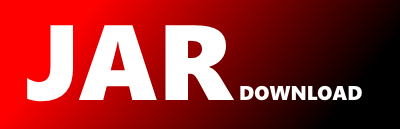
org.ow2.util.ant.archive.Ear Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.ant.archive;
import java.util.ArrayList;
import java.util.List;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.types.ZipFileSet;
import org.ow2.util.ant.archive.api.IEar;
import org.ow2.util.ant.archive.exploded.EarExploded;
import org.ow2.util.ant.archive.file.EarFile;
import org.ow2.util.ant.archive.info.EarInfo;
/**
* Task that creates an Ear archive (.ear file or .ear directory).
* @author Florent Benoit
*/
public class Ear extends AbsArchive {
/**
* List of War to package in this EAR.
*/
private List wars = null;
/**
* List of EJB to package in this EAR.
*/
private List ejbs = null;
/**
* List of Application Client to package in this EAR.
*/
private ArrayList clients = null;
/**
* lib folder.
*/
private static final String LIB_FOLDER = "lib/";
/**
* Default constructor.
*/
public Ear() {
super();
this.wars = new ArrayList();
this.ejbs = new ArrayList();
this.clients = new ArrayList();
}
/**
* Add files in lib folder.
* @param zipFileSet the fileset that contains the files.
*/
public void addLib(final ZipFileSet zipFileSet) {
zipFileSet.setPrefix(LIB_FOLDER);
addFileSet(zipFileSet);
}
/**
* Add a given war archive.
* @param war the given archive
*/
public void addConfiguredWar(final War war) {
this.wars.add(war);
}
/**
* Add a given Ejb archive.
* @param ejb the given archive
*/
public void addConfiguredEjb(final Ejb ejb) {
this.ejbs.add(ejb);
}
/**
* Add a given Application Client archive.
* @param client the given archive
*/
public void addConfiguredClient(final Client client) {
this.clients.add(client);
}
/**
* Execute the task.
*/
@Override
public void execute() {
log("Building Ear in '" + getDest() + "'.", Project.MSG_INFO);
IEar ear = null;
// 2 cases, exploded mode or not
if (isExploded()) {
ear = new EarExploded(getProject());
} else {
ear = new EarFile(getProject());
}
// Set the name of the task
((Task) ear).setTaskName(getTaskName());
// Build the info object
EarInfo earInfo = new EarInfo();
ear.setEarInfo(earInfo);
// Fill archive properties
updateArchiveInfo(earInfo);
// set children
earInfo.setEjbs(this.ejbs);
earInfo.setWars(this.wars);
earInfo.setClients(this.clients);
// Execute the task
ear.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy