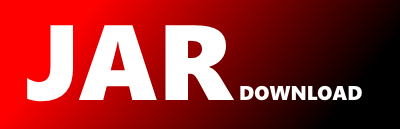
javax.persistence.EntityManagerFactory Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2010 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package javax.persistence;
import java.util.Map;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.metamodel.Metamodel;
/**
* Interface used to interact with the entity manager factory for the persistence unit.
* @see JPA 2.0 specification
* @author Florent Benoit
* @since JPA 2.0 version.
*/
public interface EntityManagerFactory {
/**
* Create a new application-managed EntityManager. This method returns a new EntityManager instance each time it is invoked.
* The isOpen method will return true on the returned instance.
* @return entity manager instance
* @throws IllegalStateException if the entity manager factory has been closed
*/
public EntityManager createEntityManager();
/**
* Create a new application-managed EntityManager with the specified Map of properties. This method returns a new
* EntityManager instance each time it is invoked. The isOpen method will return true on the returned instance.
* @param map properties for entity manager
* @return entity manager instance
* @throws IllegalStateException if the entity manager factory has been closed
*/
public EntityManager createEntityManager(Map map);
/**
* Return an instance of CriteriaBuilder for the creation of CriteriaQuery objects.
* @return CriteriaBuilder instance
* @throws IllegalStateException if the entity manager factory has been closed
*/
public CriteriaBuilder getCriteriaBuilder();
/**
* Return an instance of Metamodel interface for access to the metamodel of the persistence unit.
* @return Metamodel instance
* @throws IllegalStateException if the entity manager factory has been closed
*/
public Metamodel getMetamodel();
/**
* Indicates whether the factory is open. Returns true until the factory has been closed.
* @return boolean indicating whether the factory is open
*/
public boolean isOpen();
/**
* Close the factory, releasing any resources that it holds. After a factory instance has been closed, all methods invoked on
* it will throw the IllegalStateException, except for isOpen, which will return false. Once an EntityManagerFactory has been
* closed, all its entity managers are considered to be in the closed state.
* @throws IllegalStateException if the entity manager factory has been closed
*/
public void close();
/**
* Get the properties and associated values that are in effect for the entity manager factory. Changing the contents of the
* map does not change the configuration in effect.
* @return properties
* @throws IllegalStateException if the entity manager factory has been closed
*/
public Map getProperties();
/**
* Access the cache that is associated with the entity manager factory (the "second level cache").
* @return instance of the Cache interface
* @throws IllegalStateException if the entity manager factory has been closed
*/
public Cache getCache();
/**
* Return interface providing access to utility methods for the persistence unit.
* @return PersistenceUnitUtil interface
* @throws IllegalStateException if the entity manager factory has been closed
*/
public PersistenceUnitUtil getPersistenceUnitUtil();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy