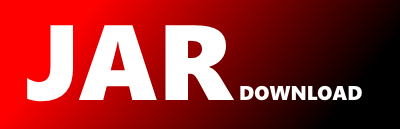
org.ow2.util.maven.jbuilding.ArtifactItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbuilding-maven-plugin Show documentation
Show all versions of jbuilding-maven-plugin Show documentation
Maven plugin that helps to build JOnAS stuff (plans, versions.properties, ...)
The newest version!
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.maven.jbuilding;
import org.apache.maven.artifact.Artifact;
/**
* Represents a maven2 artifact.
* @author Guillaume Sauthier
*
*/
public class ArtifactItem {
/**
* Artifact's groupId.
*/
private String groupId = null;
/**
* Artifact's id.
*/
private String artifactId = null;
/**
* Artifact's classifier. (may be null for default)
*/
private String classifier = null;
/**
* Artifact's type. (default jar)
*/
private String type = "jar";
/**
* Artifact's version. (latest by default)
*/
private String version = Artifact.LATEST_VERSION;
/**
* Needed to determine if the resource must be monitored. Default is false.
*/
private boolean reloadable = false;
/**
* Artifact's side (client or server) default is "both" sides.
*/
private String side = "both";
/**
* Needed to determine if the bundle must be started. Default is true.
*/
private boolean start = true;
/**
* Needed to determine if the bundle must be a referenced JAR file. Default is true.
*/
private boolean reference = true;
/**
* The bundle start level.
*/
private int startLevel = 1;
/**
* Needed to determine if the bundle start operation is transient. Default is true.
*/
private boolean startTransient = true;
/**
* Optional condition for this artifact item (could also be set on Deployment plan)
*/
private String condition = null;
/**
* @return the groupId
*/
public String getGroupId() {
return groupId;
}
/**
* @param groupId the groupId to set
*/
public void setGroupId(final String groupId) {
this.groupId = groupId.trim();
}
/**
* @return the side (server or client) where this bundle should go.
*/
public String getSide() {
return side;
}
/**
* @param side the side (server or client) where this bundle should go.
*/
public void setSide(final String side) {
this.side = side.trim();
}
/**
* @return the artifactId
*/
public String getArtifactId() {
return artifactId;
}
/**
* @param artifactId the artifactId to set
*/
public void setArtifactId(final String artifactId) {
this.artifactId = artifactId.trim();
}
/**
* @return the classifier
*/
public String getClassifier() {
return classifier;
}
/**
* @param classifier the classifier to set
*/
public void setClassifier(final String classifier) {
this.classifier = classifier.trim();
}
/**
* @return the version
*/
public String getVersion() {
return version;
}
/**
* @param version the version to set
*/
public void setVersion(final String version) {
this.version = version.trim();
}
/**
* @return the resource reloadable mode
*/
public boolean isReloadable() {
return reloadable;
}
/**
* @param reloadable the resource reloadable mode
*/
public void setReloadable(final boolean reloadable) {
this.reloadable = reloadable;
}
/**
* @return the bundle starting mode
*/
public boolean isStart() {
return start;
}
/**
* @param start the bundle starting mode to set
*/
public void setStart(final boolean start) {
this.start = start;
}
/**
* @return the bundle reference mode
*/
public boolean isReference() {
return reference;
}
/**
* @param reference the bundle reference mode to set
*/
public void setReference(final boolean reference) {
this.reference = reference;
}
/**
* @return the bundle start level
*/
public int getStartlevel() {
return startLevel;
}
/**
* @param startLevel the bundle start level to set
*/
public void setStartlevel(final int startLevel) {
this.startLevel = startLevel;
}
/**
* @return the artifact type
*/
public String getType() {
return type;
}
/**
* @param type the artifact type
*/
public void setType(final String type) {
this.type = type;
}
/**
* @return the bundle start operation mode
*/
public boolean isStartTransient() {
return startTransient;
}
/**
* @param startTransient the bundle start operation mode
*/
public void setStartTransient(final boolean startTransient) {
this.startTransient = startTransient;
}
/**
* @return ArtifactItem validity condition if any {@code null} otherwise
*/
public String getCondition() {
return condition;
}
/**
* @param condition validity condition for this ArtifactItem
*/
public void setCondition(String condition) {
this.condition = condition;
}
}