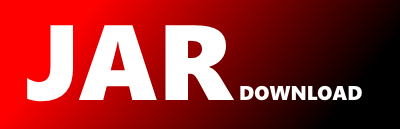
org.ow2.util.maven.jbuilding.GenerateSUGroupMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbuilding-maven-plugin Show documentation
Show all versions of jbuilding-maven-plugin Show documentation
Maven plugin that helps to build JOnAS stuff (plans, versions.properties, ...)
The newest version!
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.maven.jbuilding;
import java.io.File;
import java.io.IOException;
import java.util.Collection;
import java.util.Date;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.codehaus.plexus.util.FileUtils;
/**
* Generate SU group.
*
* @author Loris Bouzonnet
* @since 2.0.0
*/
@Mojo(name = "generate-su-group")
public class GenerateSUGroupMojo extends AbstractResolverMojo {
/**
* Location new resources directory.
*
* @since 2.0.0
*/
@Parameter(defaultValue = "${project.build.directory}/generated-su-resources")
private File output;
/**
* Group name.
*
* @since 2.0.0
*/
@Parameter(defaultValue = "jonas-autodeploy-bundles")
private String group;
/**
* Allow timestamp in SNAPSHOT version if set to true
*
* @since 2.0.0
*/
@Parameter(defaultValue = "false")
private boolean allowTimestamp = false;
public void execute() throws MojoExecutionException, MojoFailureException {
final Collection artifactsToInstall =
resolveArtifacts(SERVER_SIDE, BOTH_MODE, AUTO_DEPLOY_ARTIFACT_ITEMS, this.allowTimestamp).get(
AUTO_DEPLOY_ARTIFACT_ITEMS);
// OK, now we're sure that everything is in the local repository
// We will generate a xml file
final File propertyFile =
new File(this.output, this.group + "-su.xml");
final File parent = propertyFile.getParentFile();
// Create the directory
if (!parent.exists()) {
if (!parent.mkdirs()) {
throw new MojoExecutionException("Cannot create directory: "
+ parent);
}
}
// Generate the xml
final StringBuilder sb = new StringBuilder();
sb.append("\n");
sb.append("\n");
sb.append("\n");
if (artifactsToInstall != null) {
for (final Artifact artifact : artifactsToInstall) {
sb.append(" \n");
sb.append(" \n");
sb.append(" " + artifact.getGroupId() + " \n");
sb.append(" " + artifact.getArtifactId() + " \n");
if (artifact.getClassifier() != null) {
sb.append(" " + artifact.getClassifier() + " \n");
}
sb.append(" " + artifact.getVersion() + " \n");
sb.append(" " + artifact.getType() + " \n");
sb.append(" \n");
sb.append(" \n");
}
}
sb.append(" ");
// add a final EOL
sb.append("\n");
// Flush the content
try {
FileUtils.fileWrite(propertyFile.getPath(), sb.toString());
} catch (final IOException e) {
throw new MojoExecutionException("Cannot create file "
+ propertyFile);
}
}
}