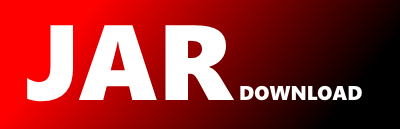
org.ow2.util.archive.impl.ArchiveManager Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.archive.impl;
import java.util.HashMap;
import java.util.Map;
import org.ow2.util.archive.api.IArchive;
import org.ow2.util.archive.api.IArchiveFactory;
import org.ow2.util.archive.api.IArchiveManager;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
/**
* Creates an archive for the given type.
* @author Florent Benoit
*/
public final class ArchiveManager implements IArchiveManager {
/**
* Logger.
*/
private static Log logger = LogFactory.getLog(ArchiveManager.class);
/**
* Unique instance of this class.
*/
private static ArchiveManager archiveManager;
/**
* Mapping between class and the associated factories.
* ie : File <--> DefaultArchiveFactory.
*/
private Map, IArchiveFactory>> factories = new HashMap, IArchiveFactory>>();
/**
* Private constructor as only one instance is built.
*/
private ArchiveManager() {
addFactory(new DefaultArchiveFactory());
addFactory(new URLArchiveFactory());
}
/**
* Gets the unique instance of this class.
* @return the unique instance.
*/
public static ArchiveManager getInstance() {
if (archiveManager == null) {
archiveManager = new ArchiveManager();
}
return archiveManager;
}
/**
* Adds the given factory on this manager. It will look the generic info
* used by this class to find the class object managed by this factory.
* @param factory the factory to add.
*/
public void addFactory(final IArchiveFactory> factory) {
Class> factoryClass = factory.getClass();
Class> argumentClass = factory.getSupportedClass();
logger.debug("Adding factory '" + factoryClass.getName() + "' for class '" + argumentClass + "'.");
// Add factory
factories.put(argumentClass, factory);
}
/**
* Removes the given factory on this manager. It will look the generic info
* used by this class to find the class object managed by this factory.
* @param factory the factory to remove.
*/
public void removeFactory(final IArchiveFactory> factory) {
Class> factoryClass = factory.getClass();
Class> argumentClass = factory.getSupportedClass();
if (factories.containsKey(argumentClass)) {
logger.debug("Removing factory '" + factoryClass.getName() + "' for class '" + argumentClass + "'.");
// Remove factory
factories.remove(argumentClass);
} else {
logger.debug("Factory '" + factoryClass.getName() + "' was not registered in the ArchiveManager.");
}
}
/**
* Creates an EZBArchive implementation object for the given object.
* @param o object to wrap into an EZBArchive.
* @return the created archive.
*/
@SuppressWarnings("unchecked")
public IArchive getArchive(final Object o) {
// try to see if there is a matching factory
Class objectClass = o.getClass();
// Factory ?
IArchiveFactory factory = factories.get(objectClass);
if (factory == null) {
// Iterates over the registered classes and find the assignable class
for (Class cls : factories.keySet()) {
if (cls.isAssignableFrom(objectClass) && factory == null) {
// we found a match
factory = factories.get(cls);
}
}
// if after all, factory is still null, raise an Exception
if (factory == null) {
throw new IllegalArgumentException("No factory found for the type '" + objectClass + "'.");
}
}
return factory.create(o);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy