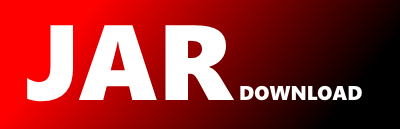
org.ow2.util.ant.archive.file.EarFile Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.ant.archive.file;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.Jar;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.ZipFileSet;
import org.ow2.util.ant.archive.AbsArchive;
import org.ow2.util.ant.archive.Client;
import org.ow2.util.ant.archive.Ejb;
import org.ow2.util.ant.archive.War;
import org.ow2.util.ant.archive.api.IEar;
import org.ow2.util.ant.archive.info.ArchiveInfo;
import org.ow2.util.ant.archive.info.EarInfo;
/**
* Creates an EAR file.
* @author Florent Benoit
*/
public class EarFile extends Jar implements IEar {
/**
* Path to the Standard deployment descriptor.
*/
private static final String DEPLOYMENT_DESCRIPTOR = "META-INF/application.xml";
/**
* Ear info object.
*/
private EarInfo earInfo = null;
/**
* Creates an archive for the given project.
* @param p the given project
*/
public EarFile(final Project p) {
super();
setProject(p);
}
/**
* Sets the information about an EAR archive.
* @param earInfo the object that holds data information.
*/
public void setEarInfo(final EarInfo earInfo) {
this.earInfo = earInfo;
}
/**
* Execute the task.
*/
@Override
public void execute() {
// First, package each ejb, war, etc. (sub modules)
// Execute children by changing the destination directory
List ejbs = this.earInfo.getEjbs();
List wars = this.earInfo.getWars();
List clients = this.earInfo.getClients();
// Add all archives
List archives = new ArrayList();
if (ejbs != null) {
for (Ejb ejb : ejbs) {
archives.add(ejb);
}
}
if (wars != null) {
for (War war : wars) {
archives.add(war);
}
}
if (clients != null) {
for (Client client : clients) {
archives.add(client);
}
}
// Update archives
for (AbsArchive archive : archives) {
// Update path
updateArchive(archive);
// Build the file
archive.execute();
// Now, add each archive built in the fileset of the Ear
addArchiveInFileSet(archive);
}
// Deployment descriptor
if (this.earInfo.getDD() != null) {
setDD(this.earInfo.getDD());
}
// Manifest
if (this.earInfo.getManifest() != null) {
setManifest(this.earInfo.getManifest());
}
// dest file
setDestFile(this.earInfo.getDest());
// fileset
for (FileSet fileSet : this.earInfo.getFileSetList()) {
addFileset(fileSet);
}
// Create directory if it is not existing
File earFileParentDirectory = this.earInfo.getDest().getParentFile();
if (!earFileParentDirectory.exists()) {
log("Creating directory '" + earFileParentDirectory + "'", Project.MSG_INFO);
earFileParentDirectory.mkdirs();
}
super.execute();
// Clean all temporary files
for (AbsArchive archive : archives) {
File tmpFile = archive.getDest();
if (tmpFile.exists()) {
tmpFile.delete();
}
}
}
/**
* Update settings of the given archive.
* @param archive the archive to update.
*/
void updateArchive(final AbsArchive archive) {
// change exploded mode to false if ear level is false
if (archive.isExploded() && !this.earInfo.isExploded()) {
log("Changing exploded mode to false as the EAR is not in exploded mode.", Project.MSG_INFO);
archive.setExploded(false);
}
// name is defined ? if not set it to the last name of the dest
if (archive.getName() == null) {
archive.setName(archive.getDest().getName());
}
try {
archive.setDest(File.createTempFile("easybeans-ant", ".tmp"));
} catch (IOException e) {
throw new BuildException("Cannot create a temp file", e);
}
// Delete the temporary file, it should be created by the archive
// packaging
archive.getDest().delete();
}
/**
* Add the given DD file into the archive.
* @param dd the path to the DDesc file.
*/
public void setDD(final File dd) {
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setFile(dd);
zipFileSet.setFullpath(DEPLOYMENT_DESCRIPTOR);
addFileset(zipFileSet);
}
/**
* Add the given archive in a fileset.
* @param archive the path to the file to include.
*/
public void addArchiveInFileSet(final AbsArchive archive) {
ZipFileSet zipFileSet = new ZipFileSet();
zipFileSet.setFile(archive.getDest());
zipFileSet.setFullpath(archive.getName());
addFileset(zipFileSet);
}
/**
* Sets the information about an archive.
* @param archiveInfo the object that holds data information.
*/
public void setArchiveInfo(final ArchiveInfo archiveInfo) {
// nothing
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy