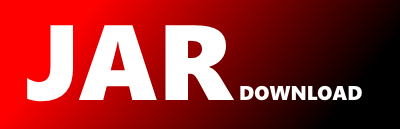
org.ow2.petals.log.handler.SourceHelpers Maven / Gradle / Ivy
/**
* Copyright (c) 2015-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.log.handler;
import java.io.File;
import java.io.FileWriter;
import java.util.logging.Handler;
import javax.xml.transform.Source;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.stream.StreamResult;
import org.apache.commons.pool.BasePoolableObjectFactory;
import org.apache.commons.pool.ObjectPool;
import org.apache.commons.pool.impl.GenericObjectPool;
/**
*
* Homemade version of easycommons-utils SourceHelper.
*
* Needed because we don't want easycommons-utils and its transformer pool arriving in the system classloader.
*
* This class should be used only by {@link PetalsPayloadDumperFileHandler}, so its visibility and methods' visibilities
* are restricted to package scope.
*
* @author vnoel
*
*/
class SourceHelpers {
private final ObjectPool transformers;
/**
*
* @param maxActive
* maximum number of instances
* @param maxIdle
* maximum number of idle instances
*/
public SourceHelpers(final int maxActive, final int maxIdle) {
this.transformers = new GenericObjectPool<>(new TransformersFactory(), maxActive,
GenericObjectPool.DEFAULT_WHEN_EXHAUSTED_ACTION, GenericObjectPool.DEFAULT_MAX_WAIT, maxIdle);
}
public void toFile(final Source source, final File fileToWrite) throws Exception {
final Transformer transformer = transformers.borrowObject();
try (final FileWriter writer = new FileWriter(fileToWrite)) {
transformer.transform(source, new StreamResult(writer));
writer.write("\n");
} finally {
transformers.returnObject(transformer);
}
}
private static class TransformersFactory extends BasePoolableObjectFactory {
/**
* this should be instantiated only once with the logging {@link Handler} that use {@link SourceHelpers} and
* thus using its classloader to choose the {@link TransformerFactory}.
*/
private final TransformerFactory factory = TransformerFactory.newInstance();
@Override
public Transformer makeObject() throws Exception {
return factory.newTransformer();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy