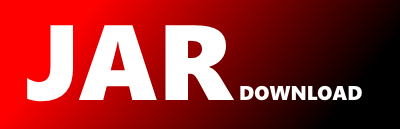
org.ow2.petals.registry_overlay.api.DataModelApi Maven / Gradle / Ivy
/**
* Copyright (c) 2013-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.registry_overlay.api;
import java.util.Map;
import org.ow2.petals.topology.generated.Topology;
import com.hazelcast.core.HazelcastInstance;
import com.hazelcast.core.ILock;
import com.hazelcast.core.IMap;
/**
* The data model of the registry overlay as distributed map, distributed multi map, distributed lock, ...
*
* @author Christophe DENEUX - Linagora
*
*/
public class DataModelApi {
/**
* Distributed map name containing the Petals ESB containers as HZL clients
*/
public static final String CLIENTS_MAP_NAME = "Petals ESB containers";
/**
* Pattern of the clients map lock name
*/
public static final String CLIENTS_LOCK_NAME_PATTERN = "Petals ESB containers lock";
/**
* Pattern of the map name containing the topologies.
*/
public static final String TOPOLOGIES_MAP_NAME_PATTERN = "Topologies";
/**
* Pattern of the topology lock name
*/
public static final String TOPOLOGY_LOCK_NAME_PATTERN = "%s: Topologies lock";
/**
* Pattern of the map name containing the endpoints
*/
public static final String ENDPOINT_MAP_NAME_PATTERN = "%s: Endpoints";
/**
* Pattern of the map name containing the endpoint descriptions
*/
public static final String ENDPOINT_DESCRIPTION_MAP_NAME_PATTERN = "%s: Endpoint descriptions";
private DataModelApi() {
// Utility class, no constructor
}
/**
* Get the name of distributed map containing the registry name of each Petals ESB container.
*
* @return The distributed map name
*/
public static String getClientsMapName() {
return CLIENTS_MAP_NAME;
}
/**
* Get the name of distributed lock about the clients map
*
* @return The distributed lock
*/
public static String getClientsMapLockName() {
return String.format(CLIENTS_LOCK_NAME_PATTERN);
}
/**
* Get the name of distributed map containing topologies.
*
* @return The distributed map name
*/
public static String getTopologiesMapName() {
return String.format(TOPOLOGIES_MAP_NAME_PATTERN);
}
/**
* Get the name of distributed lock about the topology map
*
* @return The distributed lock
*/
public static String getTopologiesMapLockName(final String registryName) {
return String.format(TOPOLOGY_LOCK_NAME_PATTERN, registryName);
}
/**
* Get the name of distributed map containing endpoints.
*
* @param registryName
* Registry name of the current container
* @return The distributed map name
*/
public static String getEndpointsMapName(final String registryName) {
return String.format(ENDPOINT_MAP_NAME_PATTERN, registryName);
}
/**
* Get the name of distributed map containing endpoint descriptions.
*
* @param registryName
* Registry name of the current container
* @return The distributed map name
*/
public static String getEndpointDescriptionsMapName(final String registryName) {
return String.format(ENDPOINT_DESCRIPTION_MAP_NAME_PATTERN, registryName);
}
/**
*
* Get the distributed map containing the registry name of each Petals ESB container.
*
*
* Caution : This map MUST be embraced by the lock returned by {@link #getClientsMapLock(HazelcastInstance)}
* to prevent concurrent modification.
*
*
* @param hzlInst
* The current HZL instance
* @return The distributed map
*/
public static IMap getClientsMap(final HazelcastInstance hzlInst) {
return hzlInst.getMap(DataModelApi.getClientsMapName());
}
/**
* Get the distributed lock about topology map
*
* @param hzlInst
* The current HZL instance
* @param registryName
* Registry name of the current container
* @return The distributed map
*/
public static ILock getClientsMapLock(final HazelcastInstance hzlInst) {
return hzlInst.getLock(DataModelApi.getClientsMapLockName());
}
/**
*
* Get the distributed map containing topologies.
*
*
* Caution : This map MUST be embraced by the lock returned by
* {@link #getTopologyLock(HazelcastInstance, String)} to prevent concurrent modification.
*
*
* @param hzlInst
* The current HZL instance
* @return The distributed map
*/
public static IMap getTopologiesMap(final HazelcastInstance hzlInst) {
return hzlInst.getMap(DataModelApi.getTopologiesMapName());
}
/**
* Get the distributed lock about topology map
*
* @param hzlInst
* The current HZL instance
* @return The distributed map
*/
public static ILock getTopologiesMapLock(final HazelcastInstance hzlInst, final String registryName) {
return hzlInst.getLock(DataModelApi.getTopologiesMapLockName(registryName));
}
/**
* Get the distributed map containing endpoints
*
* @param hzlInst
* The current HZL instance
* @param registryName
* Registry name of the current container
* @return The distributed map
*/
public static IMap getEndpointsMap(final HazelcastInstance hzlInst,
final String registryName) {
return hzlInst.getMap(DataModelApi.getEndpointsMapName(registryName));
}
/**
* Get the distributed map containing endpoint descriptions
*
* @param hzlInst
* The current HZL instance
* @param registryName
* Registry name of the current container
* @return The distributed map
*/
public static IMap getEndpointDescriptionsMap(final HazelcastInstance hzlInst,
final String registryName) {
return hzlInst.getMap(DataModelApi.getEndpointDescriptionsMapName(registryName));
}
/**
* Clean all information of a given topology
*
* @param hzlInst
* The current HZL instance
* @param registryName
* Registry name associated to the topology to clean
* @throws UnexistingTopologyException
* The given topology does not exist
* @return true
if it succeeded or false
if the topology didn't exist
*/
public static boolean cleanTopology(final HazelcastInstance hzlInst, final String registryName) {
final Map topologyMap = DataModelApi.getTopologiesMap(hzlInst);
if (topologyMap.containsKey(registryName)) {
DataModelApi.getEndpointsMap(hzlInst, registryName).clear();
DataModelApi.getEndpointDescriptionsMap(hzlInst, registryName).clear();
topologyMap.remove(registryName);
return true;
} else {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy