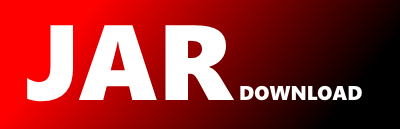
org.ow2.util.ant.archive.AbsArchive Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.ant.archive;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.ZipFileSet;
import org.ow2.util.ant.archive.info.ArchiveInfo;
/**
* Common task that creates archives.
* @author Florent Benoit
*/
public class AbsArchive extends Task {
/**
* Reference to the standard deployment descriptor.
*/
private File deploymentDescriptor = null;
/**
* Reference to the specific deployment descriptor.
*/
private File specificDeploymentDescriptor = null;
/**
* Full path to the archive.
*/
private File dest = null;
/**
* Relative Name of the archive (when embedded).
*/
private String name = null;
/**
* Exploded mode or not ? (default = file).
*/
private boolean exploded = false;
/**
* List of fileset used to add files.
*/
private List fileSetList = null;
/**
* Reference to the manifest.
*/
private File manifest = null;
/**
* Default constructor.
*/
public AbsArchive() {
this.fileSetList = new ArrayList();
}
/**
* Add the given fileset to the list of existing fileset.
* @param zipFileSet the fileset to add.
*/
public void addFileSet(final ZipFileSet zipFileSet) {
this.fileSetList.add(zipFileSet);
}
/**
* Gets the list of fileset to include in the archive.
* @return the list of fileset to include in the archive.
*/
public List getFileSetList() {
return this.fileSetList;
}
/**
* Sets the exploded mode to true or false.
* @param exploded boolean true/false
*/
public void setExploded(final boolean exploded) {
this.exploded = exploded;
}
/**
* Gets the state : exploded mode or not ?
* @return the state : exploded mode or not ?
*/
public boolean isExploded() {
return this.exploded;
}
/**
* Sets the reference to the deployment descriptor.
* @param dd the given deployment descriptor.
*/
public void setDD(final File dd) {
// Ignore empty value
if ("empty-value".equals(dd.getName())) {
return;
}
if (!dd.exists()) {
throw new BuildException("The given file '" + dd + "' for the deployment descriptor does not exist.");
}
this.deploymentDescriptor = dd;
}
/**
* Sets the reference to the specific deployment descriptor.
* @param dd the given specific deployment descriptor.
*/
public void setSpecificDD(final File dd) {
// Ignore empty value
if ("empty-value".equals(dd.getName())) {
return;
}
if (!dd.exists()) {
throw new BuildException("The given file '" + dd + "' for the deployment descriptor does not exist.");
}
this.specificDeploymentDescriptor = dd;
}
/**
* Sets the path to the archive that will be built.
* @param dest the reference to resulting archive path.
*/
public void setDest(final File dest) {
this.dest = dest;
}
/**
* Gets the path to the archive that will be built.
* @return the reference to resulting archive path.
*/
public File getDest() {
return this.dest;
}
/**
* Sets the relative Name of the archive (when embedded).
* @param name the relative Name of the archive.
*/
public void setName(final String name) {
this.name = name;
}
/**
* Gets the relative Name of the archive (when embedded).
* @return the relative Name of the archive.
*/
public String getName() {
return this.name;
}
/**
* Gets the reference to the manifest.
* @return the reference to the manifest.
*/
public File getManifest() {
return this.manifest;
}
/**
* Sets the reference to the manifest.
* @param manifest the reference to the manifest.
*/
public void setManifest(final File manifest) {
if (!manifest.exists()) {
throw new BuildException("The given file '" + manifest + "' for the MANIFEST does not exist.");
}
this.manifest = manifest;
}
/**
* Update the given archive info object with some attributes.
* @param archiveInfo the object to update
*/
protected void updateArchiveInfo(final ArchiveInfo archiveInfo) {
archiveInfo.setDest(this.dest);
archiveInfo.setDD(this.deploymentDescriptor);
archiveInfo.setSpecificDD(this.specificDeploymentDescriptor);
archiveInfo.setExploded(this.exploded);
archiveInfo.setManifest(this.manifest);
// Add the given fileset
for (FileSet fileSet : getFileSetList()) {
archiveInfo.addFileSet(fileSet);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy