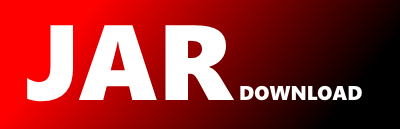
org.ow2.util.maven.jbuilding.VersionsMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbuilding-maven-plugin Show documentation
Show all versions of jbuilding-maven-plugin Show documentation
Maven plugin that helps to build JOnAS stuff (plans, versions.properties, ...)
The newest version!
/**
* Copyright 2007-2012 Bull S.A.S.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.maven.jbuilding;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.Stack;
import java.util.TreeMap;
import java.util.regex.Pattern;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.repository.DefaultRepositoryRequest;
import org.apache.maven.artifact.repository.RepositoryRequest;
import org.apache.maven.artifact.resolver.ArtifactResolutionRequest;
import org.apache.maven.artifact.resolver.ArtifactResolutionResult;
import org.apache.maven.artifact.resolver.ResolutionNode;
import org.apache.maven.artifact.resolver.filter.ScopeArtifactFilter;
import org.apache.maven.model.Dependency;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.codehaus.plexus.util.FileUtils;
/**
* Process a project and explore artifacts versions.
* @author Guillaume Sauthier
* @since 2.0.0
*/
@Mojo(name = "versions",
defaultPhase = LifecyclePhase.PROCESS_SOURCES)
public class VersionsMojo extends AbstractResolverMojo {
/**
* Property pattern.
*/
private static Pattern PROPERTY_NAME_PATTERN = Pattern.compile(".*\\.version$");
/**
* Location of the versions.properties to be created.
* @since 2.0.0
*/
@Parameter(defaultValue = "${project.build.directory}/versions.properties")
private File output;
/**
* Group Ids to be joined if they share the same version.
* @since 2.0.0
*/
@Parameter
private List joinedGroupIds = new ArrayList();
/**
* @since 2.0.0
*/
@Parameter(alias = "jbuilding.versions.properties",
defaultValue = "false")
private boolean browseProperties = false;
/**
* @since 2.0.0
*/
@Parameter
private String[] preferedGroupIds;
/**
* Dependencies depth filtering.
* @since 2.0.0
*/
@Parameter(defaultValue = "3")
private int depth = 3;
/**
* ArtifactCollector filtering on artifact's scope.
* @since 2.0.0
*/
@Parameter(defaultValue = Artifact.SCOPE_COMPILE)
private String collectScope = Artifact.SCOPE_COMPILE;
/**
* Allow timestamp in SNAPSHOT version if set to true
* @since 2.0.0
*/
@Parameter(defaultValue = "false")
private boolean allowTimestamp = false;
/**
* Joined groupIds with their default version.
*/
private Map joinGroups = new HashMap();
/**
* List of resolvables artifacts (to be used with the collector to resolve first depth dependencies).
*/
private Set collectableArtifacts = new HashSet();
/**
* Default Bean constructor.
*/
public VersionsMojo() {
// Default value : no preferences
preferedGroupIds = new String[1];
preferedGroupIds[0] = "*";
}
/**
* Writes the versions.properties file.
* @throws MojoExecutionException
* @throws MojoFailureException
*/
public void execute() throws MojoExecutionException, MojoFailureException {
getLog().debug("Introspecting project " + project.getGroupId() + ":" + project.getArtifactId());
// Resolve the artifacts provided in the plugin configuration
Map> map = resolveArtifacts(SERVER_SIDE, BOTH_MODE, ALL_ARTIFACT_ITEMS,
this.allowTimestamp);
// Init the Version map
Map versionsProperties = new TreeMap();
// Browse properties
if (browseProperties) {
getVersionsFromModelProperties(versionsProperties);
getVersionsFromProjectProperties(versionsProperties);
}
// Browse dependencies
getVersionsFromArtifactItems(map, versionsProperties);
getVersionsFromProjectDependencies(versionsProperties);
// Browse transitive dependencies
getVersionsFromTransitiveDependencies(versionsProperties);
// Don't forget joined group Ids
getVersionsFromJoinedGroupIds(versionsProperties);
// Store the file
try {
getLog().debug("Storing in " + output);
storeVersionsProperties(versionsProperties);
} catch (IOException ioe) {
throw new MojoExecutionException("Cannot store " + output, ioe);
}
}
/**
* Extract version information from project's runtime properties
* @param versions versions
*/
public void getVersionsFromProjectProperties(final Map versions) {
getLog().debug("Looking in project's properties");
// Look on the project runtime properties
filterProperties(project.getProperties(), versions);
}
/**
* Extract version information from project's model properties
* @param versions versions
*/
public void getVersionsFromModelProperties(final Map versions) {
getLog().debug("Looking in project's model properties");
// Look on the ${project.properties.*} values
filterProperties(project.getModel().getProperties(), versions);
}
/**
* Extract versions informations from ArtifactItems listed in the jbuilding plugin configuration.
* @param groups groups of ArtifactItems
* @param versions versions
*/
public void getVersionsFromArtifactItems(final Map> groups, final Map versions) {
// Iterates over groups (~ deployment-plans)
for (Map.Entry> group : groups.entrySet()) {
// Iterates over artifact items in the group
for (Artifact artifact : group.getValue()) {
String groupId = artifact.getGroupId();
String artifactVersion = null;
//Bug fix UTIL-84
if (!this.allowTimestamp && artifact.getBaseVersion() != null) {
artifactVersion = artifact.getBaseVersion();
} else {
artifactVersion = artifact.getVersion();
}
if (!filterGroupId(groupId, artifactVersion)) {
// if the artifact did need to be filtered, just write the property
String name = getArtifactName(artifact.getGroupId(),
artifact.getArtifactId());
addVersion(versions, name, artifactVersion);
}
// Add this artifact
collectableArtifacts.add(artifact);
}
}
}
/**
* Extract versions informations from project's dependencies.
* @param versions versions
*/
@SuppressWarnings({"unchecked"})
public void getVersionsFromProjectDependencies(final Map versions) {
// Iterates on all project's dependencies
List dependencies = project.getDependencies();
if (dependencies != null) {
for (Dependency dependency : dependencies) {
String groupId = dependency.getGroupId();
String artifactId = dependency.getArtifactId();
String dependencyVersion = dependency.getVersion();
String dependencyType = dependency.getType();
String dependencyClassifier = dependency.getClassifier();
if (!filterGroupId(groupId, dependencyVersion)) {
// if the artifact didn't need to be filtered, just write the property
String name = getArtifactName(groupId,
artifactId);
addVersion(versions, name, dependencyVersion);
}
// Add this artifact
collectableArtifacts.add(createArtifact(groupId, artifactId, dependencyVersion, dependencyType, dependencyClassifier));
}
}
}
/**
* Extract versions information from transitive dependencies of the
* collected artifacts (dependencies + bundle items)
* @param versions versions
* @throws MojoExecutionException if POM collection failed
*/
private void getVersionsFromTransitiveDependencies(final Map versions) throws MojoExecutionException {
// Collect metadata information from all the collected artifacts
for (Artifact collectable : collectableArtifacts) {
ArtifactResolutionResult result = resolveArtifactTransitively(collectable);
Set nodes = result.getArtifactResolutionNodes();
for (ResolutionNode node : nodes) {
// Only manage firsts depths nodes
if (node.getDepth() < depth) {
Artifact artifact = node.getArtifact();
String groupId = artifact.getGroupId();
String artifactId = artifact.getArtifactId();
String dependencyVersion = artifact.getVersion();
// For the SNAPSHOT artifacts, its base version should be use than its version.
if (!this.allowTimestamp && artifact.getBaseVersion() != null) {
dependencyVersion = artifact.getBaseVersion();
} else {
dependencyVersion = artifact.getVersion();
}
if (!filterGroupId(groupId, dependencyVersion)) {
// if the artifact didn't need to be filtered, just write the property
String name = getArtifactName(groupId,
artifactId);
addVersion(versions, name, dependencyVersion);
}
}
}
}
}
/**
* Extract versions informations from joined groupIds.
* @param versions versions
*/
public void getVersionsFromJoinedGroupIds(final Map versions) {
for (Map.Entry entry : joinGroups.entrySet()) {
String name = entry.getKey();
String version = entry.getValue();
addVersion(versions, name, version);
}
}
/**
* Filter properties values and only select the one finishing with ".version"
* @param filteredProperties properties to be filtered
* @param versions versions
*/
private void filterProperties(final Properties filteredProperties,
final Map versions) {
if (filteredProperties != null) {
// Filter all existing properties
for (Map.Entry