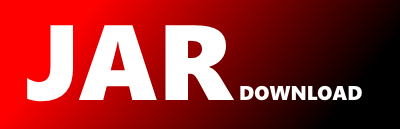
org.ow2.util.archive.impl.FileArchiveImpl Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.archive.impl;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.Iterator;
import org.ow2.util.archive.api.ArchiveException;
import org.ow2.util.archive.api.IArchiveMetadata;
import org.ow2.util.archive.api.IFileArchive;
import org.ow2.util.log.Log;
import org.ow2.util.log.LogFactory;
import org.ow2.util.url.URLUtils;
/**
* An implementation for the IFileArchive class.
* @author mleduque
*/
public class FileArchiveImpl implements IFileArchive {
/**
* Logger.
*/
private static final Log logger = LogFactory.getLog(FileArchiveImpl.class);
/**
* The file of the archive.
*/
private File file = null;
/**
* Cached URL of this archive.
*/
private URL url = null;
/**
* Constructor with no arguments.
*/
public FileArchiveImpl() {
}
/**
* Constructs a file archive wit the given file.
* @param file the given file
*/
public FileArchiveImpl(final File file) {
setFile(file);
}
/**
* {@inheritDoc}
*/
public void setFile(final File file) {
// TODO, Shouldn't we allow this to change only once ???
try {
this.file = file.getCanonicalFile();
} catch (IOException e) {
logger.debug("File ''{0}'' cannot be canonicalized, use it as is", file);
this.file = file;
}
this.url = URLUtils.fileToURL(this.file);
}
/**
* {@inheritDoc}
*/
public File getFile() {
return this.file;
}
/**
* {@inheritDoc}
*/
public boolean close() {
return true;
}
/**
* {@inheritDoc}
*/
public IArchiveMetadata getMetadata() {
return null;
}
/**
* {@inheritDoc}
*/
public String getName() {
return file.getPath();
}
/**
* {@inheritDoc}
*/
public URL getResource(final String resourceName) throws ArchiveException {
throw new ArchiveException("No resources inside this file");
}
/**
* {@inheritDoc}
*/
public Iterator getResources() throws ArchiveException {
throw new ArchiveException("No resources inside this file");
}
/**
* {@inheritDoc}
*/
public Iterator getResources(final String resourceName)
throws ArchiveException {
throw new ArchiveException("No resources inside this file");
}
/**
* {@inheritDoc}
*/
public URL getURL() throws ArchiveException {
return this.url;
}
/**
* {@inheritDoc}
*/
public Iterator getEntries() throws ArchiveException {
return null;
}
/**
* @return a string representation of the object.
*/
@Override
public String toString() {
return getName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy