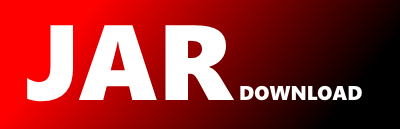
org.ow2.util.geolocation.client.GeoClient Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2009 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: GeoClient.java 5360 2010-02-24 14:29:45Z benoitf $
* --------------------------------------------------------------------------
*/
package org.ow2.util.geolocation.client;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Properties;
import org.apache.commons.httpclient.HostConfiguration;
import org.apache.commons.httpclient.HttpClient;
import org.apache.commons.httpclient.HttpException;
import org.apache.commons.httpclient.HttpMethod;
import org.apache.commons.httpclient.UsernamePasswordCredentials;
import org.apache.commons.httpclient.auth.AuthScope;
import org.apache.commons.httpclient.methods.PutMethod;
import org.apache.commons.httpclient.params.HttpConnectionManagerParams;
import org.apache.log4j.ConsoleAppender;
import org.apache.log4j.FileAppender;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
import org.apache.log4j.PatternLayout;
import org.apache.log4j.varia.LevelMatchFilter;
import org.apache.log4j.varia.NullAppender;
/**
* This class send user informations to the stat service.
*
* @author mancelin
*
*/
public class GeoClient extends Thread {
/**
* Timeout of the HTTP connection.
*/
private static final int TIMEOUT = 5000;
/**
* The logger of the class for traces.
*/
private static final Logger LOGGER_EZB = Logger.getLogger(GeoClient.class);
/**
* The logger of the class of httpclient for traces.
*/
private static final Logger LOGGER_HTTP = Logger.getLogger("org.apache.commons.httpclient");
/**
* The logger of the class of httpclient for traces.
*/
private static final Logger LOGGER_HTTP2 = Logger.getLogger("httpclient");
/**
* Properties file for the address of the webservice.
*/
private Properties clientProperties = null;
/**
* The proxy address.
*/
private String proxyaddress = null;
/**
* The proxy port.
*/
private String proxyport = null;
/**
* The proxy username.
*/
private String proxyuser = null;
/**
* The proxy password.
*/
private String proxypwd = null;
/**
* The url of the geo service.
*/
private String url = null;
/**
* The constructor of the geoclient.
* @param productName the name of the product to register.
* @param productVersion the version of the product to register.
* @param email the email address of the user
* @param proxyaddress the address of the proxy. "" if nothing.
* @param proxyport the port of the proxy. "" if nothing.
* @param proxyuser the username for the proxy. "" if nothing.
* @param proxypwd the password for the proxy. "" if nothing.
*/
public GeoClient(
final String productName,
final String productVersion,
final String email,
final String proxyaddress,
final String proxyport,
final String proxyuser,
final String proxypwd) {
this.proxyaddress = proxyaddress;
this.proxyport = proxyport;
this.proxyuser = proxyuser;
this.proxypwd = proxypwd;
try {
this.clientProperties = openPropertiesFile("url.properties");
} catch (Exception e) {
LOGGER_EZB.log(Level.INFO,
"Error while reading properties file at url.properties");
LOGGER_EZB.log(Level.ERROR, e);
System.exit(-1);
}
this.url = clientProperties.getProperty("url")
+ "product="
+ productName
+ "&version="
+ productVersion
+ "&email="
+ email;
this.url +=
"&numProc="
+ String.valueOf(Runtime.getRuntime().availableProcessors())
+ "&jreVersion="
+ System.getProperty("java.version")
+ "&jreVendor="
+ System.getProperty("java.vendor")
+ "&jvmVersion="
+ System.getProperty("java.vm.version")
+ "&jvmVendor="
+ System.getProperty("java.vm.vendor")
+ "&jvmName="
+ System.getProperty("java.vm.name")
+ "&osName="
+ System.getProperty("os.name")
+ "&osArch="
+ System.getProperty("os.arch")
+ "&osVersion="
+ System.getProperty("os.version");
this.url = this.url.replace(" ", "%20");
//sendInfo(url);
}
/**
* Run method of the thread.
*/
@Override
public void run() {
sendInfo(url);
}
/**
* This method is used to open a propertie file and load it into a
* properties object resource.
*
* @param path
* the path of the properties file to load.
* @return the Properties Object.
*/
public synchronized Properties openPropertiesFile(final String path) {
Properties tmp = new Properties();
try {
tmp.load(GeoClient.class.getClassLoader().getResourceAsStream(path));
} catch (FileNotFoundException e) {
LOGGER_EZB.log(Level.ERROR, e);
} catch (IOException e) {
LOGGER_EZB.log(Level.ERROR, e);
}
return tmp;
}
/**
* This method send the informations on the stat service with a PUT method.
*
* @param host
* the address of the stat service.
*/
public synchronized void sendInfo(final String host) {
HttpMethod method = null;
HttpClient client = new HttpClient();
HttpConnectionManagerParams params = new HttpConnectionManagerParams();
params.setConnectionTimeout(TIMEOUT);
params.setSoTimeout(TIMEOUT);
client.getHttpConnectionManager().setParams(params);
boolean proxyconfiguration = false;
boolean pwdconfiguration = false;
if (proxyaddress != null && proxyport != null) {
proxyconfiguration = (!proxyaddress.equals("")) && (!proxyport.equals(""));
}
if (proxyuser != null && proxypwd != null) {
pwdconfiguration = (!proxyuser.equals("")) && (!proxypwd.equals(""));
}
/**
* Check if proxy configuration is present
*/
if (proxyaddress != null && proxyport != null) {
if (proxyconfiguration) {
HostConfiguration hostConfig = client.getHostConfiguration();
try {
/**
* Proxy configuration within HttpClient
*/
hostConfig.setProxy(proxyaddress, Integer.valueOf(proxyport));
client.getState().setProxyCredentials(
new AuthScope(proxyaddress, Integer.valueOf(proxyport),
AuthScope.ANY_REALM),
new UsernamePasswordCredentials(proxyuser, proxypwd));
} catch (Exception e) {
LOGGER_EZB.log(Level.ERROR, "Bad proxy port parameter");
}
}
} else {
LOGGER_EZB.log(Level.ERROR, "Unknown proxy scope parameters");
}
method = new PutMethod(host);
/**
* Authentification on the proxy server
*/
if (proxyuser != null && proxypwd != null) {
if (pwdconfiguration) {
method.setDoAuthentication(true);
}
} else {
LOGGER_EZB.log(Level.ERROR, "Unknown proxy credentials parameters");
}
try {
/**
* Execute the GET method on the Easybeans file
*/
client.executeMethod(method);
} catch (HttpException e) {
LOGGER_EZB.log(Level.ERROR, e);
} catch (IOException e) {
LOGGER_EZB.log(Level.ERROR, e);
}
try {
/**
* Get the body of the PUT method
*/
String responce = method.getResponseBodyAsString();
LOGGER_EZB.log(Level.ERROR, responce);
} catch (Exception e) {
LOGGER_EZB.log(Level.ERROR, e);
}
try {
method.releaseConnection();
} catch (Exception e) {
LOGGER_EZB.log(Level.ERROR,
"Error while writing data in destination file : " + e);
}
}
/**
* Main method of the program.
*
* @param args
* the command line arguments.
*/
public static void main(final String[] args) {
/**
* Configuration of log4j loggers. The console appender only displays
* INFO traces
*/
PatternLayout layout = new PatternLayout("%d %p - %F:%L - %m%n");
PatternLayout layoutconsole = new PatternLayout("%m%n");
ConsoleAppender stdout = new ConsoleAppender(layoutconsole);
NullAppender httpclient = new NullAppender();
LevelMatchFilter filterError = new LevelMatchFilter();
LevelMatchFilter filterDebug = new LevelMatchFilter();
LevelMatchFilter filterFatal = new LevelMatchFilter();
filterError.setLevelToMatch("ERROR");
filterDebug.setLevelToMatch("DEBUG");
filterFatal.setLevelToMatch("FATAL");
filterError.setAcceptOnMatch(false);
filterDebug.setAcceptOnMatch(false);
filterFatal.setAcceptOnMatch(false);
stdout.addFilter(filterDebug);
stdout.addFilter(filterError);
stdout.addFilter(filterFatal);
FileAppender fileout, httpfileout;
try {
if (args.length != 0) {
/**
* Create log files in the installation path
*/
fileout = new FileAppender(layout, args[0] + "geoclient.log");
httpfileout = new FileAppender(layout, args[0] + "HttpClient.log");
} else {
fileout = new FileAppender(layout, "geoclient.log");
httpfileout = new FileAppender(layout, "HttpClient.log");
}
LOGGER_EZB.addAppender(fileout);
LOGGER_EZB.addAppender(stdout);
LOGGER_HTTP.addAppender(httpfileout);
LOGGER_HTTP2.addAppender(httpclient);
} catch (IOException e) {
e.printStackTrace();
}
/**
* Checking if argument are present
*/
if (args.length < 2) {
LOGGER_EZB.log(Level.ERROR, "Bad argument at launching time");
System.exit(-1);
} else {
String name = args[0];
String version = args[1];
String email = args[2];
String add = "";
String port = "";
String user = "";
String pwd = "";
if (args.length >= 4) {
add = args[3];
}
if (args.length >= 5) {
port = args[4];
}
if (args.length >= 6) {
user = args[5];
}
if (args.length >= 7) {
pwd = args[6];
}
GeoClient client = new GeoClient(name, version, email, add, port,
user, pwd);
client.start();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy