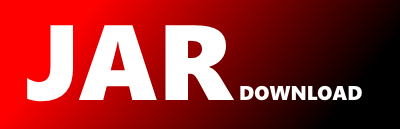
javax.jms.ConnectionFactory Maven / Gradle / Ivy
Show all versions of ow2-jms-2.0-spec Show documentation
/**
* Copyright 2013 ScalAgent Distributed Technologies
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ---------------------------------------------------------------------
* $Id: ConnectionFactory.java 6347 2013-03-13 08:52:02Z tachker $
* ---------------------------------------------------------------------
*/
package javax.jms;
/**
*
* A {@code ConnectionFactory} object encapsulates a set of configuration
* parameters defined by an administrator. A client needs to use it to create a
* connection with a Joram server.
*
* A ConnectionFactory object is a JMS administered object containing
* configuration information, it is created by an administrator and later used
* by JMS clients. Normally the JMS clients find administered objects by looking
* them up in a JNDI namespace.
*
* ConnectionFactory objects can be programmatically created using the
* LocalConnectionFactory.create or TcpConnectionFactory.create methods. Created
* objects can be then configured using FactoryParameters object.
*
* @see javax.jms.Connection
* @see javax.jms.QueueConnectionFactory
* @see javax.jms.TopicConnectionFactory
*
* @version JMS 2.0
* @since JMS 1.0
*
*/
public interface ConnectionFactory {
/**
* Creates a connection with the default user identity. The connection is
* created in stopped mode. No messages will be delivered until the
* {@code Connection.start} method is explicitly called.
*
* @return a newly created connection
*
* @exception JMSException
* if the JMS provider fails to create the connection due to some
* internal error.
* @exception JMSSecurityException
* if client authentication fails due to an invalid user name or
* password.
* @since JMS 1.1
*/
Connection createConnection() throws JMSException;
/**
* Creates a connection with the specified user identity. The connection is
* created in stopped mode. No messages will be delivered until the
* {@code Connection.start} method is explicitly called.
*
* @param userName
* the caller's user name
* @param password
* the caller's password
*
* @return a newly created connection
*
* @exception JMSException
* if the JMS provider fails to create the connection due to some
* internal error.
* @exception JMSSecurityException
* if client authentication fails due to an invalid user name or
* password.
* @since JMS 1.1
*/
Connection createConnection(String userName, String password)
throws JMSException;
/**
* Creates a JMSContext with the default user identity and an unspecified
* sessionMode.
*
* A connection and session are created for use by the new JMSContext. The
* connection is created in stopped mode but will be automatically started
* when a JMSConsumer is created.
*
*
* - The session will be non-transacted and received messages will be
* acknowledged automatically using an acknowledgement mode of
* {@code JMSContext.AUTO_ACKNOWLEDGE} For a definition of the meaning of this
* acknowledgement mode see the link below.
*
*
*
* @return a newly created JMSContext
*
* @exception JMSRuntimeException
* if the JMS provider fails to create the JMSContext due to some
* internal error.
* @exception JMSSecurityRuntimeException
* if client authentication fails due to an invalid user name or
* password.
* @since JMS 2.0
*
* @see JMSContext#AUTO_ACKNOWLEDGE
*
* @see javax.jms.ConnectionFactory#createContext(int)
* @see javax.jms.ConnectionFactory#createContext(java.lang.String,
* java.lang.String)
* @see javax.jms.ConnectionFactory#createContext(java.lang.String,
* java.lang.String, int)
* @see javax.jms.JMSContext#createContext(int)
*/
JMSContext createContext();
/**
* Creates a JMSContext with the specified user identity and an unspecified
* sessionMode.
*
* A connection and session are created for use by the new JMSContext. The
* connection is created in stopped mode but will be automatically started
* when a JMSConsumer.
*
The session will be non-transacted and received messages will be * acknowledged automatically by using acknowledgment mode of * {@code JMSContext.AUTO_ACKNOWLEDGE} * * @param userName * the caller's user name * @param password * the caller's password * * @return a newly created JMSContext * * @exception JMSRuntimeException * if the JMS provider fails to create the JMSContext due to some * internal error. * @exception JMSSecurityRuntimeException * if client authentication fails due to an invalid user name or * password. * @since JMS 2.0 * * @see JMSContext#AUTO_ACKNOWLEDGE * * @see javax.jms.ConnectionFactory#createContext() * @see javax.jms.ConnectionFactory#createContext(int) * @see javax.jms.ConnectionFactory#createContext(java.lang.String, * java.lang.String, int) * @see javax.jms.JMSContext#createContext(int) */ JMSContext createContext(String userName, String password); /** * Creates a JMSContext with the specified user identity and the specified * session mode. * * @return a newly created JMSContext * * @exception JMSRuntimeException * if the JMS provider fails to create the JMSContext due to some * internal error. * @exception JMSSecurityRuntimeException * if client authentication fails due to an invalid user name or * password. * @since JMS 2.0 * * @see JMSContext#SESSION_TRANSACTED * @see JMSContext#CLIENT_ACKNOWLEDGE * @see JMSContext#AUTO_ACKNOWLEDGE * @see JMSContext#DUPS_OK_ACKNOWLEDGE * * @see javax.jms.ConnectionFactory#createContext() * @see javax.jms.ConnectionFactory#createContext(int) * @see javax.jms.ConnectionFactory#createContext(java.lang.String, * java.lang.String) * @see javax.jms.JMSContext#createContext(int) */ JMSContext createContext(String userName, String password, int sessionMode); /** * Creates a JMSContext with the default user identity and the specified * session mode. * * @param sessionMode * indicates which of four possible session modes will be used. *
-
*
- If this method is called in a Java SE environment or in the * Java EE application client container, the permitted values are * {@code JMSContext.SESSION_TRANSACTED}, * {@code JMSContext.CLIENT_ACKNOWLEDGE}, * {@code JMSContext.AUTO_ACKNOWLEDGE} and * {@code JMSContext.DUPS_OK_ACKNOWLEDGE}. *
- If this method is called in the Java EE web or EJB container * when there is an active JTA transaction in progress then this * argument is ignored. *
- If this method is called in the Java EE web or EJB container * when there is no active JTA transaction in progress, the permitted * values are {@code JMSContext.AUTO_ACKNOWLEDGE} and * {@code JMSContext.DUPS_OK_ACKNOWLEDGE}. In this case the values * {@code JMSContext.TRANSACTED} and * {@code JMSContext.CLIENT_ACKNOWLEDGE} are not permitted. *
*