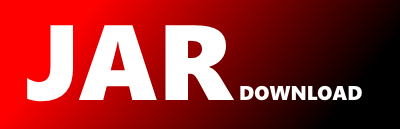
org.ow2.petals.ws.notification.WsnPersistance Maven / Gradle / Ivy
The newest version!
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2008 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id$
* -------------------------------------------------------------------------
*/
package org.ow2.petals.ws.notification;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
import javax.xml.namespace.QName;
import javax.xml.stream.FactoryConfigurationError;
import javax.xml.stream.XMLStreamException;
import org.apache.axiom.om.util.StAXUtils;
import org.apache.axiom.soap.SOAPEnvelope;
import org.apache.axiom.soap.impl.builder.StAXSOAPModelBuilder;
import org.ow2.petals.ws.topic.Topic;
import org.ow2.petals.ws.topic.WstHelper;
/**
* The persistence manager used to persist topics and subscription so that they
* can be reloaded when needed.
*
* @author Christophe HAMERLING - eBM WebSourcing
* @since 1.1
*
*/
public class WsnPersistance {
/**
* The persistence root path
*/
private File path;
/**
*
* @param path
* The root folder of the persistence. The folder is created if
* it do not exists.
*/
public WsnPersistance(File path) {
if (path == null) {
throw new NullPointerException("Persistance folder must not be null");
}
if (!path.exists()) {
path.mkdirs();
}
this.path = path;
}
/**
*
* @param subscription
* @throws Exception
*/
protected void persist(Subscription subscription) throws Exception {
SOAPEnvelope envelope = subscription.getSubscriptionEnvelope();
if (envelope == null) {
return;
}
File persist = getSubscriptionPersistanceFileName(subscription);
if (persist == null) {
throw new Exception("The persistance file can not be created");
}
if (!persist.exists()) {
persist.createNewFile();
}
envelope.serialize(new FileWriter(persist));
}
/**
* Persists the topic : Create a subfolder with the topic name.
*
* @param topic
*/
protected void persist(Topic topic) {
if (topic == null) {
throw new IllegalArgumentException("Topic is null, can not persist it");
}
File topicPath = new File(path, topic.getName());
if (!topicPath.exists()) {
topicPath.mkdirs();
}
// create the subfolers
File subsPath = new File(topicPath, "subscriptions");
if (!subsPath.exists()) {
subsPath.mkdirs();
}
}
/**
* Delete a subscription persistence file
*
* @param subscription
* @throws Exception
*/
public boolean delete(Subscription subscription) throws Exception {
boolean result = true;
String topicName = WstHelper.getRootTopicName(subscription.getFilter().getTopicName());
File topicPath = new File(path, topicName);
if (!topicPath.exists()) {
result = false;
} else {
File persist = getSubscriptionPersistanceFileName(subscription);
result = persist.delete();
}
return result;
}
/**
* Delete the Topic persistence folder
*
* @param topic
* @return
* @throws Exception
*/
protected boolean delete(Topic topic) {
return this.delete(topic.getName());
}
/**
* Delete the topic folder persistence
*
* @param topicName
* @return
*/
protected boolean delete(String topicName) {
File subsPath = getSubscriptionPath(topicName);
if (subsPath.exists() && subsPath.isDirectory()) {
File[] files = subsPath.listFiles();
for (File file : files) {
file.delete();
}
}
File topicPath = getTopicPath(topicName);
if (topicPath.exists() && topicPath.isDirectory()) {
File[] files = topicPath.listFiles();
for (File file : files) {
file.delete();
}
}
return topicPath.delete();
}
/**
*
* @param topicName
* @return
*/
protected List getSubscriptionEnvelopesForTopic(QName topicName) {
List result = new ArrayList();
File subsPath = getSubscriptionPath(WstHelper.getRootTopicName(topicName));
if (subsPath.exists()) {
File[] files = subsPath.listFiles();
for (File file : files) {
SOAPEnvelope env = loadEnvelope(file);
if (env != null) {
result.add(env);
}
}
}
return result;
}
/**
* Clean all the subscriptions persistence files in the subscription folder
*
* @param topicName
*/
protected void cleanSubscriptions(QName topicName) {
File subsPath = getSubscriptionPath(WstHelper.getRootTopicName(topicName));
if (subsPath.exists()) {
File[] files = subsPath.listFiles();
for (File file : files) {
file.delete();
}
}
}
/**
* Create the {@link SOAPEnvelope} from a file content
*
* @param f
* @return
*/
private SOAPEnvelope loadEnvelope(File f) {
SOAPEnvelope envelope = null;
try {
StAXSOAPModelBuilder builder = new StAXSOAPModelBuilder(StAXUtils
.createXMLStreamReader(new FileInputStream(f)), null);
envelope = (SOAPEnvelope) builder.getDocumentElement();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (XMLStreamException e) {
e.printStackTrace();
} catch (FactoryConfigurationError e) {
e.printStackTrace();
}
return envelope;
}
/**
*
* @param topicName
* @return
*/
private File getTopicPath(String topicName) {
return new File(path, topicName);
}
/**
*
* @param topicName
* @return
*/
private File getSubscriptionPath(String topicName) {
return new File(getTopicPath(topicName), "subscriptions");
}
/**
* Get an unique name for the persistance file. The file name is the
* consumer EPR + subscription date (as long) since the consumer can
* subscribe N times.
*
* @param subscription
* @return
*/
private File getSubscriptionPersistanceFileName(Subscription subscription) {
File result = null;
File subsPath = this.getSubscriptionPath(WstHelper.getRootTopicName(subscription
.getFilter().getTopicName()));
String fileName = subscription.getConsumerEPR().getAddress().toString() + "_"
+ subscription.getCreationTime().getTime();
try {
result = new File(subsPath, URLEncoder.encode(fileName, "UTF-8"));
} catch (UnsupportedEncodingException e) {
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy