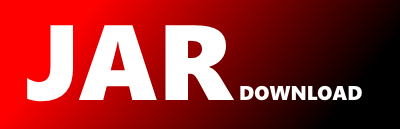
javax.ejb.ScheduleExpression Maven / Gradle / Ivy
The newest version!
/**
* EasyBeans
* Copyright (C) 2009-2011 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: ScheduleExpression.java 6276 2012-08-01 12:40:04Z benoitf $
* --------------------------------------------------------------------------
*/
package javax.ejb;
import java.io.Serializable;
import java.util.Date;
/**
* A calendar-based timeout expression for an enterprise bean timer.
* @see EJB 3.1 specification
* @author Florent Benoit
* @since EJB 3.1 version.
*/
public class ScheduleExpression implements Serializable {
/**
* Serial version UID.
*/
private static final long serialVersionUID = -3813254457230997879L;
private String dayOfMonth = "*";
private String dayOfWeek = "*";
private String hour = "0";
private String minute = "0";
private String month = "*";
private String second = "0";
private String year = "*";
private Date start;
private Date end;
private String timezoneID = "";
public ScheduleExpression dayOfMonth(int dayOfMonth) {
return dayOfMonth(Integer.toString(dayOfMonth));
}
public ScheduleExpression dayOfMonth(String dayOfMonth) {
this.dayOfMonth = dayOfMonth;
return this;
}
public ScheduleExpression dayOfWeek(int dayOfWeek) {
return dayOfWeek(Integer.toString(dayOfWeek));
}
public ScheduleExpression dayOfWeek(String dayOfWeek) {
this.dayOfWeek = dayOfWeek;
return this;
}
public ScheduleExpression end(Date e) {
this.end = e;
return this;
}
public String getDayOfMonth() {
return dayOfMonth;
}
public String getDayOfWeek() {
return dayOfWeek;
}
public Date getEnd() {
return end;
}
public String getHour() {
return hour;
}
public String getMinute() {
return minute;
}
public String getMonth() {
return month;
}
public String getSecond() {
return second;
}
public Date getStart() {
return start;
}
public String getTimezone() {
return timezoneID;
}
public String getYear() {
return year;
}
public ScheduleExpression hour(int hour) {
return hour(Integer.toString(hour));
}
public ScheduleExpression hour(String hour) {
this.hour = hour;
return this;
}
public ScheduleExpression minute(int minute) {
return minute(Integer.toString(minute));
}
public ScheduleExpression minute(String minute) {
this.minute = minute;
return this;
}
public ScheduleExpression month(int month) {
return month(Integer.toString(month));
}
public ScheduleExpression month(String month) {
this.month = month;
return this;
}
public ScheduleExpression second(int second) {
return second(Integer.toString(second));
}
public ScheduleExpression second(String second) {
this.second = second;
return this;
}
public ScheduleExpression start(Date start) {
this.start = start;
return this;
}
public ScheduleExpression timezone(String timezoneID) {
this.timezoneID = timezoneID;
return this;
}
public ScheduleExpression year(int year) {
return year(Integer.toString(year));
}
public ScheduleExpression year(String year) {
this.year = year;
return this;
}
/**
* @return stringified version.
*/
public String toString() {
StringBuilder sb = new StringBuilder(ScheduleExpression.class.getSimpleName());
sb.append("[second=");
sb.append(second);
sb.append(",minute=");
sb.append(minute);
sb.append(",hour=");
sb.append(hour);
sb.append(",dayOfMonth=");
sb.append(dayOfMonth);
sb.append(",month=");
sb.append(month);
sb.append(",dayOfWeek=");
sb.append(dayOfWeek);
sb.append(",year=");
sb.append(year);
sb.append(",timezoneID=");
sb.append(timezoneID);
sb.append(",start=");
sb.append(start);
sb.append(",end=");
sb.append(end);
sb.append("]");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy