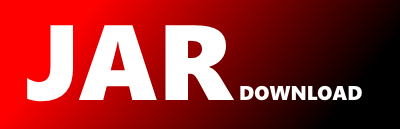
org.ow2.petals.microkernel.api.configuration.ContainerConfiguration Maven / Gradle / Ivy
/**
* Copyright (c) 2007-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.microkernel.api.configuration;
import java.net.URL;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import org.ow2.petals.clientserverapi.topology.TopologyService.ContainerPropertyDefaultValues;
import org.ow2.petals.clientserverapi.topology.TopologyService.ContainerPropertyName;
import org.ow2.petals.clientserverapi.topology.TopologyService.ContainerPropertyValues;
import org.ow2.petals.microkernel.api.communication.topology.TopologyService;
import org.ow2.petals.microkernel.api.jbi.management.recovery.SystemRecoveryService;
import org.ow2.petals.microkernel.api.jbi.messaging.RouterService;
import org.ow2.petals.microkernel.api.transport.FastRemoteTransportService;
/**
* This class holds the overall configuration of a PEtALS container.
*
* It stores both the local topology information and the global server properties information.
* The configuration service retrieves the configuration when it is started and creates an instance of this class.
*
*
* @author Adrien Louis - EBM WebSourcing
* @author Roland Naudin - EBM WebSourcing
* @author Christophe Hamerling - EBM WebSourcing
* @since Petals 2.0
*/
public final class ContainerConfiguration {
/**
* Container state enumeration.
*/
public enum ContainerState {
/**
* Started or reachable state
*/
REACHABLE,
/**
* Stopped or unreachable state
*/
UNREACHABLE,
/**
* Unknown state
*/
UNKNOWN
}
/**
* Default value of the timeout for processing the installation and deployment tasks.
*/
public static final long DEFAULT_INSTALLATION_DEPLOYMENT_TASK_TIMEOUT = 120000;
// ********** Global ************//
/**
* The location of the local configuration
*/
private URL serverPropertiesUrl;
/**
* Container name.
*/
private String name;
/**
* Container description
*/
private String description;
/**
* Host of the container.
*/
private String host;
/**
* State of the container.
*/
private ContainerState state = ContainerState.UNKNOWN;
/**
* Data root path
*/
private String dataRootPath;
/**
* Repository directory path.
*/
private String repositoryDirectoryPath;
/**
* The work directory where temporary things are stored
*/
private String workDirectoryPath;
/**
* Exchange validation.
* If true
, used by the Address resolver to know if a component
* is agree to receive a exchange from a consumer.
*/
private boolean exchangeValidation;
/**
* The timeout for processing the installation and deployment tasks.
*/
private long taskTimeout = DEFAULT_INSTALLATION_DEPLOYMENT_TASK_TIMEOUT;
/**
* Flag indicating if the JBI classLoaders must be isolated from the
* container ClassLoader.
*/
private boolean isolateJBIClassLoaders = true;
// ************ Admin **************//
/**
* Login to connect to the node.
*/
private String user;
/**
* Password to connect to the node.
*/
private String password;
/**
* Port of the RMI adaptor for JMX.
*/
private int jmxRMIConnectorPort;
// ***** Router *****//
/**
* Router Quality of Service. {@see RouterService}
*/
private String routerQOS = RouterService.DEFAULT_POLICY;
/**
* Router Strategy. {@see EndpointResolverModule}
*/
private String routerStrategy = RouterService.DEFAULT_PLATFORM_STRATEGY;
/**
* Number of attempt to send a message
*/
private short routerSendAttempt = RouterService.DEFAULT_SEND_ATTEMPTS;
/**
* Delay between each send attempt
*/
private int routerSendDelay = RouterService.DEFAULT_SEND_DELAY;
/**
* Delay to wait before to force to end pending exchanges when stopping the router.
*/
private long routerStopTrafficDelay = RouterService.DEFAULT_STOP_TRAFFIC_DELAY;
/**
* Delay to wait before to force to end pending exchanges when pausing the messaging sub-system.
*/
private long routerPauseTrafficDelay = RouterService.DEFAULT_PAUSE_TRAFFIC_DELAY;
// ***** Topology *****//
/**
* The topology pass-phrase
*/
private String topologyPassPhrase = null;
/**
* Max wait time to get a lock for the topology in the registry
*/
private int topologyLocksMaxWaitTime = TopologyService.DEFAULT_TOPOLOGY_LOCKS_MAX_WAIT_TIME;
/**
* Waiting time before to start the timer task associated to the topology pinger
*/
private long topologyPingerStartDelay = TopologyService.DEFAULT_TOPOLOGY_PINGER_START_DELAY;
/**
* Delay between two runs of the topology pinger
*/
private long topologyPingerPeriodDelay = TopologyService.DEFAULT_TOPOLOGY_PINGER_PERIOD_DELAY;
// ***** Transporter *****//
/**
* Port of the TCP communication.
*/
private int tcpPort = 0;
/**
* The interface(s) on which connection and data are received by receivers of the TCP transporter
*/
private String tcpListen = FastRemoteTransportService.DEFAULT_RECEIVER_LISTENING_ITF;
/**
* The SSL key password
*/
private String sslKeyPassword;
/**
* Max size of the transporter queues. Default value: 10000
*/
private int transporterQueueMaxSize = RouterService.DEFAULT_TRANSPORT_QUEUE_MAX_SIZE;
/**
* Offering timeout of component transporter queues, in milliseconds.
* Default value : 2500ms
*/
private long transporterQueueOfferingTimeout = RouterService.DEFAULT_TRANSPORT_QUEUE_OFFERING_TIMEOUT;
/**
* Number of message that can be received via TCP at the same time
*/
private int tcpReceivers = FastRemoteTransportService.DEFAULT_RECEIVERS;
/**
* Keep alive time, in milliseconds, before to stop unused TCP receivers
*/
private long tcpReceiversKeepAlive = FastRemoteTransportService.DEFAULT_RECEIVER_EVICTABLE_DELAY;
/**
* Number of message that can be send via TCP at the same time, per
* component
*/
private int tcpSenders = FastRemoteTransportService.DEFAULT_SENDERS;
/**
* TCP Connection timeout
*/
private long tcpConnectionTimeout = FastRemoteTransportService.DEFAULT_CONNECTION_TIMEOUT;
/**
* TCP send timeout
*/
private long tcpSendTimeout = FastRemoteTransportService.DEFAULT_SEND_TIMEOUT;
/**
* The delay before running the 'sender' eviction thread
*/
private long tcpSenderEvictorDelay = FastRemoteTransportService.DEFAULT_SEND_EVICTOR_DELAY;
/**
* The delay before an idle 'sender' is set evictable
*/
private long tcpSenderEvictableDelay = FastRemoteTransportService.DEFAULT_SEND_EVICTABLE_DELAY;
// ***** Security *****//
/**
* The SSL Keystore file
*/
private String sslKeystore;
/**
* The SSL Keystore password
*/
private String sslKeystorePassword;
/**
* The SSL Truststore file
*/
private String sslTruststore;
/**
* The SSL Truststore password
*/
private String sslTruststorePassword;
// ***** System recovery *****//
/**
* The core size of the system recovery thread pool
*/
private int recoveryCorePoolSize = SystemRecoveryService.DEFAULT_THREADPOOL_CORESIZE;
/**
* The keep alive time of the system recovery thread pool, in second
*/
private long recoveryKeepAliveTime = SystemRecoveryService.DEFAULT_THREADPOOL_KEEPALIVETIME;
/**
* The additional configuration in the server local configuration (ie. the
* content of 'server.properties
' or equivalent) defined by the
* user. Get all the 'user.*
' key/value pairs.
*/
private Map userConfiguration;
/**
* The additional configuration in the server local configuration (ie. the
* content of 'server.properties
' or equivalent) that is
* present but a priori not used.
*/
private Map extraConfiguration;
/**
* Default constructor
*/
public ContainerConfiguration() {
super();
}
/**
* Creates a {@link ContainerConfiguration} from a {@link Map}
*/
public ContainerConfiguration(final Map containerConfigurationMap) {
this.name = containerConfigurationMap.get(ContainerPropertyName.CONF_CONTAINER_NAME);
this.description = containerConfigurationMap.get(ContainerPropertyName.CONF_CONTAINER_DESCRIPTION);
this.host = containerConfigurationMap.get(ContainerPropertyName.CONF_HOST);
this.user = containerConfigurationMap.get(ContainerPropertyName.CONF_USER);
this.password = containerConfigurationMap.get(ContainerPropertyName.CONF_PWD);
final String jmxRMIConnectorPort = containerConfigurationMap.get(ContainerPropertyName.CONF_JMX_RMI_PORT);
this.jmxRMIConnectorPort = ContainerPropertyDefaultValues.CONF_JMX_RMI_PORT.equals(jmxRMIConnectorPort) ? 0
: Integer.parseInt(jmxRMIConnectorPort);
final String tcpPort = containerConfigurationMap.get(ContainerPropertyName.CONF_TRANSPORT_TCP_PORT);
this.tcpPort = ContainerPropertyDefaultValues.CONF_TRANSPORT_TCP_PORT.equals(tcpPort) ? 0 : Integer
.parseInt(tcpPort);
final String stateStr = containerConfigurationMap.get(ContainerPropertyName.CONF_STATE);
if (ContainerPropertyValues.STATE_REACHABLE.equals(stateStr)) {
this.state = ContainerState.REACHABLE;
} else if (ContainerPropertyValues.STATE_UNREACHABLE.equals(stateStr)) {
this.state = ContainerState.UNREACHABLE;
} else {
this.state = ContainerState.UNKNOWN;
}
}
/**
* @return the description
*/
public String getDescription() {
return this.description;
}
/**
* @return the host
*/
public String getHost() {
return this.host;
}
/**
* @return the jmxRMIConnectorPort
*/
public int getJmxRMIConnectorPort() {
return this.jmxRMIConnectorPort;
}
/**
* @return the name
*/
public String getName() {
return this.name;
}
/**
* @return the password
*/
public String getPassword() {
return this.password;
}
/**
* @return the repositoryDirectoryPath
*/
public String getRepositoryDirectoryPath() {
return this.repositoryDirectoryPath;
}
/**
* @return the routerQOS
*/
public String getRouterQOS() {
return this.routerQOS;
}
/**
* @return the routerSendAttempt
*/
public short getRouterSendAttempt() {
return this.routerSendAttempt;
}
/**
* @return the routerSendDelay
*/
public int getRouterSendDelay() {
return this.routerSendDelay;
}
/**
* @return the routerStopTrafficDelay
*/
public long getRouterStopTrafficDelay() {
return this.routerStopTrafficDelay;
}
/**
* @return the routerPauseTrafficDelay
*/
public long getRouterPauseTrafficDelay() {
return this.routerPauseTrafficDelay;
}
/**
* @return the routerStrategy
*/
public String getRouterStrategy() {
return this.routerStrategy;
}
/**
* @return the sslKeyPassword
*/
public String getSSLKeyPassword() {
return this.sslKeyPassword;
}
/**
* @return the sslKeystore
*/
public String getSSLKeystore() {
return this.sslKeystore;
}
/**
* @return the sslKeystorePassword
*/
public String getSSLKeystorePassword() {
return this.sslKeystorePassword;
}
/**
* @return the sslTruststore
*/
public String getSSLTruststore() {
return this.sslTruststore;
}
/**
* @return the sslTruststorePassword
*/
public String getSSLTruststorePassword() {
return this.sslTruststorePassword;
}
/**
* @return the state
*/
public ContainerState getState() {
return this.state;
}
/**
* @return the timeout for processing the installation and deployment tasks.
*/
public long getTaskTimeout() {
return this.taskTimeout;
}
/**
* @return the tcpConnectionTimeout
*/
public long getTCPConnectionTimeout() {
return this.tcpConnectionTimeout;
}
/**
* @return the tcpPort
*/
public int getTCPPort() {
return this.tcpPort;
}
public String getTCPListen() {
return this.tcpListen;
}
/**
* @return the tcpReceivers
*/
public int getTCPReceivers() {
return this.tcpReceivers;
}
/**
* @return the tcpReceiversKeepAlive
*/
public long getTCPReceiversKeepAlive() {
return this.tcpReceiversKeepAlive;
}
/**
* @return the tcpSenderEvictableDelay
*/
public long getTCPSenderEvictableDelay() {
return this.tcpSenderEvictableDelay;
}
/**
* @return the tcpSenderEvictorDelay
*/
public long getTCPSenderEvictorDelay() {
return this.tcpSenderEvictorDelay;
}
/**
* @return the tcpSenders
*/
public int getTCPSenders() {
return this.tcpSenders;
}
/**
* @return the tcpSendTimeout
*/
public long getTCPSendTimeout() {
return this.tcpSendTimeout;
}
public String getTopologyPassPhrase() {
return this.topologyPassPhrase;
}
/**
* @return The topology lock max wait time, in seconds
*/
public int getTopologyLocksMaxWaitTime() {
return this.topologyLocksMaxWaitTime;
}
/**
* @return The waiting time before to start the timer task associated to the topology pinger.
*/
public long getTopologyPingerStartDelay() {
return this.topologyPingerStartDelay;
}
/**
* @return The delay between two runs of the topology pinger.
*/
public long getTopologyPingerPeriodDelay() {
return this.topologyPingerPeriodDelay;
}
/**
* @return the user
*/
public String getUser() {
return this.user;
}
/**
* @return the userConfiguration
*/
public Map getUserConfiguration() {
return this.userConfiguration;
}
/**
* @return The extra properties. A properties is an extra property if not
* directly used by the micro-kernel but by an extension or by an
* dedicated Fractal component implementation. The {@link Map}
* returned is never null
, can be empty.
*/
public Map getExtraConfiguration() {
return this.extraConfiguration;
}
/**
* @return the workDirectoryPath
*/
public String getWorkDirectoryPath() {
return this.workDirectoryPath;
}
/**
* @return the exchangeValidation
*/
public boolean isExchangeValidation() {
return this.exchangeValidation;
}
/**
* @return the isolateJBIClassLoaders
*/
public boolean isIsolateJBIClassLoaders() {
return this.isolateJBIClassLoaders;
}
@Override
public String toString() {
StringBuilder toStringBuilder = new StringBuilder();
toStringBuilder.append("Container ").append(this.getName()).append(" on ")
.append(this.getHost());
if (this.getDescription() != null) {
toStringBuilder.append("\n\t\t\tDescription :\n\t\t\t ").append(this.getDescription());
}
toStringBuilder.append("\n\t\t\t - RepositoryDirectory: ").append(
this.getRepositoryDirectoryPath());
toStringBuilder.append("\n\t\t\t - WorkDirectory: ").append(
this.getWorkDirectoryPath());
toStringBuilder.append("\n\t\t\t - ExchangeValidation: ").append(
this.isExchangeValidation());
toStringBuilder.append("\n\t\t\t - RouterQOS: ").append(this.getRouterQOS());
toStringBuilder.append("\n\t\t\t - RouterStrategy: ").append(this.getRouterStrategy());
toStringBuilder.append("\n\t\t\t - RouterSendDelay: ").append(this.getRouterSendDelay());
toStringBuilder.append("\n\t\t\t - RouterSendAttempt: ").append(this.getRouterSendAttempt());
toStringBuilder.append("\n\t\t\t - RouterStopTrafficDelay: ").append(this.getRouterStopTrafficDelay());
toStringBuilder.append("\n\t\t\t - RouterPauseTrafficDelay: ").append(this.getRouterPauseTrafficDelay());
toStringBuilder.append("\n\t\t\t - TaskTimeout: ").append(this.getTaskTimeout());
toStringBuilder.append("\n\t\t\t - IsolateClassLoaders: ").append(
this.isIsolateJBIClassLoaders());
toStringBuilder.append("\n\t\t\t - User: ").append(
(this.getUser() != null) ? this.getUser() : "none");
if (this.getPassword() != null) {
StringBuilder hiddenPassword = new StringBuilder(this.getPassword().length());
while (hiddenPassword.length() < this.getPassword().length()) {
hiddenPassword.append("*");
}
toStringBuilder.append("\n\t\t\t - Password: ").append(hiddenPassword);
} else {
toStringBuilder.append("\n\t\t\t - Password: none");
}
toStringBuilder.append("\n\t\t\t - JMXRMIConnectionPort:").append(
this.getJmxRMIConnectorPort());
toStringBuilder.append("\n\t\t\t - TCPPort: ").append(
(this.getTCPPort() != 0) ? this.getTCPPort() : "none");
if (this.getTCPPort() != 0) {
toStringBuilder.append("\n\t\t\t - TCPListen:").append(this.getTCPListen());
}
if (this.getSSLKeyPassword() != null) {
StringBuilder hiddenPassword = new StringBuilder(this.getSSLKeyPassword().length());
while (hiddenPassword.length() < this.getSSLKeyPassword().length()) {
hiddenPassword.append("*");
}
toStringBuilder.append("\n\t\t\t - sslKeyPassword: ").append(hiddenPassword);
} else {
toStringBuilder.append("\n\t\t\t - sslKeyPassword: none");
}
toStringBuilder.append("\n\t\t\t - Keystore: ").append(
(this.getSSLKeystore() != null) ? this.getSSLKeystore() : "none");
if (this.getSSLKeystorePassword() != null) {
StringBuilder hiddenPassword = new StringBuilder(this.getSSLKeystorePassword().length());
while (hiddenPassword.length() < this.getSSLKeystorePassword().length()) {
hiddenPassword.append("*");
}
toStringBuilder.append("\n\t\t\t - KeystorePassword: ").append(hiddenPassword);
} else {
toStringBuilder.append("\n\t\t\t - KeystorePassword: none");
}
toStringBuilder.append("\n\t\t\t - Truststore: ").append(
(this.getSSLTruststore() != null) ? this.getSSLTruststore() : "none");
if (this.getSSLTruststorePassword() != null) {
StringBuilder hiddenPassword = new StringBuilder(this.getSSLTruststorePassword().length());
while (hiddenPassword.length() < this.getSSLTruststorePassword().length()) {
hiddenPassword.append("*");
}
toStringBuilder.append("\n\t\t\t - TruststorePassword: ").append(hiddenPassword);
} else {
toStringBuilder.append("\n\t\t\t - TruststorePassword: none");
}
if (this.getTCPPort() != 0) {
toStringBuilder.append("\n\t\t\t - TCPReceivers: ").append(this.getTCPReceivers());
toStringBuilder.append("\n\t\t\t - TCPReceiversKeepAlive: ").append(this.getTCPReceiversKeepAlive());
toStringBuilder.append("\n\t\t\t - TCPSenders: ").append(this.getTCPSenders());
toStringBuilder.append("\n\t\t\t - TCPConnectionTimeout: ").append(
this.getTCPConnectionTimeout());
toStringBuilder.append("\n\t\t\t - TCPSendTimeout: ").append(this.getTCPSendTimeout());
toStringBuilder.append("\n\t\t\t - TCPSenderEvictorDelay: ").append(
this.getTCPSenderEvictorDelay());
toStringBuilder.append("\n\t\t\t - TCPSenderEvictableDelay: ").append(
this.getTCPSenderEvictableDelay());
}
toStringBuilder.append("\n\t\t\t - TopologyLockMaxWaitTime: ")
.append(this.getTopologyLocksMaxWaitTime());
toStringBuilder.append("\n\t\t\t - TopologyPingerStartDelay: ").append(this.getTopologyPingerStartDelay());
toStringBuilder.append("\n\t\t\t - TopologyPingerPeriodDelay: ").append(this.getTopologyPingerPeriodDelay());
toStringBuilder.append("\n\t\t\t - State: ").append(this.getState().name());
if (this.getUserConfiguration() != null) {
StringBuilder userBuffer = new StringBuilder();
Iterator iter = this.getUserConfiguration().keySet().iterator();
while (iter.hasNext()) {
String key = iter.next();
userBuffer.append("\n\t\t\t\t").append(key).append('=')
.append(this.getUserConfiguration().get(key));
}
toStringBuilder.append("\n\t\t\t - User properties: ").append(userBuffer.toString());
}
return toStringBuilder.toString();
}
/**
* @param description
* the description to set
*/
public void setDescription(final String description) {
this.description = description;
}
/**
* @param exchangeValidation
* the exchangeValidation to set
*/
public void setExchangeValidation(final boolean exchangeValidation) {
this.exchangeValidation = exchangeValidation;
}
/**
* @param host
* the host to set
*/
public void setHost(final String host) {
this.host = host;
}
/**
* @param isolateJBIClassLoaders
* the isolateJBIClassLoaders to set
*/
public void setIsolateJBIClassLoaders(final boolean isolateJBIClassLoaders) {
this.isolateJBIClassLoaders = isolateJBIClassLoaders;
}
/**
* @param jmxRMIConnectorPort
* the jmxRMIConnectorPort to set
*/
public void setJmxRMIConnectorPort(final int jmxRMIConnectorPort) {
this.jmxRMIConnectorPort = jmxRMIConnectorPort;
}
/**
* @param name
* the name to set
*/
public void setName(final String name) {
this.name = name;
}
/**
* @param password
* the password to set
*/
public void setPassword(final String password) {
this.password = password;
}
/**
* @param repositoryDirectoryPath
* the repositoryDirectoryPath to set
*/
public void setRepositoryDirectoryPath(final String repositoryDirectoryPath) {
this.repositoryDirectoryPath = repositoryDirectoryPath;
}
/**
* @param routerQOS
* the routerQOS to set
*/
public void setRouterQOS(final String routerQOS) {
this.routerQOS = routerQOS;
}
/**
* @param routerSendAttempt
* the routerSendAttempt to set
*/
public void setRouterSendAttempt(final short routerSendAttempt) {
this.routerSendAttempt = routerSendAttempt;
}
/**
* @param routerSendDelay
* the routerSendDelay to set
*/
public void setRouterSendDelay(final int routerSendDelay) {
this.routerSendDelay = routerSendDelay;
}
/**
* @param routerStopTrafficDelay
* the routerStopTrafficDelay to set
*/
public void setRouterStopTrafficDelay(final long routerStopTrafficDelay) {
this.routerStopTrafficDelay = routerStopTrafficDelay;
}
/**
* @param routerPauseTrafficDelay
* the routerPauseTrafficDelay to set
*/
public void setRouterPauseTrafficDelay(final long routerPauseTrafficDelay) {
this.routerPauseTrafficDelay = routerPauseTrafficDelay;
}
/**
* @param routerStrategy
* the routerStrategy to set
*/
public void setRouterStrategy(final String routerStrategy) {
this.routerStrategy = routerStrategy;
}
/**
* @param sslKeyPassword
* the sslKeyPassword to set
*/
public void setSSLKeyPassword(final String sslKeyPassword) {
this.sslKeyPassword = sslKeyPassword;
}
/**
* @param sslKeystore
* the sslKeystore to set
*/
public void setSSLKeystore(final String sslKeystore) {
this.sslKeystore = sslKeystore;
}
/**
* @param sslKeystorePassword
* the sslKeystorePassword to set
*/
public void setSSLKeystorePassword(final String sslKeystorePassword) {
this.sslKeystorePassword = sslKeystorePassword;
}
/**
* @param sslTruststore
* the sslTruststore to set
*/
public void setSSLTruststore(final String sslTruststore) {
this.sslTruststore = sslTruststore;
}
/**
* @param sslTruststorePassword
* the sslTruststorePassword to set
*/
public void setSSLTruststorePassword(final String sslTruststorePassword) {
this.sslTruststorePassword = sslTruststorePassword;
}
/**
* @param state
* the state to set
*/
public void setState(final ContainerState state) {
this.state = state;
}
/**
* @param taskTimeout
* the timeout for processing the installation and deployment tasks.
*/
public void setTaskTimeout(final long taskTimeout) {
this.taskTimeout = taskTimeout;
}
/**
* @param tcpConnectionTimeout
* the tcpConnectionTimeout to set
*/
public void setTCPConnectionTimeout(final long tcpConnectionTimeout) {
this.tcpConnectionTimeout = tcpConnectionTimeout;
}
/**
* @param tcpPort
* the tcpPort to set
*/
public void setTCPPort(final int tcpPort) {
this.tcpPort = tcpPort;
}
public void setTCPListen(final String tcpListen) {
this.tcpListen = tcpListen;
}
/**
* @param tcpReceivers
* the tcpReceivers to set
*/
public void setTCPReceivers(final int tcpReceivers) {
this.tcpReceivers = tcpReceivers;
}
/**
* @param tcpReceiversKeepAlive
* the tcpReceiversKeepAlive to set
*/
public void setTCPReceiversKeepAlive(final long tcpReceiversKeepAlive) {
this.tcpReceiversKeepAlive = tcpReceiversKeepAlive;
}
/**
* @param tcpSenderEvictableDelay
* the tcpSenderEvictableDelay to set
*/
public void setTCPSenderEvictableDelay(final long tcpSenderEvictableDelay) {
this.tcpSenderEvictableDelay = tcpSenderEvictableDelay;
}
/**
* @param tcpSenderEvictorDelay
* the tcpSenderEvictorDelay to set
*/
public void setTCPSenderEvictorDelay(final long tcpSenderEvictorDelay) {
this.tcpSenderEvictorDelay = tcpSenderEvictorDelay;
}
/**
* @param tcpSenders
* the tcpSenders to set
*/
public void setTCPSenders(final int tcpSenders) {
this.tcpSenders = tcpSenders;
}
/**
* @param tcpSendTimeout
* the tcpSendTimeout to set
*/
public void setTCPSendTimeout(final long tcpSendTimeout) {
this.tcpSendTimeout = tcpSendTimeout;
}
/**
*
* @param topologyPassPhrase
*/
public void setTopologyPassPhrase(final String topologyPassPhrase) {
this.topologyPassPhrase = topologyPassPhrase;
}
/**
* Set the topology lock max wait time
*
* @param topologyLocksMaxWaitTime
* The topology lock max wait time to set
*/
public void setTopologyLocksMaxWaitTime(final int topologyLocksMaxWaitTime) {
this.topologyLocksMaxWaitTime = topologyLocksMaxWaitTime;
}
/**
* Set the waiting time before to start the timer task associated to the topology pinger
*
* @param topologyPingerStartDelay
* The wait time to set
*/
public void setTopologyPingerStartDelay(final long topologyPingerStartDelay) {
this.topologyPingerStartDelay = topologyPingerStartDelay;
}
/**
* Set the delay between two runs of the topology pinger
*
* @param topologyPingerPeriodDelay
* The delay between two runs
*/
public void setTopologyPingerPeriodDelay(final long topologyPingerPeriodDelay) {
this.topologyPingerPeriodDelay = topologyPingerPeriodDelay;
}
/**
* @param user
* the user to set
*/
public void setUser(final String user) {
this.user = user;
}
/**
* @param userConfiguration
* the userConfiguration to set
*/
public void setUserConfiguration(final Map userConfiguration) {
this.userConfiguration = userConfiguration;
}
/**
* @param extraConfiguration
* the extraConfiguration to set
*/
public void setExtraConfiguration(final Map extraConfiguration) {
this.extraConfiguration = extraConfiguration;
}
/**
* @param workDirectoryPath
* the workDirectoryPath to set
*/
public void setWorkDirectoryPath(final String workDirectoryPath) {
this.workDirectoryPath = workDirectoryPath;
}
public int getRecoveryCorePoolSize() {
return recoveryCorePoolSize;
}
public void setRecoveryCorePoolSize(final int recoveryCorePoolSize) {
this.recoveryCorePoolSize = recoveryCorePoolSize;
}
public long getRecoveryKeepAliveTime() {
return recoveryKeepAliveTime;
}
public void setRecoveryKeepAliveTime(final long recoveryKeepAliveTime) {
this.recoveryKeepAliveTime = recoveryKeepAliveTime;
}
/**
* @return the transporterQueueMaxSize
*/
public int getTransporterQueueMaxSize() {
return transporterQueueMaxSize;
}
/**
* @param transporterQueueMaxSize
* the transporterQueueMaxSize to set
*/
public void setTransporterQueueMaxSize(final int transporterQueueMaxSize) {
this.transporterQueueMaxSize = transporterQueueMaxSize;
}
/**
* @return the transporterQueueOfferingTimeout
*/
public long getTransporterQueueOfferingTimeout() {
return transporterQueueOfferingTimeout;
}
/**
* @param transporterQueueOfferingTimeout
* the transporterQueueOfferingTimeout to set
*/
public void setTransporterQueueOfferingTimeout(final long transporterQueueOfferingTimeout) {
this.transporterQueueOfferingTimeout = transporterQueueOfferingTimeout;
}
/**
* @return the serverPropertiesUrl
*/
public URL getServerPropertiesUrl() {
return this.serverPropertiesUrl;
}
/**
* @param serverPropertiesUrl
* the serverPropertiesUrl to set
*/
public void setServerPropertiesUrl(final URL serverPropertiesUrl) {
this.serverPropertiesUrl = serverPropertiesUrl;
}
/**
* @return the dataRootPath
*/
public String getDataRootPath() {
return this.dataRootPath;
}
/**
* @param dataRootPath
* the dataRootPath to set
*/
public void setDataRootPath(final String dataRootPath) {
this.dataRootPath = dataRootPath;
}
/**
* Convert the container configuration into a key/value map form.
*
* @param isSecurityOk
* if true
, sensible information are returned. Otherwise, sensible information are skipped.
* @return The container configuration as key/value map form.
*/
public Map toMap(final boolean isSecurityOk) {
final Map containerMap = new HashMap<>();
containerMap.put(ContainerPropertyName.CONF_CONTAINER_NAME, this.getName());
if (this.getDescription() != null) {
containerMap.put(ContainerPropertyName.CONF_CONTAINER_DESCRIPTION, this.getDescription());
}
containerMap.put(ContainerPropertyName.CONF_HOST, this.getHost());
if (isSecurityOk) {
containerMap.put(ContainerPropertyName.CONF_USER, this.getUser());
containerMap.put(ContainerPropertyName.CONF_PWD, this.getPassword());
}
if (this.getJmxRMIConnectorPort() != 0) {
containerMap.put(ContainerPropertyName.CONF_JMX_RMI_PORT, Integer.toString(this.getJmxRMIConnectorPort()));
} else {
containerMap.put(ContainerPropertyName.CONF_JMX_RMI_PORT, ContainerPropertyDefaultValues.CONF_JMX_RMI_PORT);
}
if (this.getTCPPort() != 0) {
containerMap.put(ContainerPropertyName.CONF_TRANSPORT_TCP_PORT, Integer.toString(this.getTCPPort()));
} else {
containerMap.put(ContainerPropertyName.CONF_TRANSPORT_TCP_PORT,
ContainerPropertyDefaultValues.CONF_TRANSPORT_TCP_PORT);
}
if (ContainerConfiguration.ContainerState.REACHABLE.equals(this.getState())) {
containerMap.put(ContainerPropertyName.CONF_STATE, ContainerPropertyValues.STATE_REACHABLE);
} else {
containerMap.put(ContainerPropertyName.CONF_STATE, ContainerPropertyValues.STATE_UNREACHABLE);
}
return containerMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy