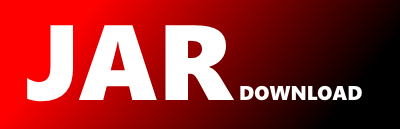
org.ow2.util.ant.archive.file.ClientFile Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.ant.archive.file;
import java.io.File;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.Jar;
import org.apache.tools.ant.taskdefs.Manifest;
import org.apache.tools.ant.taskdefs.ManifestException;
import org.apache.tools.ant.taskdefs.Manifest.Attribute;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.ZipFileSet;
import org.ow2.util.ant.archive.api.IClient;
import org.ow2.util.ant.archive.info.ArchiveInfo;
import org.ow2.util.ant.archive.info.ClientInfo;
/**
* Creates a Client file.
* @author Florent Benoit
*/
public class ClientFile extends Jar implements IClient {
/**
* Path to the Standard deployment descriptor.
*/
private static final String DEPLOYMENT_DESCRIPTOR = "META-INF/application-client.xml";
/**
* Path for the JOnAS Specific deployment descriptor.
*/
private static final String SPECIFIC_DEPLOYMENT_DESCRIPTOR = "META-INF/jonas-client.xml";
/**
* Reference to the client archive info object.
*/
private ClientInfo clientInfo = null;
/**
* Reference to the archive info object.
*/
private ArchiveInfo archiveInfo = null;
/**
* Creates an archive for the given project.
* @param p the given project
*/
public ClientFile(final Project p) {
super();
setProject(p);
}
/**
* Sets the information about an archive.
* @param archiveInfo the object that holds data information.
*/
public void setArchiveInfo(final ArchiveInfo archiveInfo) {
this.archiveInfo = archiveInfo;
}
/**
* Sets the information about a Client archive.
* @param clientInfo the object that holds data information.
*/
public void setClientInfo(final ClientInfo clientInfo) {
setArchiveInfo(clientInfo);
this.clientInfo = clientInfo;
}
/**
* Execute the task.
*/
@Override
public void execute() {
// Deployment descriptor
if (this.archiveInfo.getDD() != null) {
setDD(this.archiveInfo.getDD());
}
// Specific Deployment descriptor
if (this.archiveInfo.getSpecificDD() != null) {
setSpecificDD(this.archiveInfo.getSpecificDD());
}
// Manifest
if (this.archiveInfo.getManifest() != null) {
setManifest(this.archiveInfo.getManifest());
}
// Main class attribute ?
String mainClass = this.clientInfo.getMainClass();
if (mainClass != null) {
Manifest mf = new Manifest();
Attribute attribute = new Attribute();
attribute.setName("Main-Class");
attribute.setValue(mainClass);
// Add attribute
try {
mf.addConfiguredAttribute(attribute);
} catch (ManifestException e) {
throw new BuildException("Cannot add the Main-Class attribute in the manifest", e);
}
// Add Manifest
try {
addConfiguredManifest(mf);
} catch (ManifestException e) {
throw new BuildException("Cannot add the manifest", e);
}
}
// dest file
setDestFile(this.archiveInfo.getDest());
// fileset
for (FileSet fileSet : this.archiveInfo.getFileSetList()) {
addFileset(fileSet);
}
super.execute();
}
/**
* Add the given DD file into the archive.
* @param dd the path to the DDesc file.
*/
public void setDD(final File dd) {
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setFile(dd);
zipFileSet.setFullpath(DEPLOYMENT_DESCRIPTOR);
addFileset(zipFileSet);
}
/**
* Add the given Specific DD file into the archive.
* @param dd the path to the DDesc file.
*/
public void setSpecificDD(final File dd) {
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setFile(dd);
zipFileSet.setFullpath(SPECIFIC_DEPLOYMENT_DESCRIPTOR);
addFileset(zipFileSet);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy