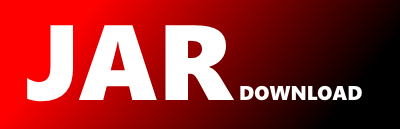
org.ow2.petals.se.jsr181.Jsr181SuManager Maven / Gradle / Ivy
/**
* Copyright (c) 2008-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.se.jsr181;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.axis2.context.ConfigurationContext;
import org.ow2.petals.component.framework.api.configuration.SuConfigurationParameters;
import org.ow2.petals.component.framework.api.exception.PEtALSCDKException;
import org.ow2.petals.component.framework.jbidescriptor.generated.Provides;
import org.ow2.petals.component.framework.se.AbstractServiceEngine;
import org.ow2.petals.component.framework.se.ServiceEngineServiceUnitManager;
import org.ow2.petals.component.framework.su.ServiceUnitDataHandler;
import org.ow2.petals.se.jsr181.axis.Axis2Deployer;
import org.ow2.petals.se.jsr181.model.JaxConfiguration;
import org.ow2.petals.se.jsr181.model.JaxConfigurationHandler;
/**
* The Service-Unit manager for the JSR-181 SE.
*
* @author Christophe HAMERLING - EBM WebSourcing
* @author Vincent Zurczak - EBM WebSourcing
*/
public class Jsr181SuManager extends ServiceEngineServiceUnitManager {
/**
* The name of the parameter defining the JAX-WS class name.
*/
private final static String CONFIG_CLASS = "class";
/**
* A map associating service-unit names and configuration handlers.
*/
private final Map> suNameToJaxConfigHandler =
new HashMap> ();
/**
* Constructor.
* @param component the service engine instance
*/
public Jsr181SuManager(final AbstractServiceEngine component) {
super( component );
}
@Override
protected void doDeploy(final ServiceUnitDataHandler suDH) throws PEtALSCDKException {
/*
* In case where we would like the generate the WSDL at deployment time,
* we should also generate the jbi.xml file. Both are too coupled.
*
* Here is the code to generate the WSDL from the JAX-WS class.
*
* // Get a JaxConfigurationHandler
* ByteArrayOutputStream bos = new ByteArrayOutputStream();
* jaxConfigurationHandler.getAxisService().printWSDL( bos );
* result = XMLUtil.createDocumentFromString( bos.toString());
*/
final List providesList = suDH.getDescriptor().getServices().getProvides();
if (providesList.size() == 0) {
throw new PEtALSCDKException( "This service-unit does not specify any service (no provides sections)." );
}
final List handlers = new ArrayList(providesList.size());
this.suNameToJaxConfigHandler.put(suDH.getName(), handlers);
final Axis2Deployer deployer = getComponent().getAxis2Deployer();
for( Provides provides : providesList ) {
final SuConfigurationParameters extensions = suDH.getConfigurationExtensions(provides);
String className = extensions.get( CONFIG_CLASS );
if( className != null )
className = className.trim();
if( className == null || className.length() == 0 )
throw new PEtALSCDKException( "The class name cannot be null or empty." );
try {
// Create a JBI URL, not used but required...
final URL serviceURL = new URL("http://localhost/services/" + provides.getServiceName().getLocalPart());
final String edptName = provides.getEndpointName();
// Register handlers that will be used at runtime
final JaxConfiguration jaxConfiguration = new JaxConfiguration(serviceURL, suDH.getInstallRoot(),
className, edptName, suDH.getName());
final JaxConfigurationHandler jaxConfigHandler = new JaxConfigurationHandler(jaxConfiguration);
handlers.add( jaxConfigHandler );
jaxConfigHandler.start(deployer, this.logger);
// Register the handler in the component
getComponent().registerJaxConfigurationHandler(edptName, jaxConfigHandler);
} catch( MalformedURLException e ) {
throw new PEtALSCDKException( e );
}
}
}
@Override
protected void doUndeploy(final ServiceUnitDataHandler suDH) throws PEtALSCDKException {
final List handlers = this.suNameToJaxConfigHandler.remove(suDH.getName());
if( handlers != null ) {
final ConfigurationContext axisContext = getComponent().getAxisContext();
final PEtALSCDKException ex = new PEtALSCDKException("Error during undeploy");
for (JaxConfigurationHandler handler : handlers) {
getComponent().removeJaxConfigurationHandler(handler.getEndpointName());
try {
handler.stop(axisContext, this.logger);
} catch (final PEtALSCDKException e) {
ex.addSuppressed(e);
}
}
ex.throwIfNeeded();
}
}
@Override
protected Jsr181Se getComponent() {
return (Jsr181Se) super.getComponent();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy