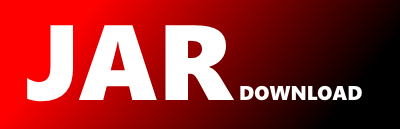
org.ow2.util.maven.jbuilding.GenerateMaven2DeploymentPlansMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbuilding-maven-plugin Show documentation
Show all versions of jbuilding-maven-plugin Show documentation
Maven plugin that helps to build JOnAS stuff (plans, versions.properties, ...)
The newest version!
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.maven.jbuilding;
import java.io.File;
import java.util.Collection;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.model.Resource;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.ow2.util.plan.bindings.deploymentplan.ObjectFactory;
import org.ow2.util.plan.bindings.deploymentplan.maven2.Maven2Deployment;
import org.ow2.util.plan.bindings.deploymentplan.url.UrlDeployment;
/**
* Generate maven2 deployment plans describe in the plugin configuration.
* @author Francois Fornaciari
* @since 2.0.0
*/
@Mojo(name = "generate-maven2-deployment-plans")
public class GenerateMaven2DeploymentPlansMojo extends AbstractResolverMojo {
/**
* Location new resources directory.
* @since 2.0.0
*/
@Parameter(defaultValue = "${project.build.directory}/configuration-resources")
private File output;
/**
* Location of the generated maven2 deployment plans
* @since 2.0.0
*/
@Parameter(defaultValue = "repositories/url-internal")
private String directory;
/**
* Allow timestamp in SNAPSHOT version if set to true
* @since 2.0.0
*/
@Parameter(defaultValue = "false")
private boolean allowTimestamp = false;
/**
* JAXBContext for DP marshalling
*/
private JAXBContext jaxbContext;
public GenerateMaven2DeploymentPlansMojo() {
Class deploymentPlanClass =
org.ow2.util.plan.bindings.deploymentplan.DeploymentPlan.class;
try {
jaxbContext = JAXBContext.newInstance(deploymentPlanClass.getPackage().getName(), deploymentPlanClass.getClassLoader());
} catch (JAXBException e) {
throw new RuntimeException("Cannot create deployment plan marshaller.", e);
}
}
/**
* @throws MojoExecutionException When I cannot copy the artifacts in the
* output directory.
* @see org.ow2.util.maven.jbuilding.AbstractResolverMojo#execute()
*/
public void execute() throws MojoExecutionException {
Map> plans = resolveArtifacts(SERVER_SIDE, BOTH_MODE, DEPLOYMENT_PLANS_ARTIFACT_ITEMS,
this.allowTimestamp);
// Generate maven2 deployment plans describe in the plugin configuration.
Set>> deploymentPlanEntries = plans.entrySet();
for (Entry> deploymentPlanEntry : deploymentPlanEntries) {
String deploymentPlanName = deploymentPlanEntry.getKey();
File file = new File(getDeploymentPlanDirectory(deploymentPlanName), deploymentPlanName.concat(".xml"));
String[] includes = deploymentPlanIncludes.get(deploymentPlanName);
generateDeploymentPlan(file, deploymentPlanEntry.getValue(), includes, deploymentPlanName);
}
}
/**
* @param file
* @param artifacts
* @param includes
* @param deploymentPlanName the deployment plan name
* @throws MojoExecutionException
*/
private void generateDeploymentPlan(final File file, final Collection artifacts,
final String[] includes, final String deploymentPlanName)
throws MojoExecutionException {
try {
ObjectFactory objectFactory = new ObjectFactory();
Marshaller marshaller = jaxbContext.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_ENCODING, "UTF-8");
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE);
marshaller.setProperty(Marshaller.JAXB_SCHEMA_LOCATION,
"http://jonas.ow2.org/ns/deployment-plan/1.0 http://jonas.ow2.org/ns/deployment-plan/1.0/deployment-plan-1.0.xsd " +
"http://jonas.ow2.org/ns/deployment-plan/maven2/1.0 http://jonas.ow2" +
".org/ns/deployment-plan/maven2-deployment-plan-1.0.xsd");
org.ow2.util.plan.bindings.deploymentplan.DeploymentPlan jaxbDeploymentPlan =
objectFactory.createDeploymentPlan();
jaxbDeploymentPlan.setAtomic(false);
jaxbDeploymentPlan.setId(deploymentPlanName);
DeploymentPlan deploymentPlan = deploymentPlanMap.get(deploymentPlanName);
if (deploymentPlan != null) {
String condition = deploymentPlan.getCondition();
if (condition != null) {
jaxbDeploymentPlan.setCondition(condition);
}
}
if (includes != null) {
for (String include : includes) {
UrlDeployment urlDeployment = new UrlDeployment();
// Do not generate deployed element
urlDeployment.setDeployed(null);
urlDeployment.setId(include);
urlDeployment.setResource(include + ".xml");
jaxbDeploymentPlan.getDeployments().add(urlDeployment);
}
}
for (Artifact artifact : artifacts) {
// Get the reloadable mode
ArtifactItem artifactItem = artifactItemMap.get(artifact.getDependencyConflictId());
boolean reloadable = artifactItem.isReloadable();
// Get the bundle start mode
boolean start = artifactItem.isStart();
// Get the bundle reference mode
boolean reference = artifactItem.isReference();
// Get the bundle start level
int startLevel = artifactItem.getStartlevel();
// Get the bundle start operation mode
boolean startTransient = artifactItem.isStartTransient();
String condition = artifactItem.getCondition();
Maven2Deployment maven2Deployment = new Maven2Deployment();
// Do not generate deployed element
maven2Deployment.setDeployed(null);
maven2Deployment.setIdAttr(artifact.getDependencyConflictId());
maven2Deployment.setCondition(condition);
maven2Deployment.setStartAttr(start);
maven2Deployment.setReloadableAttr(reloadable);
maven2Deployment.setReferenceAttr(reference);
maven2Deployment.setStartlevelAttr(startLevel);
maven2Deployment.setStarttransientAttr(startTransient);
maven2Deployment.setGroupId(artifact.getGroupId());
maven2Deployment.setArtifactId(artifact.getArtifactId());
if (artifact.getClassifier() != null) {
maven2Deployment.setClassifier(artifact.getClassifier());
}
String version;
if (this.allowTimestamp) {
version = artifact.getVersion();
} else {
version = artifact.getBaseVersion();
}
maven2Deployment.setVersion(version);
maven2Deployment.setType(artifact.getType());
jaxbDeploymentPlan.getDeployments().add(maven2Deployment);
}
marshaller.marshal(objectFactory.createDeploymentPlan(jaxbDeploymentPlan), file);
} catch (JAXBException e) {
throw new MojoExecutionException("Cannot create deployment plan: " + deploymentPlanName, e);
}
// Add the generated file in the resources of maven
Resource resource = new Resource();
resource.setDirectory(output.getPath());
project.addResource(resource);
}
/**
* @return the output file.
*/
public File getOutput() {
return output;
}
/**
* @return the directory.
*/
public String getDirectory() {
return directory;
}
/**
* Set the given output.
* @param output the output file
*/
public void setOutput(final File output) {
this.output = output;
}
/**
* Sets the given directory.
* @param directory the directory path
*/
public void setDirectory(final String directory) {
this.directory = directory;
}
private File getDeploymentPlanDirectory(final String deploymentPlanName) throws MojoExecutionException {
String planDirectoryLocation = directory;
DeploymentPlan deploymentPlan = deploymentPlanMap.get(deploymentPlanName);
if (deploymentPlan != null && deploymentPlan.getDirectory() != null) {
planDirectoryLocation = deploymentPlan.getDirectory();
}
File planDirectoryLocationFile = new File(output, planDirectoryLocation.replace('/', File.separatorChar));
// Create the directory
if (!planDirectoryLocationFile.exists()) {
if (!planDirectoryLocationFile.mkdirs()) {
throw new MojoExecutionException("Cannot create directory: " + planDirectoryLocationFile);
}
}
return planDirectoryLocationFile;
}
}