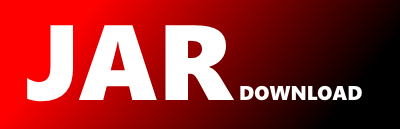
org.ow2.petals.ws.addressing.WsaHelper Maven / Gradle / Ivy
The newest version!
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2007 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id$
* -------------------------------------------------------------------------
*/
package org.ow2.petals.ws.addressing;
import java.net.URI;
import org.apache.axiom.om.OMElement;
import org.ow2.petals.ws.fault.WsnFault;
import org.ow2.petals.ws.util.AxiomHelper;
/**
* @author chamerling - eBM WebSourcing
*
*/
public class WsaHelper {
/**
*
* @param element
* @return
* @throws WsnFault
*/
public static MessageHeader messageHeaderFromOM(OMElement element)
throws WsnFault {
if (element == null) {
throw new WsnFault("Message header element is null");
}
MessageHeader result = new MessageHeader();
// to EPR
OMElement toElement = element
.getFirstChildWithName(WsaConstants.TO_QNAME);
if (toElement == null) {
throw new WsnFault("");
}
EndpointReference toEPR = new EndpointReference(URI.create(toElement
.getText()));
result.setToEPR(toEPR);
// from EPR
OMElement fromElement = element
.getFirstChildWithName(WsaConstants.FROM_QNAME);
if (fromElement != null) {
EndpointReference fromEpr = new EndpointReference(URI
.create(fromElement.getText()));
result.setFromEPR(fromEpr);
}
// fault EPR
OMElement faultElement = element
.getFirstChildWithName(WsaConstants.FAULT_TO_QNAME);
if (faultElement != null) {
EndpointReference faultEpr = new EndpointReference(URI
.create(faultElement.getText()));
result.setFromEPR(faultEpr);
}
// action
OMElement actionElement = element
.getFirstChildWithName(WsaConstants.ACTION_QNAME);
if (actionElement != null) {
result.setAction(actionElement.getText());
}
// message ID
OMElement messageIDElement = element
.getFirstChildWithName(WsaConstants.MESSAGE_ID_QNAME);
if (messageIDElement != null) {
result.setMessageID(messageIDElement.getText());
}
// relation
OMElement relationElement = element
.getFirstChildWithName(WsaConstants.RELATES_TO_QNAME);
if (relationElement != null) {
// TODO
}
return result;
}
/**
*
* @param header
* @return
*/
public static OMElement MessageHeaderToOM(MessageHeader header) {
OMElement result = AxiomHelper.createSOAPHeader();
String action = header.getAction();
if (action != null) {
OMElement element = AxiomHelper
.createOMElement(WsaConstants.ACTION_QNAME);
element.setText(action);
result.addChild(element);
}
EndpointReference faultEPR = header.getFaultToEPR();
if (faultEPR != null) {
OMElement element = AxiomHelper
.createOMElement(WsaConstants.FAULT_TO_QNAME);
element.setText(faultEPR.getAddress().toString());
result.addChild(element);
}
EndpointReference fromEPR = header.getFromEPR();
if (fromEPR != null) {
OMElement element = AxiomHelper
.createOMElement(WsaConstants.FROM_QNAME);
element.setText(fromEPR.getAddress().toString());
result.addChild(element);
}
String messageID = header.getMessageID();
if (messageID != null) {
OMElement element = AxiomHelper
.createOMElement(WsaConstants.MESSAGE_ID_QNAME);
element.setText(messageID);
result.addChild(element);
}
EndpointReference replyEPR = header.getReplyToEPR();
if (replyEPR != null) {
OMElement element = AxiomHelper
.createOMElement(WsaConstants.REPLY_TO_QNAME);
element.setText(replyEPR.getAddress().toString());
result.addChild(element);
}
EndpointReference toEPR = header.getToEPR();
if (toEPR != null) {
OMElement element = AxiomHelper
.createOMElement(WsaConstants.TO_QNAME);
element.setText(toEPR.getAddress().toString());
result.addChild(element);
}
return result;
}
/**
*
* @param element
* @return
* @throws WsnFault
*/
public static EndpointReference eprFromOM(OMElement element)
throws WsnFault {
if (element == null) {
throw new WsnFault("");
}
if (!element.getQName().equals(WsaConstants.ADDRESS_QNAME)) {
throw new WsnFault("Invalid element for EPR parsing");
}
EndpointReference epr = new EndpointReference(URI.create(element
.getText()));
return epr;
}
/**
*
* @param element
* @return
*/
public static OMElement eprToOM(EndpointReference epr) {
if (epr == null) {
// TODO
}
OMElement result = AxiomHelper
.createOMElement(WsaConstants.ADDRESS_QNAME);
result.setText(epr.getAddress().toString());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy