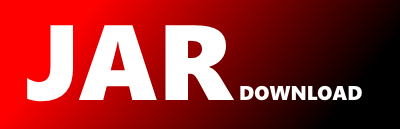
org.ow2.petals.ws.topic.TopicNamespace Maven / Gradle / Ivy
The newest version!
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2007 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id$
* -------------------------------------------------------------------------
*/
package org.ow2.petals.ws.topic;
import java.util.LinkedHashMap;
import java.util.Map;
import org.ow2.petals.ws.fault.WsnFault;
/**
* A forest of Topic trees grouped together into the same namespace for
* administrative purposes.
*
* @author chamerling - eBM WebSourcing
*
*/
public class TopicNamespace {
protected String targetNamespace;
protected String name;
protected Map rootTopics = new LinkedHashMap();
/**
* Creates a new instance of {@link TopicNamespace}
*
* @param targetNameSpace
*/
public TopicNamespace(String targetNameSpace) {
this.targetNamespace = targetNameSpace;
}
/**
* Add a topic to the topic namespace
*
* @param topic
* the topic to add
* @throws WsnFault
*/
public synchronized void addTopic(Topic topic) throws WsnFault {
if (topic == null) {
throw new NullPointerException("Topic can not be null");
}
String name = topic.getName();
if (rootTopics.containsKey(name)) {
throw new WsnFault("Topic is already registered in the topic namespace");
}
TopicNamespace topicSpace = topic.getTopicNamespace();
if (topicSpace != this) {
throw new WsnFault("Can not find the topic namespace for this topic");
}
rootTopics.put(name, topic);
}
/**
* Get a topic
*
* @param topicName
* @return the topic or null if not found
*/
public synchronized final Topic getTopic(String topicName) {
return rootTopics.get(topicName);
}
/**
* Remove the topic fro mthe topic namespace
*
* @param topicName
* the topic name of the topic to delete
* @return true if the topic has been deleted, false if it has not been
* found
*/
public synchronized boolean removeTopic(String topicName) {
Topic tmp = rootTopics.remove(topicName);
return tmp != null;
}
/**
* @return the name
*/
public String getName() {
return this.name;
}
/**
* @param name
* the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the targetNamespace
*/
public String getTargetNamespace() {
return this.targetNamespace;
}
/**
* @param targetNamespace
* the targetNamespace to set
*/
public void setTargetNamespace(String targetNamespace) {
this.targetNamespace = targetNamespace;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy