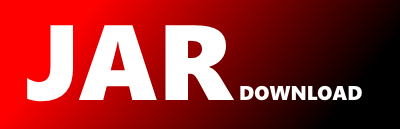
org.ow2.petals.ws.topic.Topic Maven / Gradle / Ivy
The newest version!
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2007 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id$
* -------------------------------------------------------------------------
*/
package org.ow2.petals.ws.topic;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import javax.xml.namespace.QName;
import org.ow2.petals.ws.notification.NotificationMessage;
/**
* @author chamerling - eBM WebSourcing
*
*/
public class Topic {
/**
* The map of child topics
*/
protected Map children = new LinkedHashMap();
/**
* The topic local name
*/
protected String name;
/**
*
*/
protected TopicNamespace topicNamespace;
/**
* The parent topic
*/
protected Topic parent;
/**
* The last published message
*/
protected NotificationMessage currentMessage;
/**
* The message types for the current topic
*/
protected Set messageTypes;
/**
* The topic is final, no further child topics can be added dynamically to
* the topic.
*/
protected boolean finalTopic = false;
/**
*
*/
protected String messagePattern;
/**
* Creates a new instance of {@link Topic}. The creation is recursive and
* will create all the child topics.
*
* @param name
* the name of the topic, the name can contains multiple topic
* names (separated by '/'). If so, the topic names are extracted
* and the child topics are created.
* @param topicNamespace
*/
public Topic(String name, TopicNamespace topicNamespace) {
int slash = name.indexOf('/');
int end = slash >= 0 ? slash : name.length();
this.topicNamespace = topicNamespace;
this.name = name.substring(0, end);
if (end < this.name.length()) {
String childName = name.substring(end + 1);
addTopic(new Topic(childName, topicNamespace));
}
}
/**
*
* @param child
*/
public void addTopic(Topic child) {
String name = child.getName();
child.setParent(this);
children.put(name, child);
}
public boolean isRoot() {
return (this.getParent() == null);
}
/**
* @return the children
*/
public Map getChildren() {
return children;
}
/**
* @param children
* the children to set
*/
public void setChildren(Map children) {
this.children = children;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name
* the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the parent
*/
public Topic getParent() {
return parent;
}
/**
* @param parent
* the parent to set
*/
public void setParent(Topic parent) {
this.parent = parent;
}
/**
* @return the currentMessage
*/
public NotificationMessage getCurrentMessage() {
return this.currentMessage;
}
/**
* @param currentMessage
* the currentMessage to set
*/
public void setCurrentMessage(NotificationMessage currentMessage) {
this.currentMessage = currentMessage;
}
/**
* @return the finalTopic
*/
public boolean isFinalTopic() {
return this.finalTopic;
}
/**
* @param finalTopic
* the finalTopic to set
*/
public void setFinalTopic(boolean finalTopic) {
this.finalTopic = finalTopic;
}
/**
* @return the messageTypes
*/
public Set getMessageTypes() {
return this.messageTypes;
}
/**
* @param messageTypes
* the messageTypes to set
*/
public void setMessageTypes(Set messageTypes) {
this.messageTypes = messageTypes;
}
/**
* @return the topicNamespace
*/
public TopicNamespace getTopicNamespace() {
return this.topicNamespace;
}
/**
* @param topicNamespace
* the topicNamespace to set
*/
public void setTopicNamespace(TopicNamespace topicNamespace) {
this.topicNamespace = topicNamespace;
}
/**
* @return the messagePattern
*/
public String getMessagePattern() {
return this.messagePattern;
}
/**
* @param messagePattern
* the messagePattern to set
*/
public void setMessagePattern(String messagePattern) {
this.messagePattern = messagePattern;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy