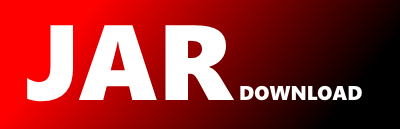
org.ow2.petals.ws.notification.Subscription Maven / Gradle / Ivy
The newest version!
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2007 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id$
* -------------------------------------------------------------------------
*/
package org.ow2.petals.ws.notification;
import java.util.Date;
import org.apache.axiom.soap.SOAPEnvelope;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.ow2.petals.ws.addressing.EndpointReference;
import org.ow2.petals.ws.client.SimpleSoapClient;
import org.ow2.petals.ws.client.SoapClient;
import org.ow2.petals.ws.client.WsnConsumerClient;
import org.ow2.petals.ws.fault.WsnFault;
/**
* Class used to manage subscription ono the server side. It makes the link
* between the notification consumer and provider.
*
* @author chamerling - eBM WebSourcing
*
*/
public class Subscription {
/**
* The client used to send messages to the notification consumers
*/
protected WsnConsumerClient client;
/**
* The EPR of the notification consumer
*/
protected EndpointReference consumerEPR;
/**
* The creation time of the current subscription
*/
protected Date creationTime;
/**
* The associated filter of the subscription
*/
protected Filter filter;
/**
* The EPR of the notification producer
*/
protected EndpointReference producerEPR;
/**
* The SOAP message which has created the current subscription
*/
protected SOAPEnvelope subscriptionEnvelope;
/**
* Logger
*/
protected static Log logger = LogFactory.getLog(Subscription.class);
/**
* Creates a new instance of {@link Subscription}
*
*/
public Subscription() {
this.creationTime = new Date();
}
/**
*
* @param soapClient
* @return
*/
protected WsnConsumerClient createConsumerClient(SoapClient soapClient) {
EndpointReference consumer = getConsumerEPR();
EndpointReference producer = getProducerEPR();
if (soapClient == null) {
soapClient = new SimpleSoapClient();
}
WsnConsumerClient client = new WsnConsumerClient(producer, consumer,
soapClient);
return client;
}
/**
*
* @return
*/
protected synchronized WsnConsumerClient getConsumerClient() {
if (this.client == null) {
this.client = createConsumerClient(null);
}
return this.client;
}
/**
*
* @param soapClient
* @return
*/
protected synchronized WsnConsumerClient getConsumerClient(
SoapClient soapClient) {
if (this.client == null) {
this.client = createConsumerClient(soapClient);
}
return this.client;
}
/**
*
* @return
*/
public EndpointReference getConsumerEPR() {
return consumerEPR;
}
public Date getCreationTime() {
return creationTime;
}
public Filter getFilter() {
return this.filter;
}
public EndpointReference getProducerEPR() {
return this.producerEPR;
}
/**
*
* @param consumer
*/
public void setConsumerEPR(EndpointReference consumer) {
if (consumer == null) {
throw new NullPointerException("Consumer can not be null");
}
this.consumerEPR = consumer;
}
/**
*
* @param filter
*/
public void setFilter(Filter filter) {
this.filter = filter;
}
/**
*
* @param producerEPR
*/
public void setProducerEPR(EndpointReference producerEPR) {
if (producerEPR == null) {
throw new NullPointerException("Producer can not be null");
}
this.producerEPR = producerEPR;
}
/**
* Publish a notification message
*
* @param message
*/
public SOAPEnvelope publish(NotificationMessage message) {
return this.publish(message, null);
}
/**
*
* @param message
* @param soapClient
*/
public SOAPEnvelope publish(NotificationMessage message, SoapClient soapClient) {
logger.debug("Publishing message");
SOAPEnvelope result = null;
if (!getFilter().accept(message)) {
return result;
}
message.setProducerEPR(getProducerEPR());
message.setSubscriptionEPR(getConsumerEPR());
try {
WsnConsumerClient client = getConsumerClient(soapClient);
result = client.notify(message);
if (logger.isDebugEnabled() && result != null) {
logger.debug("Notification result from consumer : " + result.toString());
}
} catch (WsnFault e) {
logger.error("Error while notifying consumer", e);
}
return result;
}
/**
*
* @return
*/
public SOAPEnvelope getSubscriptionEnvelope() {
return subscriptionEnvelope;
}
/**
*
* @param subscriptionEnvelope
*/
public void setSubscriptionEnvelope(SOAPEnvelope subscriptionEnvelope) {
this.subscriptionEnvelope = subscriptionEnvelope;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy