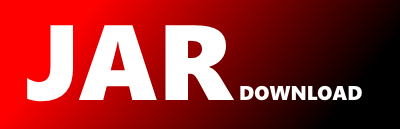
org.ow2.petals.microkernel.container.ContainerController Maven / Gradle / Ivy
/**
* Copyright (c) 2005-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.microkernel.container;
import org.ow2.petals.jbi.descriptor.original.generated.Jbi.SharedLibrary;
import org.ow2.petals.jbi.descriptor.original.generated.ServiceAssembly;
import org.ow2.petals.microkernel.api.container.ComponentLifeCycle;
import org.ow2.petals.microkernel.api.container.Installer;
import org.ow2.petals.microkernel.api.container.ServiceAssemblyLifeCycle;
import org.ow2.petals.microkernel.api.container.SharedLibraryLifeCycle;
import org.ow2.petals.microkernel.api.server.FractalHelper;
import org.ow2.petals.microkernel.container.exception.ContainerCtrlException;
/**
* The {@link ContainerController} manages Fractal components associated to JBI artifacts member of the Fractal
* composite {@link FractalHelper#CONTAINER_COMPOSITE}.
*
* @author Christophe DENEUX - Linagora
*/
public interface ContainerController {
/**
* Logger name of the container controller
*/
public static final String FRACTAL_COMPONENT_LOGGER_NAME = "Petals.Container.ContainerController";
/**
* Name of the server interface of the Fractal component {@value FractalHelper#CONTAINER_CONTROLLER_COMPONENT}
*/
public static final String FRACTAL_SRV_ITF_NAME = "service";
/**
* Prefix of the installer name.
*/
public static final String PREFIX_INSTALLER_NAME = "Installer.";
/**
* Prefix of the component life cycle name.
*/
public static final String PREFIX_COMPONENT_LIFE_CYCLE_NAME = "ComponentLifeCycle.";
/**
* Prefix of the service assembly life cycle name.
*/
public static final String PREFIX_SERVICE_ASSEMBLY_LIFE_CYCLE_NAME = "ServiceAssemblyLifeCycle.";
/**
* Prefix of the shared library life cycle name.
*/
public static final String PREFIX_SHARED_LIBRARY_LIFE_CYCLE_NAME = "SharedLibraryLifeCycle.";
/**
* Create a JBI component installer and its associated Fractal component, from its component description, and then
* starts it.
*
* @param componentDescription
* the component description of the JBI component
* @return the installer of the JBI component
* @throws ContainerCtrlException
* An error occurs when creating the Fractal component associated to the installer of the JBI component
*/
Installer createInstaller(final org.ow2.petals.jbi.descriptor.original.generated.Component componentDescription)
throws ContainerCtrlException;
/**
* Remove the Fractal component associated to the JBI component installer identified by its JBI component
* identifier.
*
* @param name
* The JBI component identifier
* @throws ContainerCtrlException
* An error occurs when removing the Fractal component associated to the JBI component installer
*/
void removeInstaller(final String name) throws ContainerCtrlException;
/**
* Create a JBI component life cycle and its associated Fractal component, from its component description, and then
* starts it.
*
* @param componentDescription
* the component description of the JBI component
* @return the life cycle of the JBI component
* @throws ContainerCtrlException
* An error occurs when creating the Fractal component associated to the life cycle of the JBI component
*/
ComponentLifeCycle createComponentLifeCycle(
final org.ow2.petals.jbi.descriptor.original.generated.Component componentDescription)
throws ContainerCtrlException;
/**
* Remove the Fractal component associated to the component life cycle of a JBI component identified by its JBI
* component identifier.
*
* @param name
* The JBI component identifier
* @throws ContainerCtrlException
* An error occurs when removing the Fractal component associated to the component life cycle of the JBI
* component
*/
void removeComponentLifeCycle(final String name) throws ContainerCtrlException;
/**
* Create the Fractal component associated to a life cycle of a JBI service assembly, and then starts it.
*
* @param sa
* the description of the JBI service assembly
* @return the Fractal component associated to the life cycle of the JBI service assembly
* @throws ContainerCtrlException
* An error occurs when creating the Fractal component associated to the life cycle of the JBI service
* assembly
*/
public ServiceAssemblyLifeCycle createServiceAssemblyLifeCycle(final ServiceAssembly sa)
throws ContainerCtrlException;
/**
* Remove the Fractal component associated to the life cycle of a JBI service assembly identified by its JBI
* identifier.
*
* @param saName
* The JBI service assembly identifier
* @throws ContainerCtrlException
* An error occurs when removing the Fractal component associated to the life cycle of the JBI service
* assembly
*/
void removeServiceAssemblyLifeCycle(final String saName) throws ContainerCtrlException;
/**
* Create the Fractal component associated to a life cycle of a JBI shared library, and then starts it.
*
* @param sl
* the description of the JBI shared library
* @return the Fractal component associated to the life cycle of the JBI shared library
* @throws ContainerCtrlException
* An error occurs when creating the Fractal component associated to the life cycle of the JBI shared
* library
*/
public SharedLibraryLifeCycle createSharedLibraryLifeCycle(final SharedLibrary sl) throws ContainerCtrlException;
/**
* Remove the Fractal component associated to a JBI shared library identified by its JBI identifier and its version.
*
* @param slName
* The JBI shared library identifier
* @param slVersion
* The shared library version
* @throws ContainerCtrlException
* An error occurs when removing the Fractal component associated to the JBI shared library
*/
void removeSharedLibraryLifeCycle(final String slName, final String slVersion) throws ContainerCtrlException;
/**
* Stop all the Fractal components associated to the JBI components, SAs and SLs
*
* @throws ContainerCtrlException
* An error occurs
*/
public void stopAllJbiArtefacts() throws ContainerCtrlException;
/**
* Start all the Fractal components associated to the JBI components, SAs and SLs
*
* @throws ContainerCtrlException
* An error occurs
*/
public void startAllJbiArtefacts() throws ContainerCtrlException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy