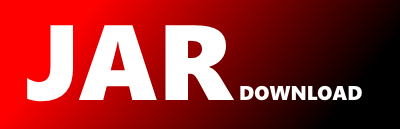
org.ow2.petals.probes.api.key.StringArrayProbeKey Maven / Gradle / Ivy
/**
* Copyright (c) 2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.probes.api.key;
import java.util.Arrays;
/**
* A {@link ProbeKey} implementation using a {@link String} array internally and taking care of common concerns such as
* optimisation, toString or equals/hashCode.
*
* @author Victor Noel
*
*/
public class StringArrayProbeKey implements ProbeKey {
/**
* For optimization reason, the string representation of the ServiceClientKey is computed at constructor level as it
* is immutable, and not each time it is needed.
*/
private final String[] keyAsStringArray;
public StringArrayProbeKey(String... keys) {
assert keys.length > 0;
keyAsStringArray = keys.clone();
}
@Override
public String[] toStringArray() {
return keyAsStringArray.clone();
}
@Override
public String toReadableString() {
if (keyAsStringArray.length == 1) {
return keyAsStringArray[0];
} else {
return Arrays.toString(keyAsStringArray);
}
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + Arrays.hashCode(keyAsStringArray);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
StringArrayProbeKey other = (StringArrayProbeKey) obj;
if (!Arrays.equals(keyAsStringArray, other.keyAsStringArray))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy