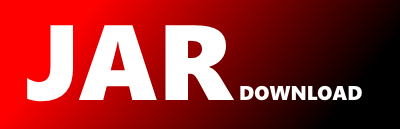
org.ow2.petals.microkernel.server.PetalsCompositeControllerImpl Maven / Gradle / Ivy
/**
* Copyright (c) 2013-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.microkernel.server;
import java.util.Properties;
import java.util.logging.Logger;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Interface;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.api.control.IllegalBindingException;
import org.objectweb.fractal.api.control.IllegalContentException;
import org.objectweb.fractal.api.control.IllegalLifeCycleException;
import org.objectweb.fractal.api.factory.InstantiationException;
import org.objectweb.fractal.fraclet.annotations.Lifecycle;
import org.objectweb.fractal.fraclet.annotations.Requires;
import org.objectweb.fractal.fraclet.extensions.Controller;
import org.objectweb.fractal.fraclet.types.Step;
import org.objectweb.fractal.util.Fractal;
import org.ow2.petals.basisapi.exception.PetalsException;
import org.ow2.petals.microkernel.api.communication.sharedarea.SharedAreaService;
import org.ow2.petals.microkernel.api.configuration.ConfigurationService;
import org.ow2.petals.microkernel.api.implementation.exception.MutableImplException;
import org.ow2.petals.microkernel.api.server.Binding;
import org.ow2.petals.microkernel.api.server.FractalHelper;
import org.ow2.petals.microkernel.container.ContainerController;
import org.ow2.petals.microkernel.container.exception.ContainerCtrlException;
import org.ow2.petals.microkernel.extension.PreExtensionsManager;
import org.ow2.petals.microkernel.server.exception.FractalComponentNotFoundException;
import org.ow2.petals.microkernel.server.exception.PetalsCompositeCtrlException;
import com.ebmwebsourcing.easycommons.log.LoggingUtil;
/**
* The controller implementation of the Fractal composite 'Petals'
*
* @author Christophe DENEUX - Linagora
*
*/
@org.objectweb.fractal.fraclet.annotations.Component(provides = @org.objectweb.fractal.fraclet.annotations.Interface(name = PetalsCompositeController.FRACTAL_SRV_ITF_NAME, signature = PetalsCompositeController.class))
public class PetalsCompositeControllerImpl implements PetalsCompositeController {
/**
* The configuration service
*/
@Requires(name = "configuration")
private ConfigurationService configurationService;
/**
* The pre-extension manager service
*/
@Requires(name = "preExtensionsMgr")
private PreExtensionsManager preExtensionsMgr;
@Controller
private Component comp;
/**
* The content controller of the composite 'Petals'
*/
private ContentController petalsCompositeCC;
private final LoggingUtil log = new LoggingUtil(Logger.getLogger(PetalsCompositeController.COMPONENT_LOGGER_NAME));
/**
*
* Starts the composite controller.
*
*
* @throws PetalsCompositeCtrlException
* An error occurs during the instantiation of a composite or a component.
*/
@Lifecycle(step = Step.START)
public void start() throws PetalsCompositeCtrlException {
this.log.call();
try {
this.petalsCompositeCC = Fractal.getContentController(FractalHelper.getParentComponent(this.comp));
} catch (final NoSuchInterfaceException | PetalsException e) {
throw new PetalsCompositeCtrlException(e);
}
}
/**
* Stops the registry manager.
*/
@Lifecycle(step = Step.STOP)
public void stop() {
this.log.call();
}
@Override
public void replaceSharedArea(final Class> sharedAreaImplDef) throws PetalsCompositeCtrlException {
try {
final Component composite = FractalHelper.getRecursiveComponentByName(this.petalsCompositeCC,
FractalHelper.SHARED_AREA_COMPOSITE);
final BindingController compositeBC = Fractal.getBindingController(composite);
final ContentController compositeCC = Fractal.getContentController(composite);
final Component oldSharedAreaComp = FractalHelper.getComponentByName(compositeCC,
FractalHelper.SHARED_AREA_COMPONENT);
// Remove the current implementation of the registry. Next it will be replaced by the expected one.
// no need to fail if the binding is not there (but if the interface is missing, there is a problem!
if (compositeBC.lookupFc(SHARED_AREA_FRACTAL_INT_SRV_ITF_NAME) != null) {
compositeBC.unbindFc(SHARED_AREA_FRACTAL_INT_SRV_ITF_NAME);
}
final BindingController oldSharedAreaImplBC = Fractal.getBindingController(oldSharedAreaComp);
// no need to fail if the binding is not there
try {
if (oldSharedAreaImplBC.lookupFc(SharedAreaService.CONFIGURATION_FRACTAL_ITF_NAME) != null) {
oldSharedAreaImplBC.unbindFc(SharedAreaService.CONFIGURATION_FRACTAL_ITF_NAME);
}
} catch (final NoSuchInterfaceException e) {
// no need to fail if the interface itself is not there
}
// no need to fail if the component is not there…
if (oldSharedAreaComp != null) {
compositeCC.removeFcSubComponent(oldSharedAreaComp);
}
// Instantiates the shared area implementation as a Fractal component
final Component sharedAreaImpl = FractalHelper.createPrimitiveComponent(sharedAreaImplDef);
this.log.debug("Shared area: Fractal component created.");
FractalHelper.addComponent(sharedAreaImpl, composite, FractalHelper.SHARED_AREA_COMPONENT,
new Binding(SharedAreaService.CONFIGURATION_FRACTAL_ITF_NAME,
(Interface) compositeCC.getFcInternalInterface(CONFIGURATION_FRACTAL_INT_ITF_NAME)));
// We bind the server interface 'service' of the component 'SharedAreaServiceImpl' with the server interface
// 'service' of the composite 'SharedArea'
compositeBC.bindFc(SHARED_AREA_FRACTAL_INT_SRV_ITF_NAME,
sharedAreaImpl.getFcInterface(SharedAreaService.FRACTAL_SRV_ITF_NAME));
this.log.debug("Shared area: interfaces bound.");
} catch (final NoSuchInterfaceException | IllegalLifeCycleException | IllegalBindingException
| IllegalContentException | InstantiationException e) {
throw new PetalsCompositeCtrlException(e);
}
}
@Override
public void stopJbiArtefacts() throws PetalsCompositeCtrlException {
this.log.start();
try {
final Component containerCtrlComponent = FractalHelper.getRecursiveComponentByName(this.petalsCompositeCC,
FractalHelper.CONTAINER_CONTROLLER_COMPONENT);
if (containerCtrlComponent != null) {
final ContainerController containerController = (ContainerController) containerCtrlComponent
.getFcInterface(ContainerController.FRACTAL_SRV_ITF_NAME);
containerController.stopAllJbiArtefacts();
} else {
throw new FractalComponentNotFoundException(FractalHelper.CONTAINER_CONTROLLER_COMPONENT);
}
} catch (final NoSuchInterfaceException | ContainerCtrlException e) {
throw new PetalsCompositeCtrlException(e);
} finally {
this.log.end();
}
}
@Override
public AutoCloseable pauseEndpointDirectory() throws PetalsCompositeCtrlException {
return this.pauseComponent(FractalHelper.ENDPOINT_DIRECTORY_COMPONENT);
}
private final void stopComponent(final String componentName) throws PetalsCompositeCtrlException {
this.log.start();
try {
final Component component = FractalHelper.getRecursiveComponentByName(this.petalsCompositeCC,
componentName);
FractalHelper.stopComponent(component, this.log);
} catch (final IllegalLifeCycleException e) {
throw new PetalsCompositeCtrlException(
String.format("An error occurs stopping the component '%s'.", componentName), e);
} finally {
this.log.end();
}
}
private AutoCloseable pauseComponent(final String componentName) throws PetalsCompositeCtrlException {
this.stopComponent(componentName);
return new AutoCloseable() {
private boolean closed = false;
@Override
public void close() throws Exception {
if (!closed) {
closed = true;
startComponent(componentName);
}
}
};
}
/**
* Compose as safely as possible two {@link AutoCloseable}.
*/
private static AutoCloseable compose(final AutoCloseable c1, final AutoCloseable c2) {
return new AutoCloseable() {
private boolean closed;
@Override
public void close() throws Exception {
if (!closed) {
closed = true;
Exception ex = null;
try {
c2.close();
} catch (final Exception e) {
ex = e;
} finally {
try {
c1.close();
} catch (final Exception e) {
if (ex != null) {
ex.addSuppressed(e);
} else {
ex = e;
}
}
}
if (ex != null) {
throw ex;
}
}
}
};
}
private final void startComponent(final String componentName) throws PetalsCompositeCtrlException {
this.log.start();
try {
final Component composite = FractalHelper.getRecursiveComponentByName(this.petalsCompositeCC,
componentName);
FractalHelper.startComponent(composite, this.log);
} catch (final IllegalLifeCycleException e) {
throw new PetalsCompositeCtrlException(String.format("An error occurs starting the component '%s'.",
componentName), e);
} finally {
this.log.end();
}
}
@SuppressWarnings("resource")
@Override
public AutoCloseable pauseMessagingSubsystem() throws PetalsCompositeCtrlException {
this.log.start();
final AutoCloseable c1 = this.pauseComponent(FractalHelper.LOCAL_TRANSPORTER_COMPONENT);
try {
final AutoCloseable c2 = this.pauseComponent(FractalHelper.TCP_TRANSPORTER_COMPONENT);
return compose(c1, c2);
} catch (final Exception e) {
// if the second fails, let's not forget to close the first before failing the method
try {
c1.close();
} catch (final Exception e2) {
e.addSuppressed(e2);
}
throw e;
}
}
@Override
public AutoCloseable pauseSharedArea() throws PetalsCompositeCtrlException {
return this.pauseComponent(FractalHelper.SHARED_AREA_COMPOSITE);
}
@Override
public Properties getSharedAreaCltConfiguration(final String providedTopologyPassphrase)
throws PetalsCompositeCtrlException {
try {
final Component sharedAreaComponent = FractalHelper.getRecursiveComponentByName(this.petalsCompositeCC,
FractalHelper.SHARED_AREA_COMPOSITE);
if (sharedAreaComponent != null) {
final SharedAreaService sharedArea = (SharedAreaService) sharedAreaComponent
.getFcInterface(SharedAreaService.FRACTAL_SRV_ITF_NAME);
return sharedArea.getConfiguration(providedTopologyPassphrase);
} else {
throw new FractalComponentNotFoundException(FractalHelper.SHARED_AREA_COMPOSITE);
}
} catch (final NoSuchInterfaceException e) {
throw new PetalsCompositeCtrlException(
"An error occurs getting the configuration of the shared area mutable implementation", e);
} catch (final MutableImplException e) {
throw new PetalsCompositeCtrlException(
"An error occurs getting the configuration of the shared area mutable implementation", e);
}
}
@Override
public void stopSystemRecoveryService() throws PetalsCompositeCtrlException {
this.stopComponent(FractalHelper.SYSTEMRECOVERY_COMPONENT);
}
@Override
public void startSystemRecoveryService() throws PetalsCompositeCtrlException {
this.startComponent(FractalHelper.SYSTEMRECOVERY_COMPONENT);
}
@Override
public void onConfigurationUpdated() throws PetalsCompositeCtrlException {
this.preExtensionsMgr.onConfigurationUpdated();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy