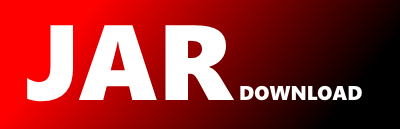
org.ow2.petals.engine.sampleclient.gui.Console Maven / Gradle / Ivy
/**
* Copyright (c) 2005-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.engine.sampleclient.gui;
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.GridLayout;
import java.awt.Menu;
import java.awt.MenuBar;
import java.awt.MenuItem;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ComponentEvent;
import java.awt.event.MouseAdapter;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.Enumeration;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import javax.jbi.servicedesc.ServiceEndpoint;
import javax.swing.AbstractButton;
import javax.swing.BorderFactory;
import javax.swing.ButtonGroup;
import javax.swing.DefaultComboBoxModel;
import javax.swing.DefaultListModel;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JScrollPane;
import javax.swing.JSeparator;
import javax.swing.JTabbedPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.ListModel;
import javax.swing.ListSelectionModel;
import javax.swing.WindowConstants;
import javax.swing.border.TitledBorder;
import javax.swing.filechooser.FileFilter;
import org.ow2.petals.engine.sampleclient.PropertiesRequestBeanRW;
import org.ow2.petals.engine.sampleclient.RequestBean;
import org.ow2.petals.engine.sampleclient.RequestBeanRW;
import org.ow2.petals.engine.sampleclient.SampleClient;
import org.ow2.petals.engine.sampleclient.gui.model.ServiceListRenderer;
/**
* Console of the SampleClient
*
* @author Adrien Louis - EBM WebSourcing
* @author ddesjardins - EBM WebSourcing
* @author Marc Dutoo - Open Wide
*/
public class Console extends javax.swing.JFrame {
/**
* @author Christophe HAMERLING - EBM WebSourcing
*/
private class ServiceSelector extends MouseAdapter {
private final String name;
/**
* Creates a new instance of ServiceSelector
*
* @param name
*/
public ServiceSelector(final String name) {
this.name = name;
}
/*
* (non-Javadoc)
*
* @see java.awt.event.MouseAdapter#mouseClicked(java.awt.event.MouseEvent)
*/
@Override
public void mouseClicked(final java.awt.event.MouseEvent e) {
Console.this.serviceNameField.setText(this.name);
Console.this.tabbedPane.setSelectedIndex(0);
}
/*
* (non-Javadoc)
*
* @see java.awt.event.MouseAdapter#mousePressed(java.awt.event.MouseEvent)
*/
@Override
public void mousePressed(final java.awt.event.MouseEvent e) {
Console.this.serviceNameField.setText(this.name);
Console.this.tabbedPane.setSelectedIndex(0);
}
}
/**
*
*/
private static final long serialVersionUID = -8117896325113377153L;
/**
* Lock the frame size
*
* @param frame
*/
private static void lockInMinSize(final JFrame frame) {
// Ensures user cannot resize frame to be smaller than frame is right
// now.
final int origX = frame.getSize().width;
final int origY = frame.getSize().height;
frame.addComponentListener(new java.awt.event.ComponentAdapter() {
/*
* (non-Javadoc)
*
* @see java.awt.event.ComponentAdapter#componentResized(java.awt.event.ComponentEvent)
*/
@Override
public void componentResized(final ComponentEvent event) {
final int X = (frame.getWidth() < origX) ? origX : frame.getWidth();
final int Y = (frame.getHeight() < origY) ? origY : frame.getHeight();
frame.setSize(X, Y);
}
});
}
private JButton asreference;
private JLabel background;
private JLabel background1;
private JPanel botQueryPanel;
private JTextField endpointNameField;
private JTextArea in;
private JRadioButton inout;
private JRadioButton inoutopt;
private JTextField interfaceNameField;
private JButton jButton1;
private JButton jButton2;
private JButton jButton3;
private JButton jButton31;
private JButton jButtonAddAttachments;
private JButton jButtonRemoveAttachments;
private JFileChooser jFileChooserAttachment;
private JLabel jLabelItf;
private JLabel jLabelSvc;
private JLabel jLabelEdp;
private JLabel jLabelOp;
private JLabel jLabel3;
private JLabel jLabel4;
private JLabel jLabel8;
private JLabel jLabel81;
private JList jList1;
private DefaultListModel jListModelSelectedAttachments;
private JList jListSelectedAttachments;
private JPanel jPanel1;
private JPanel jPanel2;
private JPanel jPanel3;
private JPanel jPanel4;
private JPanel jPanelAttachment;
private JRadioButton inonly;
private JScrollPane jScrollPane = null;
private JScrollPane jScrollPane1;
private JScrollPane jScrollPane2;
private JSeparator jSeparator1;
private JTabbedPane tabbedPane; // @jve:decl-index=0:visual-constraint="10,842"
private JTextArea jTextArea1;
private JPanel midQueryPanel;
private JTextField operation;
private JTextArea out;
private JLabel propJLabel = null;
private JTextArea props = null;
private JPanel queryPanel;
private JButton resolve;
private JRadioButton robustin;
private SampleClient sampleClient;
private JPanel sendPanel;
private JButton sendSync;
private JTextField serviceNameField;
private ServiceEndpoint[] services;
private JScrollPane servicesScrollPanel;
private JTextField timeoutField;
private JPanel topQueryPanel;
private ButtonGroup typeGroup;
/**
* Creates a new instance of Console
*/
public Console() {
super();
this.initialize();
this.initGUI();
}
/**
* Creates a new instance of Console
* @param sampleClient
*/
public Console(final SampleClient sampleClient) {
this();
this.sampleClient = sampleClient;
}
/**
*
*/
private void getAsReference() {
final ServiceEndpoint se = this.services[this.jList1.getSelectedIndex()];
final String msg = this.sampleClient.getAsReference(se);
this.openDialogBox("Reference of " + se, msg);
}
/**
* Retrieves the selected attachment files from the UI and returns them as a
* List
*
* @return
*/
private List getAttachmentFiles() {
final ArrayList attachmentFiles = new ArrayList();
final Enumeration> attachmentEnum = this.jListModelSelectedAttachments.elements();
while (attachmentEnum.hasMoreElements()) {
final Object attachment = attachmentEnum.nextElement();
if (attachment instanceof File) {
attachmentFiles.add((File) attachment);
} // else silent error
}
return attachmentFiles;
}
/**
* This method initializes jScrollPane
*
* @return javax.swing.JScrollPane
*/
private JScrollPane getJScrollPane() {
if (this.jScrollPane == null) {
this.jScrollPane = new JScrollPane();
this.jScrollPane.setBounds(new Rectangle(5, 281, 576, 64));
this.jScrollPane.setViewportView(this.getProps());
}
return this.jScrollPane;
}
/**
* This method initializes jTextArea
*
* @return javax.swing.JTextArea
*/
private JLabel getPropJLabel() {
if (this.propJLabel == null) {
this.propJLabel = new JLabel();
this.propJLabel.setBounds(new Rectangle(5, 258, 576, 13));
this.propJLabel.setText("Properties");
}
return this.propJLabel;
}
/**
* This method initializes props
*
* @return javax.swing.JTextArea
*/
private JTextArea getProps() {
if (this.props == null) {
this.props = new JTextArea();
}
return this.props;
}
/**
* Get all the services
*/
private void getServices() {
final ServiceEndpoint[] serviceEndpoints = this.sampleClient.getEndpoints();
this.services = serviceEndpoints;
final DefaultListModel listModel = new DefaultListModel();
this.jList1.setCellRenderer(new ServiceListRenderer(this.jList1.getCellRenderer()));
for (final ServiceEndpoint serviceEndpoint : serviceEndpoints) {
final String name = String.format("%s (%s) -> %s", serviceEndpoint.getServiceName().toString(),
serviceEndpoint.getInterfaces()[0].toString(), serviceEndpoint.getEndpointName());
final JLabel label = new JLabel();
label.setText(name);
label.setToolTipText("Click to select in the Send Panel");
label.addMouseListener(new ServiceSelector(name));
listModel.addElement(label);
}
this.jList1.addMouseListener(new java.awt.event.MouseAdapter() {
@Override
public void mouseClicked(final java.awt.event.MouseEvent e) {
if ((e.getClickCount() == 2) && (Console.this.jList1.getSelectedValue() != null)) {
final JLabel label = Console.this.jList1.getSelectedValue();
final String selectedValue = label.getText();
final String serviceName = selectedValue.substring(0, selectedValue.indexOf(' '));
final String interfaceName = selectedValue.substring(selectedValue.indexOf(" (") + 2,
selectedValue.indexOf(") ->"));
final String endpointName = selectedValue.substring(selectedValue.indexOf("->") + 3);
Console.this.interfaceNameField.setText(interfaceName);
Console.this.serviceNameField.setText(serviceName);
Console.this.endpointNameField.setText(endpointName);
Console.this.tabbedPane.setSelectedIndex(0);
}
}
});
this.jList1.setModel(listModel);
if (serviceEndpoints.length > 0) {
this.jList1.setSelectedIndex(0);
}
}
private MenuBar initMenu() {
final MenuBar menuBar = new MenuBar();
final Menu menu = new Menu("File");
MenuItem load = new MenuItem("Load request");
final JFileChooser choose = new JFileChooser();
choose.setDialogTitle("Select request file to load");
choose.setFileSelectionMode(JFileChooser.FILES_ONLY);
choose.setFileFilter(new FileFilter() {
@Override
public boolean accept(File f) {
return f.getName().endsWith("properties");
}
@Override
public String getDescription() {
// TODO Auto-generated method stub
return null;
}
});
load.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
final int res = choose.showOpenDialog(Console.this);
if (res == JFileChooser.APPROVE_OPTION) {
File f = choose.getSelectedFile();
RequestBeanRW reader = new PropertiesRequestBeanRW();
Properties props = new Properties();
try {
props.load(new FileInputStream(f));
RequestBean bean = reader.read(props);
updateConsole(bean);
} catch (FileNotFoundException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
});
menu.add(load);
MenuItem save = new MenuItem("Save request");
final JFileChooser chooseSave = new JFileChooser();
chooseSave.setDialogTitle("Select the folder to save request to...");
chooseSave.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
save.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
final int res = chooseSave.showOpenDialog(Console.this);
if (res == JFileChooser.APPROVE_OPTION) {
File folder = chooseSave.getSelectedFile();
Date d = new Date(System.currentTimeMillis());
SimpleDateFormat sdf = new SimpleDateFormat("ddMMyy-HHmmss");
File f = new File(folder, "petals-client-request-" + sdf.format(d) + ".properties");
RequestBeanRW writer = new PropertiesRequestBeanRW();
Properties props = new Properties();
writer.write(getRequestBean(), props);
try {
props.store(new FileOutputStream(f), "Stored on " + sdf.format(d));
} catch (FileNotFoundException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
});
menu.add(save);
menuBar.add(menu);
// help
Menu help = new Menu("?");
MenuItem about = new MenuItem("About");
about.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
help.add(about);
menuBar.add(help);
return menuBar;
}
/**
* Update the console entries with the request bean
*
* @param request
*/
private void updateConsole(RequestBean request) {
if (request.getMessage() != null)
in.setText(request.getMessage());
if (request.getInterfaceName() != null)
interfaceNameField.setText(request.getInterfaceName());
if (request.getServiceName() != null)
serviceNameField.setText(request.getServiceName());
if (request.getEndpointName() != null)
endpointNameField.setText(request.getEndpointName());
if (request.getTimeout() != null)
timeoutField.setText(request.getTimeout());
if (request.getProperties() != null)
props.setText(request.getProperties());
if (request.getOperation() != null)
operation.setText(request.getOperation());
if (request.getMep() != null) {
if (request.getMep().equalsIgnoreCase(SampleClient.INOUT)) {
inout.setSelected(true);
} else if (request.getMep().equalsIgnoreCase(SampleClient.INONLY)) {
inonly.setSelected(true);
} else if (request.getMep().equalsIgnoreCase(SampleClient.INOPTIONALOUT)) {
inoutopt.setSelected(true);
} else if (request.getMep().equalsIgnoreCase(SampleClient.ROBUSTINONLY)) {
robustin.setSelected(true);
}
}
}
private RequestBean getRequestBean() {
RequestBean bean = new RequestBean();
bean.setEndpointName(endpointNameField.getText());
bean.setInterfaceName(interfaceNameField.getText());
bean.setMep(getMep());
bean.setMessage(in.getText());
bean.setOperation(operation.getText());
bean.setProperties(props.getText());
bean.setServiceName(serviceNameField.getText());
bean.setTimeout(timeoutField.getText());
return bean;
}
/**
* Get the selected MEP
*
* @return
*/
private String getMep() {
String result = null;
JRadioButton selected = null;
final Enumeration rbs = this.typeGroup.getElements();
while (rbs.hasMoreElements()) {
final AbstractButton but = rbs.nextElement();
if (but.isSelected()) {
selected = (JRadioButton) but;
}
}
if (selected != null) {
result = selected.getText();
}
return result;
}
/**
* Initialize the GUI
*/
private void initGUI() {
try {
final MenuBar menuBar = initMenu();
this.setMenuBar(menuBar);
final GridLayout gridLayout1 = new GridLayout();
gridLayout1.setRows(2);
final GridLayout gridLayout = new GridLayout();
gridLayout.setRows(4);
this.setDefaultCloseOperation(WindowConstants.DO_NOTHING_ON_CLOSE);
{
this.tabbedPane = new JTabbedPane();
this.getContentPane().add(this.tabbedPane);
this.tabbedPane.setSize(592, 758);
// jTabbedPane1.setBackground(new Color(242, 237, 243));
{
this.sendPanel = new JPanel();
byte[] buffer = null;
InputStream inStream = this.getClass().getResourceAsStream("/ebmlogo.jpg");
try {
if (inStream == null) {
inStream = new FileInputStream("src" + File.separator + "img"
+ File.separator + "ebmlogo.jpg");
}
final DataInputStream dis = new DataInputStream(inStream);
buffer = new byte[dis.available()];
dis.readFully(buffer);
final ImageIcon img1 = new ImageIcon(buffer);
this.background = new JLabel(img1);
this.background.setBounds(360, 640, img1.getIconWidth(), img1
.getIconHeight());
this.sendPanel.add(this.background);
} catch (final Exception e) {
JOptionPane.showMessageDialog(this, e.getMessage(), "Error",
JOptionPane.ERROR_MESSAGE);
}
this.sendPanel.setLayout(null);
this.tabbedPane.addTab("Send", null, this.sendPanel, null);
this.sendPanel.setOpaque(false);
{
{
this.typeGroup = new ButtonGroup();
}
this.jPanel1 = new JPanel();
this.jPanel1.setLayout(gridLayout);
this.jPanel1.setBounds(new Rectangle(0, 0, 586, 90));
this.jPanel1.setBorder(BorderFactory.createTitledBorder("Service"));
this.jPanel1.setOpaque(false);
this.jLabelItf = new JLabel();
this.jLabelItf.setText("Interface");
this.interfaceNameField = new JTextField();
this.jLabelSvc = new JLabel();
this.jLabelSvc.setText("Service");
this.serviceNameField = new JTextField();
this.jLabelOp = new JLabel();
this.jLabelOp.setText("Operation");
this.jLabelEdp = new JLabel();
this.jLabelEdp.setText("Endpoint");
this.endpointNameField = new JTextField();
this.operation = new JTextField();
this.jPanel1.add(this.jLabelItf, null);
this.jPanel1.add(this.interfaceNameField, null);
this.jPanel1.add(this.jLabelSvc, null);
this.jPanel1.add(this.serviceNameField, null);
this.jPanel1.add(this.jLabelEdp, null);
this.jPanel1.add(this.endpointNameField, null);
this.jPanel1.add(this.jLabelOp, null);
this.jPanel1.add(this.operation, null);
}
{
// creating attachments panel
this.jPanelAttachment = new JPanel(new BorderLayout());
this.jPanelAttachment.setBounds(new Rectangle(0, 85, 586, 95));
this.jPanelAttachment.setBorder(BorderFactory
.createTitledBorder("Attachments"));
this.jPanelAttachment.setOpaque(false);
{
this.jListSelectedAttachments = new JList();
this.jListSelectedAttachments
.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
this.jListModelSelectedAttachments = new DefaultListModel();
this.jListSelectedAttachments
.setModel(this.jListModelSelectedAttachments);
}
{
// creating add attachment button
this.jButtonAddAttachments = new JButton();
this.jButtonAddAttachments.setText("Add attachments");
this.jButtonAddAttachments.setBounds(new Rectangle(5, 20, 196, 26));
this.jButtonAddAttachments.setPreferredSize(new java.awt.Dimension(130,
18));
this.jFileChooserAttachment = new JFileChooser();
this.jFileChooserAttachment.setMultiSelectionEnabled(true);
this.jButtonAddAttachments.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent e) {
Console.this.jFileChooserAttachment
.setDialogTitle("Select files to attach");
final int res = Console.this.jFileChooserAttachment
.showOpenDialog(Console.this);
if (res == JFileChooser.APPROVE_OPTION) {
for (final File attachmentFile : Console.this.jFileChooserAttachment
.getSelectedFiles()) {
Console.this.jListModelSelectedAttachments
.addElement(attachmentFile);
}
}
}
});
}
{
// creating remove attachment button
this.jButtonRemoveAttachments = new JButton();
this.jButtonRemoveAttachments.setText("Remove selected");
this.jButtonRemoveAttachments.setBounds(new Rectangle(5, 20, 196, 26));
this.jButtonRemoveAttachments.setPreferredSize(new java.awt.Dimension(
130, 18));
this.jButtonRemoveAttachments.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent e) {
for (final Object attachment : Console.this.jListSelectedAttachments
.getSelectedValues()) {
Console.this.jListModelSelectedAttachments
.removeElement(attachment);
}
}
});
}
{
final JPanel jPanelAttachmentButtons = new JPanel(new GridLayout(1, 2));
jPanelAttachmentButtons.add(this.jButtonAddAttachments, null);
jPanelAttachmentButtons.add(this.jButtonRemoveAttachments, null);
this.jPanelAttachment.add(
new JScrollPane(this.jListSelectedAttachments),
BorderLayout.CENTER);
this.jPanelAttachment.add(jPanelAttachmentButtons, BorderLayout.SOUTH);
}
}
{
this.typeGroup = new ButtonGroup();
}
{
this.jPanel3 = new JPanel();
this.jPanel3.setLayout(gridLayout1);
this.jPanel3.setLocation(new Point(0, 180));
this.jPanel3.setSize(586, 80);
this.jPanel3.setBorder(BorderFactory
.createTitledBorder("Message exchange pattern"));
this.jPanel3.setOpaque(false);
{
this.inonly = new JRadioButton();
this.inonly.setText(SampleClient.INONLY);
this.inonly.setAutoscrolls(true);
this.typeGroup.add(this.inonly);
this.inonly.setSelected(true);
this.inonly.setOpaque(false);
}
{
this.inout = new JRadioButton();
this.inout.setText(SampleClient.INOUT);
this.typeGroup.add(this.inout);
this.inout.setOpaque(false);
}
{
this.inoutopt = new JRadioButton();
this.jPanel3.add(this.inonly, null);
this.inoutopt.setText(SampleClient.INOPTIONALOUT);
this.typeGroup.add(this.inoutopt);
this.inoutopt.setOpaque(false);
}
{
this.robustin = new JRadioButton();
this.jPanel3.add(this.inout, null);
this.jPanel3.add(this.inoutopt, null);
this.jPanel3.add(this.robustin, null);
this.robustin.setText(SampleClient.ROBUSTINONLY);
this.robustin.setOpaque(false);
this.typeGroup.add(this.robustin);
}
}
{
this.jPanel2 = new JPanel();
this.jPanel2.setLayout(null);
this.jPanel2.setSize(586, 500);
this.jPanel2.setLocation(new Point(0, 260));
this.jPanel2.setPreferredSize(new java.awt.Dimension(10, 10));
this.jPanel2.setBorder(BorderFactory.createTitledBorder(null,
"Request / Response body", TitledBorder.LEADING, TitledBorder.TOP));
this.jPanel2.setOpaque(false);
{
this.jLabel3 = new JLabel();
this.jLabel3.setBounds(new Rectangle(5, 22, 576, 15));
this.jLabel3.setText("In");
}
{
this.jScrollPane1 = new JScrollPane();
this.jScrollPane1.setBounds(new Rectangle(5, 45, 576, 90));
this.jScrollPane1.setAutoscrolls(true);
this.jPanel2.add(this.jLabel3, null);
{
this.in = new JTextArea(3, 40);
this.in.setLineWrap(true);
this.in.setWrapStyleWord(true);
this.in.setText("hi ! ");
this.jScrollPane1.setViewportView(this.in);
}
}
this.jPanel2.add(this.jScrollPane1, null);
{
this.jLabel4 = new JLabel();
this.jLabel4.setBounds(new Rectangle(5, 142, 576, 13));
this.jLabel4.setText("Out");
}
this.jPanel2.add(this.jLabel4, null);
{
this.jScrollPane2 = new JScrollPane();
this.jScrollPane2.setBounds(new Rectangle(5, 163, 576, 90));
{
this.out = new JTextArea(3, 40);
this.out.setLineWrap(true);
this.out.setWrapStyleWord(true);
}
this.jScrollPane2.setViewportView(this.out);
}
this.jPanel2.add(this.jScrollPane2, null);
}
{
this.jPanel4 = new JPanel();
this.jPanel2.add(this.jPanel4, null);
this.jPanel2.add(this.getPropJLabel(), null);
this.jPanel2.add(this.getJScrollPane(), null);
this.sendPanel.add(this.jPanel1);
this.sendPanel.add(this.jPanelAttachment); // adding
// the
// attachments UI
this.sendPanel.add(this.jPanel3, null);
this.sendPanel.add(this.jPanel2, null);
this.jPanel4.setLayout(null);
this.jPanel4.setOpaque(false);
this.jPanel4.setBounds(new Rectangle(0, 350, 586, 120));
this.jPanel4.setBorder(BorderFactory.createTitledBorder("Send"));
{
this.sendSync = new JButton();
this.sendSync.setText("SendSync");
this.sendSync.setBounds(new Rectangle(5, 20, 196, 26));
this.sendSync.setPreferredSize(new java.awt.Dimension(130, 18));
this.sendSync.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.sendSync();
}
});
}
{
this.timeoutField = new JTextField();
this.timeoutField.setText("2000");
this.timeoutField.setToolTipText("timeout, in ms");
this.timeoutField.setBounds(new Rectangle(209, 23, 42, 23));
}
{
this.jButton1 = new JButton();
this.jPanel4.add(this.sendSync, null);
this.jPanel4.add(this.timeoutField, null);
this.jPanel4.add(this.jButton1, null);
this.jButton1.setText("Send - Accept");
this.jButton1.setBounds(new Rectangle(5, 55, 196, 27));
this.jButton1.setPreferredSize(new java.awt.Dimension(152, 15));
this.jButton1.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.send();
}
});
}
}
}
{
this.queryPanel = new JPanel();
this.queryPanel.setOpaque(false);
this.tabbedPane.addTab("Query", null, this.queryPanel, null);
{
byte[] buffer = null;
InputStream inStream = this.getClass().getResourceAsStream("/ebmlogo.jpg");
try {
if (inStream == null) {
inStream = new FileInputStream("src" + File.separator + "img"
+ File.separator + "ebmlogo.jpg");
}
final DataInputStream dis = new DataInputStream(inStream);
buffer = new byte[dis.available()];
dis.readFully(buffer);
final ImageIcon img1 = new ImageIcon(buffer);
this.background1 = new JLabel(img1);
} catch (final Exception e) {
JOptionPane.showMessageDialog(this, e.getMessage(), "Error",
JOptionPane.ERROR_MESSAGE);
}
{
this.topQueryPanel = new JPanel();
this.topQueryPanel.setLayout(null);
this.topQueryPanel.setBorder(BorderFactory
.createTitledBorder("Query the server to find endpoints"));
this.topQueryPanel.setOpaque(false);
{
this.jLabel8 = new JLabel();
this.jLabel8.setBounds(new Rectangle(5, 0, 241, 23));
this.jLabel8.setFont(new java.awt.Font("Tahoma", 0, 14));
}
{
this.jButton2 = new JButton();
this.jButton2.setText("Find all the endpoints");
this.jButton2.setBounds(new Rectangle(5, 30, 181, 25));
this.jButton2.setPreferredSize(new java.awt.Dimension(123, 23));
this.jButton2.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.getServices();
}
});
}
{
this.jSeparator1 = new JSeparator();
this.jSeparator1.setBounds(new Rectangle(5, 60, 576, 8));
}
{
this.resolve = new JButton();
this.resolve.setText("Resolve following description");
this.resolve.setActionCommand("Resolve following description");
this.resolve.setBounds(new Rectangle(5, 65, 241, 25));
this.resolve.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.resolveDescription();
}
});
}
{
this.jTextArea1 = new JTextArea(6, 20);
this.jTextArea1.setText("< enter a w3c document fragment>");
this.jTextArea1.setLineWrap(true);
this.jTextArea1.setWrapStyleWord(true);
this.jTextArea1.setBounds(new Rectangle(5, 95, 576, 71));
this.jTextArea1.setAutoscrolls(true);
this.jTextArea1.setBorder(BorderFactory.createEtchedBorder());
}
this.topQueryPanel.add(this.jLabel8, null);
this.topQueryPanel.add(this.jButton2, null);
this.topQueryPanel.add(this.jSeparator1, null);
this.topQueryPanel.add(this.resolve, null);
this.topQueryPanel.add(this.jTextArea1, null);
}
this.midQueryPanel = new JPanel();
this.midQueryPanel.setLayout(null);
this.midQueryPanel.setBorder(BorderFactory
.createTitledBorder("Result : List of endpoints"));
this.midQueryPanel.setOpaque(false);
final ListModel jList1Model = new DefaultComboBoxModel(new String[0]);
this.jList1 = new JList();
this.jList1.setModel(jList1Model);
this.servicesScrollPanel = new JScrollPane(this.jList1);
this.servicesScrollPanel.setBounds(new Rectangle(5, 50, 576, 300));
this.servicesScrollPanel.setAutoscrolls(true);
this.midQueryPanel.add(this.servicesScrollPanel, null);
}
{
this.botQueryPanel = new JPanel();
this.botQueryPanel.setLayout(null);
this.botQueryPanel.setBorder(BorderFactory
.createTitledBorder("Action on the selected endpoint"));
this.botQueryPanel.setOpaque(false);
{
this.jButton3 = new JButton();
this.jButton3.setText("Get service description");
this.jButton3.setBounds(new Rectangle(5, 20, 251, 25));
this.jButton3.setPreferredSize(new java.awt.Dimension(145, 26));
this.jButton3.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.showDescription();
}
});
this.jButton31 = new JButton();
this.jButton31.setBounds(new Rectangle(265, 20, 231, 25));
this.jButton31.setText("Get interfaces");
this.jButton31.setPreferredSize(new Dimension(145, 26));
this.jButton31.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.showInterfaces();
}
});
}
{
this.asreference = new JButton();
this.botQueryPanel.add(this.jButton3, null);
this.botQueryPanel.add(this.asreference, null);
this.botQueryPanel.add(this.jButton31, null);
this.asreference.setText("Get selected endpoint as reference");
this.asreference.setBounds(new Rectangle(5, 55, 251, 25));
this.asreference.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent evt) {
Console.this.topQueryPanel.add(Console.this.jLabel81, null);
Console.this.getAsReference();
}
});
if (this.background1 != null) {
this.background1.setBounds(new Rectangle(360, 63, this.background
.getWidth(), this.background.getHeight()));
this.botQueryPanel.add(this.background1, null);
}
}
}
// botQueryPanel.setBorder(new LineBorder(Color.BLACK, 2));
// test.setBorder(new LineBorder(Color.BLACK, 2));
// topQueryPanel.setBorder(new LineBorder(Color.BLACK, 2));
this.queryPanel.setLayout(new GridBagLayout());
final GridBagConstraints c = new GridBagConstraints();
c.fill = GridBagConstraints.BOTH;
c.gridx = 0;
c.gridy = 0;
c.gridwidth = 3;
c.ipady = 207;
c.weighty = 0.0;
c.weightx = 0.0;
this.queryPanel.add(this.topQueryPanel, c);
c.fill = GridBagConstraints.BOTH;
c.gridx = 0;
c.gridy = 1;
c.gridwidth = 3;
c.ipady = 0;
c.ipadx = 0;
c.weighty = 1.0;
c.weightx = 1.0;
this.queryPanel.add(this.midQueryPanel, c);
c.fill = GridBagConstraints.BOTH;
c.gridx = 0;
c.gridy = 3;
c.ipady = 130;
c.ipadx = 500;
c.gridwidth = 3;
c.weighty = 0.0;
c.weightx = 0.0;
this.queryPanel.add(this.botQueryPanel, c);
}
}
this.pack();
} catch (final Exception e) {
e.printStackTrace();
}
}
/**
* This method initializes this
*
*/
private void initialize() {
this.setLayout(new BorderLayout());
this.setTitle("Petals - Sample client");
this.setResizable(true);
this.setSize(600, 789);
lockInMinSize(this);
}
/**
* open a new JFrame with a textArea
*
* @param title
* @param textMsg
*/
private void openDialogBox(final String title, final String textMsg) {
final JFrame f = new JFrame(title);
final JTextArea text = new JTextArea(textMsg);
text.setLineWrap(true);
text.setWrapStyleWord(true);
final JScrollPane sp = new JScrollPane(text);
f.getRootPane().getContentPane().add(sp);
f.pack();
f.setSize(300, 300);
f.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
f.setVisible(true);
}
/**
*
*/
private void resolveDescription() {
final String fragmentDescription = this.jTextArea1.getText();
final ServiceEndpoint se = this.sampleClient.resolveDescription(fragmentDescription);
final DefaultListModel listModel = new DefaultListModel();
listModel.addElement(se);
this.jList1.setModel(listModel);
}
/**
* Save the attachments, show the save window.
*
* @return
*/
public String saveFolder() {
final JFileChooser saveFolder = new JFileChooser();
saveFolder.setDialogTitle("Select folder to write attachments");
saveFolder.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
final int res = saveFolder.showSaveDialog(this);
String result = ".";
if (res == JFileChooser.APPROVE_OPTION) {
result = saveFolder.getSelectedFile().getAbsolutePath();
}
return result;
}
/**
* Send a message
*
*/
private void send() {
JRadioButton selected = null;
this.out.setText("");
final Enumeration rbs = this.typeGroup.getElements();
while (rbs.hasMoreElements()) {
final AbstractButton but = rbs.nextElement();
if (but.isSelected()) {
selected = (JRadioButton) but;
break;
}
}
this.sampleClient.send(this.interfaceNameField.getText(), this.serviceNameField.getText(), this.operation.getText(),
this.endpointNameField.getText(), this.in.getText(),
selected != null ? selected.getText() : SampleClient.INONLY, this.getAttachmentFiles(),
this.props.getText(), -1);
}
/**
* Send a message
*/
private void sendSync() {
JRadioButton selected = null;
this.out.setText("");
final Enumeration rbs = this.typeGroup.getElements();
while (rbs.hasMoreElements()) {
final AbstractButton but = rbs.nextElement();
if (but.isSelected()) {
selected = (JRadioButton) but;
break;
}
}
this.sampleClient.send(this.interfaceNameField.getText(), this.serviceNameField.getText(), this.operation.getText(),
this.endpointNameField.getText(), this.in.getText(),
selected != null ? selected.getText() : SampleClient.INONLY, this.getAttachmentFiles(),
this.props.getText(), Long.parseLong(this.timeoutField.getText()));
}
/**
* Set the message response in the output area
*
* @param text
*/
public void setResponse(final String text) {
this.out.setText(text);
}
/**
* Set the message response and properties in their areas
*
* @param text
* @param properties
*/
public void setResponse(final String text, final Map properties) {
this.out.setText(text);
this.props.setText("");
for (final String name : properties.keySet()) {
this.props.append(name + "=" + properties.get(name) + ";");
}
}
/**
* Show the service description
*/
private void showDescription() {
final ServiceEndpoint se = this.services[this.jList1.getSelectedIndex()];
final String msg = this.sampleClient.getDescription(se);
this.openDialogBox("Description of " + se, msg);
}
/**
* Show the message error
*
* @param e
*/
public void showError(final Throwable e) {
final StringWriter sw = new StringWriter();
final PrintWriter psw = new PrintWriter(sw);
e.printStackTrace(psw);
JOptionPane.showMessageDialog(this, sw.toString(), "error", JOptionPane.ERROR_MESSAGE);
}
/**
* Show the interfaces
*/
private void showInterfaces() {
final ServiceEndpoint se = this.services[this.jList1.getSelectedIndex()];
final String msg = this.sampleClient.getInterfaces(se);
this.openDialogBox("Interfaces of " + se, msg);
}
/**
* Show a warning
*
* @param msg
*/
public void showWarning(final String msg) {
JOptionPane.showMessageDialog(this, msg, "warning", JOptionPane.WARNING_MESSAGE);
}
} // @jve:decl-index=0:visual-constraint="10,10"
© 2015 - 2025 Weber Informatics LLC | Privacy Policy