;
} else {
return toFileReplaceHome(arg);
}
}
private static File toFileReplaceHome(final String arg) {
final String path;
if (arg.startsWith("~" + File.separator)) {
path = arg.replaceFirst("~", System.getProperty("user.home"));
} else {
path = arg;
}
return new File(path);
}
/**
* List zip files in the specified directory and return an array of URLs
*
* @param directory
* @return return an array of URLs containing ZIP file
* @throws IOException
*/
public static URL[] getUrls(final File directory) throws IOException {
final FileFilter filter = new FileFilter() {
@Override
public boolean accept(File pathname) {
if (pathname.getAbsolutePath().toLowerCase().endsWith("zip")) {
return true;
}
return false;
}
};
final File[] files = directory.listFiles(filter);
if (files == null) {
throw new FileNotFoundException(directory.getAbsolutePath()
+ " (No such file or directory)");
}
final int length = files.length;
final URL[] urls = new URL[length];
for (int i = 0; i < length; i++) {
urls[i] = files[i].toURI().toURL();
}
return urls;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy