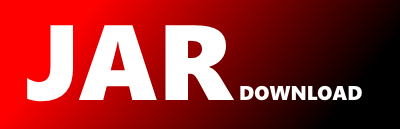
org.ow2.util.archive.impl.URLArchiveImpl Maven / Gradle / Ivy
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.archive.impl;
import java.io.IOException;
import java.net.URL;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.jar.JarInputStream;
import java.util.jar.Manifest;
import java.util.zip.ZipEntry;
import org.ow2.util.archive.api.IArchive;
/**
* Implementation of an archive from an url targeting a jar.
* @author Gael Lalire
*/
public class URLArchiveImpl extends AbsArchiveImpl implements IArchive {
/**
* Entries in jar.
*/
private Map entries;
/**
* Jar url.
*/
private URL url;
/**
* Constructor.
* @param url the url targeting a jar
* @throws IOException if jar could not be read
*/
public URLArchiveImpl(final URL url) throws IOException {
this.url = url;
entries = new HashMap();
JarInputStream jarInputStream = new JarInputStream(url.openStream());
Manifest manifest = jarInputStream.getManifest();
// Read manifest
if (manifest != null) {
readManifest(manifest);
}
ZipEntry zipEntry = jarInputStream.getNextEntry();
while (zipEntry != null) {
String name = zipEntry.getName();
entries.put(name, new URL("jar:" + url + "!/" + name));
zipEntry = jarInputStream.getNextEntry();
}
jarInputStream.close();
}
/**
* Close the archive.
* @return true
*/
public boolean close() {
return true;
}
/**
* Get archive name.
* @return the url path
*/
public String getName() {
return url.getPath();
}
/**
* @param resourceName the resource name
* @return a resource with resourceName
*/
public URL getResource(final String resourceName) {
return entries.get(resourceName);
}
/**
* @return all resources in jar
*/
public Iterator getResources() {
return entries.values().iterator();
}
/**
* @param resourceName the resource name
* @return all resources with resourceName
*/
public Iterator getResources(final String resourceName) {
List urlList = new LinkedList();
URL url = entries.get(resourceName);
if (url != null) {
urlList.add(url);
}
return urlList.iterator();
}
/**
* @return the url of archive
*/
public URL getURL() {
return url;
}
/**
* @return all resources name
*/
public Iterator getEntries() {
return entries.keySet().iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy