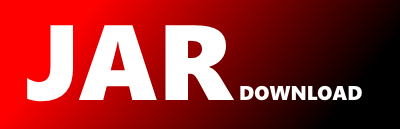
org.ow2.util.ant.archive.exploded.AbsExplodedArchive Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.ant.archive.exploded;
import java.io.File;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.Task;
import org.apache.tools.ant.taskdefs.Copy;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.ZipFileSet;
import org.ow2.util.ant.archive.api.IArchive;
import org.ow2.util.ant.archive.info.ArchiveInfo;
/**
* Abstract task for creating exploded archive.
* @author Florent Benoit
*/
public abstract class AbsExplodedArchive extends Task implements IArchive {
/**
* Path to the Persistence deployment descriptor.
*/
private static final String PERSISTENCE_DEPLOYMENT_DESCRIPTOR = "META-INF/persistence.xml";
/**
* Reference to the archive info object.
*/
private ArchiveInfo archiveInfo = null;
/**
* Creates an archive for the given project.
* @param p the given project
*/
public AbsExplodedArchive(final Project p) {
super();
setProject(p);
}
/**
* Gets the path to the standard deployment descriptor.
* @return the path to the standard deployment descriptor.
*/
public abstract String getDDStandardName();
/**
* Gets the path to the specific deployment descriptor.
* @return the path to the specific deployment descriptor.
*/
public abstract String getDDSpecificame();
/**
* Sets the information about an archive.
* @param archiveInfo the object that holds data information.
*/
public void setArchiveInfo(final ArchiveInfo archiveInfo) {
this.archiveInfo = archiveInfo;
}
/**
* Add the standard deployment descriptor in the archive.
*/
public void addDD() {
if (archiveInfo.getDD() != null) {
// Add a fileset
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setProject(getProject());
zipFileSet.setFile(archiveInfo.getDD());
zipFileSet.setFullpath(getDDStandardName());
archiveInfo.getFileSetList().add(zipFileSet);
}
if (archiveInfo.getSpecificDD() != null) {
// Add a fileset
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setProject(getProject());
zipFileSet.setFile(archiveInfo.getSpecificDD());
zipFileSet.setFullpath(getDDSpecificame());
archiveInfo.getFileSetList().add(zipFileSet);
}
// persistence dd if any
if (archiveInfo.getPersistenceDD() != null) {
// Add a fileset
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setProject(getProject());
zipFileSet.setFile(archiveInfo.getPersistenceDD());
zipFileSet.setFullpath(PERSISTENCE_DEPLOYMENT_DESCRIPTOR);
archiveInfo.getFileSetList().add(zipFileSet);
}
}
/**
* Execute the task.
*/
@Override
public void execute() {
// Include the DD if any
addDD();
// Suffix dest dir with the name of the file
File destdir = archiveInfo.getDest();
// For each fileset, execute a copy
for (FileSet fileSet : archiveInfo.getFileSetList()) {
Copy copy = new Copy();
copy.setProject(getProject());
copy.setTaskName(getTaskName());
copy.setTodir(destdir);
// Change the dest dir if there is a prefix
if (ZipFileSet.class.getName().equals(fileSet.getClass().getName())) {
ZipFileSet archiveFileSet = (ZipFileSet) fileSet;
String prefix = archiveFileSet.getPrefix(getProject());
if (prefix != null && !prefix.equals("")) {
File toDir = new File(destdir, prefix);
copy.setTodir(toDir);
}
String fullpath = archiveFileSet.getFullpath(getProject());
if (fullpath != null && !fullpath.equals("")) {
File toFile = new File(destdir, fullpath);
copy.setTodir(null);
copy.setTofile(toFile);
}
}
copy.addFileset(fileSet);
copy.execute();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy