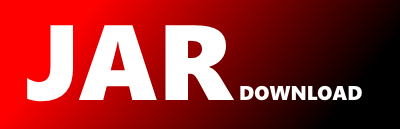
org.ow2.petals.engine.sampleclient.SampleClient Maven / Gradle / Ivy
/**
* Copyright (c) 2005-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.engine.sampleclient;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.security.Principal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.StringTokenizer;
import java.util.logging.Logger;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.jbi.JBIException;
import javax.jbi.component.Component;
import javax.jbi.component.ComponentContext;
import javax.jbi.component.ComponentLifeCycle;
import javax.jbi.component.ServiceUnitManager;
import javax.jbi.messaging.DeliveryChannel;
import javax.jbi.messaging.InOnly;
import javax.jbi.messaging.InOptionalOut;
import javax.jbi.messaging.InOut;
import javax.jbi.messaging.MessageExchange;
import javax.jbi.messaging.MessagingException;
import javax.jbi.messaging.NormalizedMessage;
import javax.jbi.messaging.RobustInOnly;
import javax.jbi.servicedesc.ServiceEndpoint;
import javax.management.ObjectName;
import javax.security.auth.Subject;
import javax.xml.namespace.QName;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.Source;
import org.ow2.petals.commons.log.FlowAttributes;
import org.ow2.petals.commons.log.FlowAttributesExchangeHelper;
import org.ow2.petals.commons.log.Level;
import org.ow2.petals.commons.log.PetalsExecutionContext;
import org.ow2.petals.component.framework.logger.ConsumeExtFlowStepBeginLogData;
import org.ow2.petals.component.framework.logger.StepLogHelper;
import org.ow2.petals.engine.sampleclient.gui.Console;
import org.ow2.petals.jaas.GroupPrincipal;
import org.ow2.petals.jaas.UserPrincipal;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentFragment;
import org.w3c.dom.Element;
import org.xml.sax.InputSource;
import com.ebmwebsourcing.easycommons.xml.JVMDocumentBuilders;
import com.ebmwebsourcing.easycommons.xml.XMLHelper;
/**
* Main class of the Sample Client component.
*
* This client is used to manage a container and to test it.
*
*
* @author Adrien Louis - EBM WebSourcing
* @author ddesjardins - EBM WebSourcing
* @author Marc Dutoo - Open Wide
*/
public class SampleClient implements Component, ComponentLifeCycle {
private static final QName WSA_TO = new QName("http://www.w3.org/2005/08/addressing", "To", "wsa");
private ComponentContext context;
private DeliveryChannel channel;
@SuppressWarnings("unused")
private ServiceEndpoint endpointReference;
private SampleClientListener listener;
private Console console;
private Logger logger;
/*
* (non-Javadoc)
* @see javax.jbi.component.ComponentLifeCycle#init(javax.jbi.component.ComponentContext)
*/
public void init(ComponentContext context) throws JBIException {
this.context = context;
this.logger = context.getLogger("", null);
console = new Console(this);
this.channel = this.context.getDeliveryChannel();
this.listener = new SampleClientListener(this.channel, console,
logger);
logger.log(Level.INFO, "init");
}
/*
* (non-Javadoc)
* @see javax.jbi.component.ComponentLifeCycle#start()
*/
public void start() throws JBIException {
logger.log(Level.INFO, "start");
Thread listenerThread = new Thread(this.listener, context
.getComponentName()
+ "-JBI listener thread");
listenerThread.start();
console.setVisible(true);
}
/*
* (non-Javadoc)
* @see javax.jbi.component.ComponentLifeCycle#stop()
*/
public void stop() throws JBIException {
logger.log(Level.INFO, "stop");
this.listener.stopProcessing();
console.setVisible(false);
}
/*
* (non-Javadoc)
* @see javax.jbi.component.ComponentLifeCycle#shutDown()
*/
public void shutDown() throws JBIException {
logger.log(Level.INFO, "shutDown");
this.listener.stopProcessing();
console= null;
channel.close();
channel = null;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.Component#getLifeCycle()
*/
public ComponentLifeCycle getLifeCycle() {
return this;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.Component#getServiceDescription(javax.jbi.servicedesc.ServiceEndpoint)
*/
public Document getServiceDescription(ServiceEndpoint arg0) {
return null;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.Component#getServiceUnitManager()
*/
public ServiceUnitManager getServiceUnitManager() {
return null;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.Component#isExchangeWithConsumerOkay(javax.jbi.servicedesc.ServiceEndpoint, javax.jbi.messaging.MessageExchange)
*/
public boolean isExchangeWithConsumerOkay(ServiceEndpoint arg0,
MessageExchange arg1) {
return false;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.Component#isExchangeWithProviderOkay(javax.jbi.servicedesc.ServiceEndpoint, javax.jbi.messaging.MessageExchange)
*/
public boolean isExchangeWithProviderOkay(ServiceEndpoint arg0,
MessageExchange arg1) {
logger.log(Level.INFO, "SampleClient accept the exchange");
return true;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.Component#resolveEndpointReference(org.w3c.dom.DocumentFragment)
*/
public ServiceEndpoint resolveEndpointReference(DocumentFragment arg0) {
return null;
}
/*
* (non-Javadoc)
* @see javax.jbi.component.ComponentLifeCycle#getExtensionMBeanName()
*/
public ObjectName getExtensionMBeanName() {
return null;
}
public static final String INONLY = "InOnly";
public static final String INOUT = "InOut";
public static final String INOPTIONALOUT = "InOptionalOut";
public static final String ROBUSTINONLY = "RobustInOnly";
/**
* Sends a message through Petals JBI
*
* @param itf
* the target JBI interface, optional if endpoint specified
* @param service
* the target JBI service
* @param op
* @param content
* the message content
* @param type
* @param attachmentFiles
* the message's attached files (if any)
* @param properties
* the message's properties
* @param syncTime
*/
public void send(String itf, String service, String op, String endpoint,
String content, String type, List attachmentFiles,
String properties, long syncTime) {
logger.log(Level.INFO, "SampleClient try to send");
final FlowAttributes flowAttributes = PetalsExecutionContext.initFlowAttributes();
logger.log(
Level.MONIT,
"",
new ConsumeExtFlowStepBeginLogData(flowAttributes.getFlowInstanceId(), flowAttributes.getFlowStepId()));
try {
Source source = Utils.createSource(content);
final MessageExchange msg;
switch (type) {
case INONLY: {
msg = channel.createExchangeFactory().createInOnlyExchange();
break;
}
case INOUT: {
msg = channel.createExchangeFactory().createInOutExchange();
break;
}
case INOPTIONALOUT: {
msg = channel.createExchangeFactory().createInOptionalOutExchange();
break;
}
case ROBUSTINONLY: {
msg = channel.createExchangeFactory().createRobustInOnlyExchange();
break;
}
default: {
throw new RuntimeException("impossible case");
}
}
FlowAttributesExchangeHelper.setFlowAttributes(msg, flowAttributes);
final NormalizedMessage nm = msg.createMessage();
// setting content
nm.setContent(source);
Map map = null;
if (properties != null) {
map = new HashMap();
StringTokenizer tk = new StringTokenizer(properties, ";");
while (tk.hasMoreTokens()) {
StringTokenizer tk2 = new StringTokenizer(tk.nextToken(),
"=");
String key = tk2.nextToken().trim();
String value = tk2.nextToken().trim();
msg.setProperty(key, value);
nm.setProperty(key, value);
map.put(key, value);
}
}
// get the security properties
if (map != null) {
// get security data
String user = map.get("sec.user");
String group = map.get("sec.group");
if (user != null) {
Subject subject = new Subject();
Set principals = subject.getPrincipals();
UserPrincipal up = new UserPrincipal(user);
principals.add(up);
GroupPrincipal gp = null;
if (group != null) {
gp = new GroupPrincipal(group);
} else {
gp = GroupPrincipal.ALL;
}
principals.add(gp);
nm.setSecuritySubject(subject);
}
// WS-Addressing support
String wsaTo = map.get("wsa.to");
Map headers = new HashMap(1);
if (wsaTo != null) {
// create the document fragment for wsa handling...
// TODO : Use CDK...
DocumentFragment frag = createWSAToDocFrag(WSA_TO, wsaTo);
headers.put(WSA_TO.toString(), frag);
}
// set the map in the message
nm.setProperty("javax.jbi.messaging.protocol.headers", headers);
}
// setting attachments if any
if (attachmentFiles != null) {
for (File attachmentFile : attachmentFiles) {
try {
nm.addAttachment(attachmentFile.getName(),
new DataHandler(new FileDataSource(
attachmentFile)));
} catch (MessagingException e) {
throw new Exception("Error when attaching file "
+ attachmentFile.getName());
}
}
}
// Set Endpoint value on the exchange
if (!Utils.isNullOrEmpty(endpoint)) {
// test if the endpoint is activated
ServiceEndpoint svcEndpoint = context.getEndpoint(QName.valueOf(service), endpoint);
if (svcEndpoint == null) {
throw new Exception("The endpoint '" + endpoint + "' for service '" + service
+ "' is not registered.");
} else {
msg.setEndpoint(svcEndpoint);
}
msg.setEndpoint(svcEndpoint);
}
// Set Service on the exchange
if (!Utils.isNullOrEmpty(service)) {
msg.setService(QName.valueOf(service));
}
// Set Interface on the exchange
if (!Utils.isNullOrEmpty(itf)) {
msg.setInterfaceName(QName.valueOf(itf));
}
// set the operation
msg.setOperation(QName.valueOf(op));
if (type.equals(INONLY))
((InOnly) msg).setInMessage(nm);
else if (type.equals(INOUT))
((InOut) msg).setInMessage(nm);
else if (type.equals(INOPTIONALOUT))
((InOptionalOut) msg).setInMessage(nm);
else if (type.equals(ROBUSTINONLY))
((RobustInOnly) msg).setInMessage(nm);
if (syncTime < 0) {
channel.send(msg);
} else {
boolean ok = channel.sendSync(msg, syncTime);
if (ok) {
listener.process(msg);
} else {
console.setResponse("Time out !");
StepLogHelper.addMonitExtFailureTrace(logger, flowAttributes, "Time out!", true);
}
}
} catch (Exception e) {
StepLogHelper.addMonitExtFailureTrace(logger, flowAttributes, e, true);
logger.log(Level.SEVERE, e.getMessage());
console.setResponse(e.getMessage());
console.showError(e);
}
}
/**
*
* @return
*/
public ServiceEndpoint[] getEndpoints() {
return context.getEndpoints(null);
}
/**
*
* @param text
* @return
*/
public ServiceEndpoint resolveDescription(String text) {
InputSource source = createSource(text);
DocumentFragment result = null;
try {
DocumentBuilderFactory factory = DocumentBuilderFactory
.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(source);
result = document.createDocumentFragment();
} catch (Exception e) {
e.printStackTrace();
}
return context.resolveEndpointReference(result);
}
/**
* Get the service description
*
* @param se
* @return
*/
public String getDescription(ServiceEndpoint se) {
Document doc;
try {
doc = context.getEndpointDescriptor(se);
if(doc == null) {
return null;
} else {
return XMLHelper.createStringFromDOMDocument(doc);
}
} catch (Throwable e) {
console.showError(e);
}
return null;
}
/**
* Get the service interfaces
*
* @param se
* @return
*/
public String getInterfaces(ServiceEndpoint se) {
String out = "";
try {
for (QName interfaces : se.getInterfaces()) {
out += interfaces;
}
return out;
} catch (Throwable e) {
console.showError(e);
}
return null;
}
/**
*
* @param se
* @return
*/
public String getAsReference(ServiceEndpoint se) {
try {
DocumentFragment doc = se.getAsReference(null);
if(doc == null) {
return null;
} else {
return XMLHelper.createStringFromDOMDocument(doc);
}
} catch (Throwable e) {
console.showError(e);
}
return null;
}
/**
* Create a source from a string
*
* @param msg
* @return
*/
protected InputSource createSource(String msg) {
InputSource source = new InputSource();
byte[] msgByte = msg.getBytes();
ByteArrayInputStream in = new ByteArrayInputStream(msgByte);
source.setByteStream(in);
return source;
}
/**
* Create the WSA:TO document fragment
*
* @param wsaToValue
* @return
*/
protected DocumentFragment createWSAToDocFrag(QName name, String wsaToValue) {
DocumentFragment result = null;
Document doc = JVMDocumentBuilders.newDocument();
Element elt = doc.createElementNS(name.getNamespaceURI(), name.getLocalPart());
elt.setPrefix(name.getPrefix());
result = doc.createDocumentFragment();
result.appendChild(doc.importNode(elt, true));
result.getFirstChild().setTextContent(wsaToValue);
result.normalize();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy