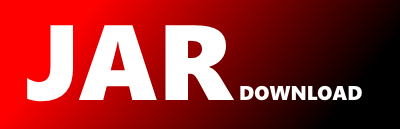
org.ow2.util.geolocation.business.entity.TechnicalAttributes Maven / Gradle / Ivy
/**
* Copyright 2009-2012 Serli
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.geolocation.business.entity;
import java.io.Serializable;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
/**
* Entity class for technical specification of users computer.
*
* @author Mathieu ANCELIN
*/
@Entity
public class TechnicalAttributes implements Serializable {
/**
* The version UID.
*/
private static final long serialVersionUID = 1L;
/**
* The id of the product name.
*/
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
/**
* The number of processors.
*/
private String numProc;
/**
* The jre version.
*/
private String jreVersion;
/**
* The jre vendor name.
*/
private String jreVendor;
/**
* The JVM version.
*/
private String jvmVersion;
/**
* The JVM vendor name.
*/
private String jvmVendor;
/**
* The JVM name.
*/
private String jvmName;
/**
* The OS name.
*/
private String osName;
/**
* The OS architecture.
*/
private String osArch;
/**
* The version of the OS.
*/
private String osVersion;
/**
* @return the id of the product name.
*/
public Long getId() {
return id;
}
/**
* Set the id of the product name.
* @param id the id of the product name.
*/
public void setId(final Long id) {
this.id = id;
}
@Override
public int hashCode() {
int hash = 0;
if (id != null) {
hash += id.hashCode();
} else {
hash += 0;
}
return hash;
}
@Override
public boolean equals(final Object object) {
if (!(object instanceof ProductName)) {
return false;
}
TechnicalAttributes other = (TechnicalAttributes) object;
if ((this.id == null && other.id != null) || (this.id != null && !this.id.equals(other.id))) {
return false;
}
return true;
}
@Override
public String toString() {
return "org.ow2.util.geolocation.business.entity.TechnicalAttributes[id=" + id + "]";
}
/**
* @return the jre vendor.
*/
public String getJreVendor() {
return jreVendor;
}
/**
*
* @param jreVendor the jre vendor.
*/
public void setJreVendor(final String jreVendor) {
this.jreVendor = jreVendor;
}
/**
*
* @return the jre version.
*/
public String getJreVersion() {
return jreVersion;
}
/**
*
* @param jreVersion the jre version.
*/
public void setJreVersion(final String jreVersion) {
this.jreVersion = jreVersion;
}
/**
*
* @return the jvm name
*/
public String getJvmName() {
return jvmName;
}
/**
*
* @param jvmName the jvm name.
*/
public void setJvmName(final String jvmName) {
this.jvmName = jvmName;
}
/**
*
* @return the jvm vendor name.
*/
public String getJvmVendor() {
return jvmVendor;
}
/**
*
* @param jvmVendor the jvm vendor name.
*/
public void setJvmVendor(final String jvmVendor) {
this.jvmVendor = jvmVendor;
}
/**
*
* @return the jvm verison.
*/
public String getJvmVersion() {
return jvmVersion;
}
/**
*
* @param jvmVersion the jvm version.
*/
public void setJvmVersion(final String jvmVersion) {
this.jvmVersion = jvmVersion;
}
/**
*
* @return the number of processors.
*/
public String getNumProc() {
return numProc;
}
/**
*
* @param numProc the number of processors.
*/
public void setNumProc(final String numProc) {
this.numProc = numProc;
}
/**
*
* @return the os architecture.
*/
public String getOsArch() {
return osArch;
}
/**
*
* @param osArch set the os architecture.
*/
public void setOsArch(final String osArch) {
this.osArch = osArch;
}
/**
*
* @return the os name.
*/
public String getOsName() {
return osName;
}
/**
*
* @param osName set the os name.
*/
public void setOsName(final String osName) {
this.osName = osName;
}
/**
*
* @return the os version
*/
public String getOsVersion() {
return osVersion;
}
/**
*
* @param osVersion set the os version.
*/
public void setOsVersion(final String osVersion) {
this.osVersion = osVersion;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy