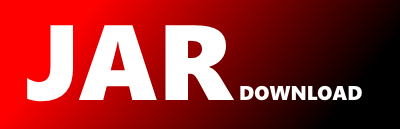
org.ow2.petals.ws.topic.WstHelper Maven / Gradle / Ivy
The newest version!
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2007 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id$
* -------------------------------------------------------------------------
*/
package org.ow2.petals.ws.topic;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
import java.util.StringTokenizer;
import javax.xml.namespace.QName;
import org.apache.axiom.om.OMElement;
import org.ow2.petals.ws.fault.InvalidTopicExpressionFault;
import org.ow2.petals.ws.fault.WsnFault;
/**
* @author chamerling - eBM WebSourcing
*
*/
public class WstHelper {
private WstHelper() {
}
/**
* Create a topic from an omElement
*
* @param element
* the element
* @return
*/
public static final Topic topicFromOM(OMElement element, TopicNamespace tns) throws WsnFault {
if (element == null) {
throw new NullPointerException("Topic element is null");
}
if (tns == null) {
throw new NullPointerException("Topic NS is null");
}
// get the topic name
String topicName = element.getAttributeValue(new QName("name"));
if (topicName == null || topicName.length() == 0) {
throw new InvalidTopicExpressionFault("No topic name");
}
Topic topic = new Topic(topicName, tns);
// topic is final ?
String finalValue = element.getAttributeValue(new QName(WstConstants.FINAL));
if (finalValue != null && finalValue.length() > 0) {
topic.setFinalTopic(Boolean.valueOf(finalValue).booleanValue());
}
// get the message types
String messageTypesValue = element.getAttributeValue(new QName(WstConstants.MESSAGE_TYPES));
Set messageTypeSet = new HashSet();
if (messageTypesValue != null && messageTypesValue.length() > 0) {
// the message types are separated by blanks like 'xyz:m1 tns:m2'
StringTokenizer tokenizer = new StringTokenizer(messageTypesValue, " ");
while (tokenizer.hasMoreTokens()) {
String tmp = tokenizer.nextToken();
// get a qname from the token
// FIXME : do a QName resolution
// QName qname = XmlUtils.parseQName(tmp, element);
// messageTypeSet.add(qname);
}
}
topic.setMessageTypes(messageTypeSet);
// get the pattern
OMElement patternElement = element.getFirstChildWithName(WstConstants.PATTERN_QNAME);
if (patternElement != null) {
topic.setMessagePattern(patternElement.getText());
}
Iterator iter = element.getChildrenWithName(WstConstants.TOPIC_QNAME);
while (iter.hasNext()) {
OMElement filterChildElement = (OMElement) iter.next();
Topic childTopic = WstHelper.topicFromOM(filterChildElement, tns);
topic.addTopic(topic);
}
return topic;
}
/**
* Create a topic NS from an omElement
*
* @param element
* @return
*/
public static final TopicNamespace topicNSFromOM(OMElement element) throws WsnFault {
if (element == null) {
throw new NullPointerException("Topic NS is null");
}
String targetNamespace = element.getAttributeValue(new QName("targetNamespace"));
if (targetNamespace == null) {
throw new InvalidTopicExpressionFault("No topic space NS");
}
TopicNamespace tns = new TopicNamespace(targetNamespace);
tns.setName(element.getAttributeValue(new QName("name")));
// get children (topics)
Iterator iter = element.getChildrenWithName(WstConstants.TOPIC_QNAME);
while (iter.hasNext()) {
Topic topic = topicFromOM((OMElement) iter.next(), tns);
tns.addTopic(topic);
}
return tns;
}
/**
* Get the name of the root topic. The names are seperated by '/' and are
* hierarchic; For example, for 'A/B/C', A is the root, B is its child. And
* B is also the parent of C.
*
* @param topicName
* @return
*/
public static final String getRootTopicName(QName topicName) {
String name = topicName.getLocalPart();
int slash = name.indexOf('/');
int end = slash >= 0 ? slash : name.length();
return name.substring(0, end);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy