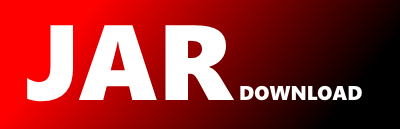
javax.persistence.criteria.From Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2010 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package javax.persistence.criteria;
import java.util.Set;
import javax.persistence.metamodel.CollectionAttribute;
import javax.persistence.metamodel.ListAttribute;
import javax.persistence.metamodel.MapAttribute;
import javax.persistence.metamodel.SetAttribute;
import javax.persistence.metamodel.SingularAttribute;
/**
* Represents a bound type, usually an entity that appears in
* the from clause, but may also be an embeddable belonging to
* an entity in the from clause.
* Serves as a factory for Joins of associations, embeddables, and
* collections belonging to the type, and for Paths of attributes
* belonging to the type.
* @param the source type
* @param the target type
* @see JPA 2.0 specification
* @author Florent Benoit
* @since JPA 2.0 version.
*/
public interface From extends Path, FetchParent {
/**
* Return the joins that have been made from this bound type.
* Returns empty set if no joins have been made from this
* bound type.
* Modifications to the set do not affect the query.
* @return joins made from this type
*/
Set> getJoins();
/**
* Whether the From object has been obtained as a result of
* correlation (use of a Subquery correlate method).
* @return boolean indicating whether the object has been
* obtained through correlation
*/
boolean isCorrelated();
/**
* Returns the parent From object from which the correlated
* From object has been obtained through correlation (use
* of a Subquery correlate method).
* @return the parent of the correlated From object
* @throws IllegalStateException if the From object has
* not been obtained through correlation
*/
From getCorrelationParent();
/**
* Create an inner join to the specified single-valued
* attribute.
* @param attribute target of the join
* @return the resulting join
*/
Join join(SingularAttribute super X, Y> attribute);
/**
* Create a join to the specified single-valued attribute
* using the given join type.
* @param attribute target of the join
* @param jt join type
* @return the resulting join
*/
Join join(SingularAttribute super X, Y> attribute,
JoinType jt);
/**
* Create an inner join to the specified Collection-valued
* attribute.
* @param collection target of the join
* @return the resulting join
*/
CollectionJoin join(
CollectionAttribute super X, Y> collection);
/**
* Create an inner join to the specified Set-valued attribute.
* @param set target of the join
* @return the resulting join
*/
SetJoin join(SetAttribute super X, Y> set);
/**
* Create an inner join to the specified List-valued attribute.
* @param list target of the join
* @return the resulting join
*/
ListJoin join(ListAttribute super X, Y> list);
/**
* Create an inner join to the specified Map-valued attribute.
* @param map target of the join
* @return the resulting join
*/
MapJoin join(MapAttribute super X, K, V> map);
/**
* Create a join to the specified Collection-valued attribute
* using the given join type.
* @param collection target of the join
* @param jt join type
* @return the resulting join
*/
CollectionJoin join(
CollectionAttribute super X, Y> collection, JoinType jt);
/**
* Create a join to the specified Set-valued attribute using
* the given join type.
* @param set target of the join
* @param jt join type
* @return the resulting join
*/
SetJoin join(SetAttribute super X, Y> set,
JoinType jt);
/**
* Create a join to the specified List-valued attribute using
* the given join type.
* @param list target of the join
* @param jt join type
* @return the resulting join
*/
ListJoin join(ListAttribute super X, Y> list,
JoinType jt);
/**
* Create a join to the specified Map-valued attribute using
* the given join type.
* @param map target of the join
* @param jt join type
* @return the resulting join
*/
MapJoin join(MapAttribute super X, K, V> map,
JoinType jt);
//String-based:
/**
* Create an inner join to the specified attribute.
* @param attributeName name of the attribute for the
* target of the join
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
Join join(String attributeName);
/**
* Create an inner join to the specified Collection-valued
* attribute.
* @param attributeName name of the attribute for the
* target of the join
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
CollectionJoin joinCollection(String attributeName);
/**
* Create an inner join to the specified Set-valued attribute.
* @param attributeName name of the attribute for the
* target of the join
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
SetJoin joinSet(String attributeName);
/**
* Create an inner join to the specified List-valued attribute.
* @param attributeName name of the attribute for the
* target of the join
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
ListJoin joinList(String attributeName);
/**
* Create an inner join to the specified Map-valued attribute.
* @param attributeName name of the attribute for the
* target of the join
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
MapJoin joinMap(String attributeName);
/**
* Create a join to the specified attribute using the given
* join type.
* @param attributeName name of the attribute for the
* target of the join
* @param jt join type
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
Join join(String attributeName, JoinType jt);
/**
* Create a join to the specified Collection-valued attribute
* using the given join type.
* @param attributeName name of the attribute for the
* target of the join
* @param jt join type
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
CollectionJoin joinCollection(String attributeName,
JoinType jt);
/**
* Create a join to the specified Set-valued attribute using
* the given join type.
* @param attributeName name of the attribute for the
* target of the join
* @param jt join type
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
SetJoin joinSet(String attributeName, JoinType jt);
/**
* Create a join to the specified List-valued attribute using
* the given join type.
* @param attributeName name of the attribute for the
* target of the join
* @param jt join type
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
ListJoin joinList(String attributeName, JoinType jt);
/**
* Create a join to the specified Map-valued attribute using
* the given join type.
* @param attributeName name of the attribute for the
* target of the join
* @param jt join type
* @return the resulting join
* @throws IllegalArgumentException if attribute of the given
* name does not exist
*/
MapJoin joinMap(String attributeName,
JoinType jt);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy