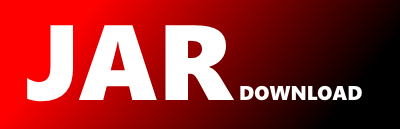
org.ow2.petals.microkernel.server.PetalsCompositeController Maven / Gradle / Ivy
/**
* Copyright (c) 2013-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.microkernel.server;
import java.util.Properties;
import org.ow2.petals.microkernel.api.server.FractalHelper;
import org.ow2.petals.microkernel.server.exception.PetalsCompositeCtrlException;
/**
*
* Controller of the Fractal composite 'Petals'.
*
*
* The Fractal composite 'Petals' is in charge of loading all Fractal architecture. The controller will load all the
* Fractal architecture except the component 'Configuration' according to the configuration.
*
*
* @author Christophe DENEUX - Linagora
*
*/
public interface PetalsCompositeController {
/**
* Logger name
*/
public static final String COMPONENT_LOGGER_NAME = "Petals.Server";
/**
* Name of the server interface of the Fractal component {@value FractalHelper#PETALS_COMPOSITE_CTRL_COMPONENT}
*/
public static final String FRACTAL_SRV_ITF_NAME = "service";
/**
* Name of the internal server interface 'sharedArea' of the composite 'SharedArea'
*/
public static final String SHARED_AREA_FRACTAL_INT_SRV_ITF_NAME = "service";
/**
* Name of the internal client interface 'configuration' of the composite 'SharedArea'
*/
public static final String CONFIGURATION_FRACTAL_INT_ITF_NAME = "configuration";
/**
* Stop and shutdown all the Fractal components associated to the JBI components and SAs
*
* @throws PetalsCompositeCtrlException
* An error occurs
*/
public void stopJbiArtefacts() throws PetalsCompositeCtrlException;
/**
* Stop the Fractal component {@link FractalHelper#ENDPOINT_DIRECTORY_COMPONENT}.
*
* @return An {@link AutoCloseable} to restart the component.
* @throws PetalsCompositeCtrlException
* An error occurs stopping {@link FractalHelper#ENDPOINT_DIRECTORY_COMPONENT}
*/
public AutoCloseable pauseEndpointDirectory() throws PetalsCompositeCtrlException;
/**
*
* Stop the Fractal sub-system to pause the message exchanges processing:
*
* - We wait for all pending exchange finish. If they are not terminated under a given duration, the remaining
* pending exchanges can be lost or can end with error,
* - and the composite {@link FractalHelper#TRANSPORTERS_COMPOSITE} is stopped to prevent all incoming message
* exchanges.
*
*
*
* @return An {@link AutoCloseable} to restart the component.
* @throws PetalsCompositeCtrlException
* An error occurs pausing the messaging sub-system
*/
public AutoCloseable pauseMessagingSubsystem() throws PetalsCompositeCtrlException;
/**
*
* Stop the Fractal component {@link FractalHelper#SHARED_AREA_COMPONENT}.
*
*
* @return An {@link AutoCloseable} to restart the component.
* @throws PetalsCompositeCtrlException
* An error occurs stopping the registry
*/
public AutoCloseable pauseSharedArea() throws PetalsCompositeCtrlException;
/**
*
* Retrieve the configuration of the shared area client mutable implementation as {@link Properties}. See API
* of the shared area client mutable implementation for the property list.
*
*
* All properties are prefixed by the class name of the shared area client mutable implementation
*
*
* @param providedTopologyPassphrase
* Passphrase needed to return sensible information. If null
, empty or not matching the one
* of the container on which the connection is established, no sensible information will be returned.
* @return The configuration of the shared area client mutable implementation as {@link Properties}. Not
* null
.
* @throws PetalsCompositeCtrlException
* An error occurs getting the configuration of the shared area client mutable implementation
*/
public Properties getSharedAreaCltConfiguration(final String providedTopologyPassphrase)
throws PetalsCompositeCtrlException;
/**
*
* Stop the Fractal component {@link FractalHelper#SYSTEMRECOVERY_COMPONENT}.
*
*
* @throws PetalsCompositeCtrlException
* An error occurs stopping the system recovery service
*/
public void stopSystemRecoveryService() throws PetalsCompositeCtrlException;
/**
*
* Start the Fractal component {@link FractalHelper#SYSTEMRECOVERY_COMPONENT}.
*
*
* @throws PetalsCompositeCtrlException
* An error occurs stopping the system recovery service
*/
public void startSystemRecoveryService() throws PetalsCompositeCtrlException;
/**
* The configuration of the Petals container has changed and must be taken into account.
*
* @throws PetalsCompositeCtrlException
*/
public void onConfigurationUpdated() throws PetalsCompositeCtrlException;
/**
* Replace the configured shared area implementation ({@link FractalHelper#SHARED_AREA_COMPONENT}) in the
* {@link FractalHelper#SHARED_AREA_COMPOSITE}.
*/
public void replaceSharedArea(final Class> sharedAreaImplDef) throws PetalsCompositeCtrlException;
}