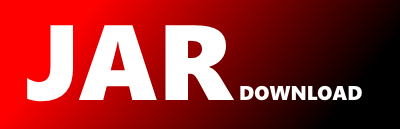
org.ow2.petals.se.jsr181.axis.Axis2Deployer Maven / Gradle / Ivy
/**
* Copyright (c) 2008-2012 EBM WebSourcing, 2012-2016 Linagora
*
* This program/library is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 2.1 of the License, or (at your
* option) any later version.
*
* This program/library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program/library; If not, see http://www.gnu.org/licenses/
* for the GNU Lesser General Public License version 2.1.
*/
package org.ow2.petals.se.jsr181.axis;
import java.lang.annotation.Annotation;
import java.net.URL;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.apache.axis2.deployment.util.Utils;
import org.apache.axis2.description.AxisOperation;
import org.apache.axis2.description.AxisService;
import org.apache.axis2.description.java2wsdl.AnnotationConstants;
import org.apache.axis2.jaxws.description.DescriptionFactory;
import org.apache.axis2.jaxws.server.JAXWSMessageReceiver;
import org.apache.axis2.util.Loader;
import org.ow2.petals.se.jsr181.Jsr181Se;
/**
* A class used to deploy the JAX-WS class as an Axis2 service.
*
* @author Christophe HAMERLING - EBM WebSourcing
* @author Vincent Zurczak - EBM WebSourcing
*/
public class Axis2Deployer {
/**
* The Axis2 configuration context
*/
private final Jsr181Se jsr181Se;
/**
* The component's logger.
*/
private final Logger logger;
/**
* Constructor.
* @param jsr181Se the component instance
*/
public Axis2Deployer( Jsr181Se jsr181Se ) {
this.jsr181Se = jsr181Se;
this.logger = jsr181Se.getLogger();
}
/**
* Deploys the annotated class as an Axis2 service.
* @param className the class name
* @param classloader the class loader into which the class must be loaded
* @param serviceLocation the service location
* @param suName the service-unit name (for log)
* @return the created Axis2 service (may be null)
* @throws Exception
*/
public AxisService deployJAXWS(
String className,
ClassLoader classloader,
URL serviceLocation,
String suName ) throws Exception {
AxisService axisService = null;
Class> jaxWsClass = Loader.loadClass( classloader, className );
if( this.logger.isLoggable( Level.FINE ))
this.logger.fine( suName + ": the " + className + " class was succesfully loaded." );
/**
* Schema generation done in two steps:
*
* 1. Load all the methods and create type for methods parameters (if the
* parameters are Bean then it will create Complex types for
* those , and if the parameters are simple type which
* describe in SimpleTypeTable nothing will happen).
*
* 2. In the next stage for all the methods messages and port types will be created
*/
boolean foundWsAnnotation = false;
for( Annotation ann : jaxWsClass.getAnnotations()) {
if( AnnotationConstants.WEB_SERVICE.equals( ann.annotationType().getName())) {
foundWsAnnotation = true;
axisService = this.createAxisService( serviceLocation, jaxWsClass );
/*
* There used to be a hack here for MEPs (synchronize Petals and Axis2 MEPs).
* Since the logs indicate Axis2 and Petals MEPs were the same,
* this hack was removed. Check the revision 15372 to see this hack in action.
*/
this.jsr181Se.getAxisContext().getAxisConfiguration().addService( axisService);
break;
}
}
if( this.logger.isLoggable( Level.FINE )) {
if( foundWsAnnotation )
this.logger.fine( suName + ": a JAX-WS annotated class was found." );
else
this.logger.fine( suName + ": no JAX-WS annotated class was found." );
}
return axisService;
}
/**
* Creates an Axis Service.
*
* @param serviceLocation the service location
* @param jaxWsClass the JAX-WS class
* @return the created Axis service
* @throws Exception if an error occurred
*/
private AxisService createAxisService( URL serviceLocation, Class> jaxWsClass ) throws Exception {
final AxisService axisService = DescriptionFactory.createAxisService(jaxWsClass, jsr181Se.getAxisContext());
// Create a wrapper for this service
if( axisService != null ) {
for( Iterator> operations = axisService.getOperations(); operations.hasNext(); ) {
AxisOperation axisOperation = (AxisOperation) operations.next();
if( axisOperation.getMessageReceiver() == null ) {
axisOperation.setMessageReceiver(new JAXWSMessageReceiver());
}
}
axisService.setElementFormDefault( false );
axisService.setFileName( serviceLocation );
Utils.fillAxisService(
axisService,
this.jsr181Se.getAxisContext().getAxisConfiguration(),
new ArrayList (),
new ArrayList ());
// Not needed at this case, the message receivers always set to RPC
// => setMessageReceivers(axisService);
}
return axisService;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy