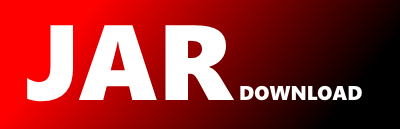
org.ow2.util.archive.impl.MemoryArchive Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.archive.impl;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.ow2.util.archive.api.IArchive;
/**
* Emulate an archive in memory.
* @author Gael Lalire
*/
public class MemoryArchive extends AbsArchiveImpl implements IArchive {
/**
* Map which associate an url to a class name.
*/
private Map urlMap;
/**
* Construct an archive in memory.
*/
public MemoryArchive() {
this.urlMap = new HashMap();
}
/**
* Construct an archive in memory.
* @param classLoader this classloader is use to load class by their name
* @param classNames the name of classes in archive
*/
public MemoryArchive(final ClassLoader classLoader, final List classNames) {
this.urlMap = new HashMap();
addClassResource(classLoader, classNames);
}
/**
* Close the archive.
* @return true
*/
public boolean close() {
return true;
}
/**
* @return ArchiveInMemory
*/
public String getName() {
return "ArchiveInMemory";
}
/**
* @param resourceName the resource name
* @return the url of a class.
*/
public URL getResource(final String resourceName) {
return this.urlMap.get(resourceName);
}
/**
* @return all classes url
*/
public Iterator getResources() {
return this.urlMap.values().iterator();
}
/**
* @param resourceName the resource name
* @return a singleton iterator with url of the class
*/
public Iterator getResources(final String resourceName) {
LinkedList linkedList = new LinkedList();
linkedList.add(this.urlMap.get(resourceName));
return linkedList.iterator();
}
/**
* The memory archive has no url.
* @return null
*/
public URL getURL() {
try {
return new URL("file:///" + System.identityHashCode(this));
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Cannot build URL", e);
}
}
/**
* @param resourceName the resource name
* @param url the url
*/
public void addResource(final String resourceName, final URL url) {
urlMap.put(resourceName, url);
}
/**
* @param classLoader this classloader is use to load class by their name
* @param classNames the name of classes in archive
*/
public void addClassResource(final ClassLoader classLoader, final List classNames) {
for (String className : classNames) {
String resourceName = className.replace('.', '/').concat(".class");
this.urlMap.put(resourceName, classLoader.getResource(resourceName));
}
}
/**
* @return all resources name
*/
public Iterator getEntries() {
return urlMap.keySet().iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy